Linear Search in C Language with Example Program
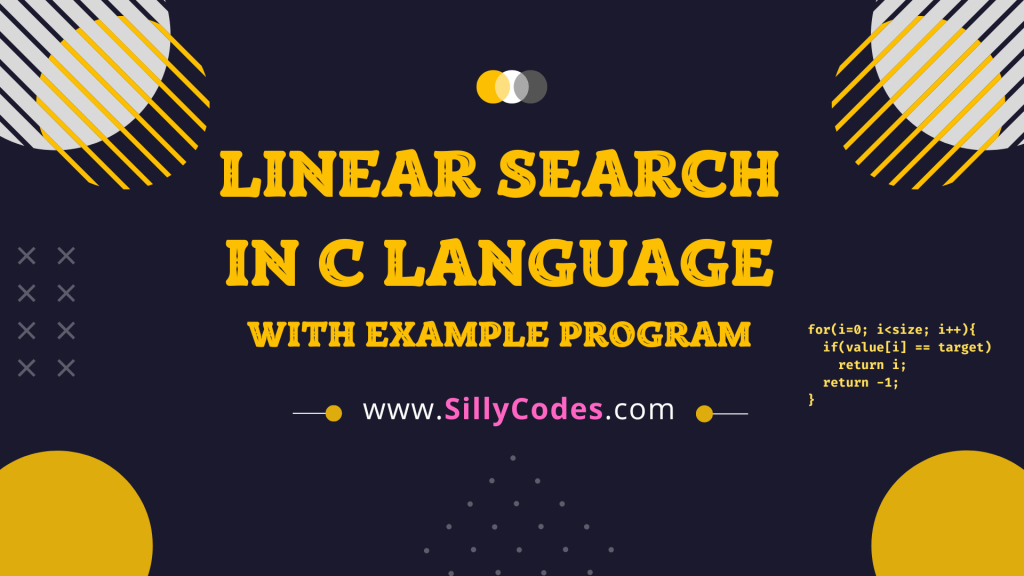
Program Description:
Write a Program to demonstrate the Linear Search in C programming language. The program should accept an array and number to be searched from the user, Then the program should check if the given number is found in the input array using the Linear Search Algorithm.
Example Input and Output:
Input:
Enter desired array size(1-100): 7
Please enter array elements(7) : 24 78 13 56 87 32 76
Enter a Number to search in Array: 13
Output:
FOUND - The 13 number Found at index 2
The target number 13 is found at the index 2 in the Input array 24 78 13 56 87 32 76.
Prerequisites:
It is recommended to know the basics of the Arrays and Functions in C language.
- Arrays in C Language with Example Programs
- Functions in C programming – how to create and use functions
- Passing Arrays to Functions in C
What is Linear Search:
Linear search is one of the most simple searching algorithms. The Linear search is used to search for an element in an array (Any Data structure).
In the Linear search, We check each element in the array one by one until the desired element (target element) is found or till the end of the array. If we reach the end of the array without finding the target element, Then the target element is not present in the given array.
The Time Complexity of the Linear Search is O(n) (Big O(n))
So It takes linear time to search for an element in the array. The Linear search is useful to search small arrays but can be inefficient if the array size is very large (As we need to check all elements).
📢 Time Complexity of Linear Search is O(n)
One of the advantages of Linear Search is it can work even when the input array is Not Sorted ( Unlike Binary Search, Where the array must be Sorted).
Linear Search in C Algorithm:
Here is the Algorithm for the linear Search in programming.
Let’s say the input array as the values and the size is the array size and the targetNum as the number to search.
- Iterate over the input array from the first element to the last element ( For Loop, Start from i=0 to i<size)
- At Each Iteration, Check if the current element (
values[i]) is equal to the target number(
targetNum).
- If the current element is equal to the target element, Then we found the element( targetNum), Return the Index of the current element.
- If the current element is not equal to the target element, Then Continue to the next iteration.
- If the i reaches end of the array, Then targetNum is not found in the array, Return -1. (Not Found)
Therefore, we can determine whether the target number is present in the array by examining the returned Index.
Let’s look at the step-by-step explanation of the Linear Search Program in C language.
Linear Search in C Program Explanation:
- Start the program by declaring an array named values[100]. The values array can hold up to 100 elements. ( Change this number if you want to try with large arrays)
- We defined two functions. readInput() function and the linear_search() function. Let’s look at them in detail.
- The readInput() function is used to take the input values for the array elements. So it takes two formal arguments the values array and its size. The readInput() function updates the values array of elements based on user input.
- Call the readInput function from the main() function – readInput(values, size);
- Take the Number to be searched from the user i.e targetNum.
- Now, To search for the target number ( targetNum) in the values array, Call the linear_search() function with the values array, size and targetNum as the parameters. – linear_search(values, size, targetNum);
- The
linear_search() function uses the linear search to search for the
targetNum in the
values array.
- Uses a For loop to traverse through the array. Starts a For loop from i=0 and goes till the i<size, At Each Iteration,
- Check if the values[i] is equal to targetNum – if(values[i] == targetNum)
- If the above condition is true, Then we found the targetNum in the values array. Return the index i back to the caller.
- If the above condition is false, Then continue to the next element in the array. Increment the i by 1. and continue the above steps.
- Once the for loop is completed and we still haven’t found the targetNum in the array, Then return -1.
- Uses a For loop to traverse through the array. Starts a For loop from i=0 and goes till the i<size, At Each Iteration,
- In the main() function, Grab the return value of the linear_search() function in index variable. – index = linear_search(values, size, targetNum);
- Check if the index == -1, If it is true, Then targetNum is not found in the values array. display the NOT FOUND message on the standard output.
- Otherwise, We found the targetNum in the values array, Display the FOUND message on the console.
- Stop the program.
Program to demonstrate Linear Search in C Language:
Here is the Linear Search Program in C language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
/* Program to Search for an elemenet in array. sillycodes.com */ #include <stdio.h> /** * @brief - Read the input from the user and update 'values' array * * @param values - 'values' array * @param size - size of 'values' array */ void readInput(int values[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("values[%d] : ", i); scanf("%d", &values[i]); } } /** * @brief - Search for the 'targetNum' in the 'values' array * * @param values - array to be searched * @param size - size of 'values' array * @param targetNum - Number to be searched. * @return int - (-1) if not found, otherwise index of element */ int linear_search(int values[], int size, int targetNum) { int i; // find the 'targetNum' in 'values' array for(i = 0; i < size; i++) { if(values[i] == targetNum) { // found the number, Return Index return i; } } // Not found, Return -1 return -1; } int main() { // declare the 'values' array int values[100]; int targetNum, i, size; printf("Enter desired array size(1-100): "); scanf("%d", &size); // Read user input and update the array readInput(values, size); printf("Enter a Number to search in Array: "); scanf("%d", &targetNum); // Call the 'linear_search' function to search for 'targetNum' int index = linear_search(values, size, targetNum); // Check if the 'targetNum' is found if(index == -1) printf("NOT FOUND - The %d number Not Found in Array\n", targetNum); else printf("FOUND - The %d number Found at index %d\n", targetNum, index); return 0; } |
Prototype details of the linear_search function
- Function Name: linear_search
- Argument List: (int values[], int size, int targetNum)
- Return Type:
int
- returns index if the targetNum found in the values array
- Otherwise, -1
Program Output:
Compile and Run the program.
We are using the GCC compiler on the Ubuntu Linux system.
$ gcc linear-search.c
Run the Program. The above command generates the executable file a.out
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 7 Please enter array elements(7) : 24 78 13 56 87 32 76 Enter a Number to search in Array: 13 FOUND - The 13 number Found at index 2 $ |
Let’s try a few more examples
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 10 20 30 40 50 Enter a Number to search in Array: 50 FOUND - The 50 number Found at index 4 $ |
As we can see from the above output, The target number 50 is found in the input array( 10 20 30 40 50) at index 4.
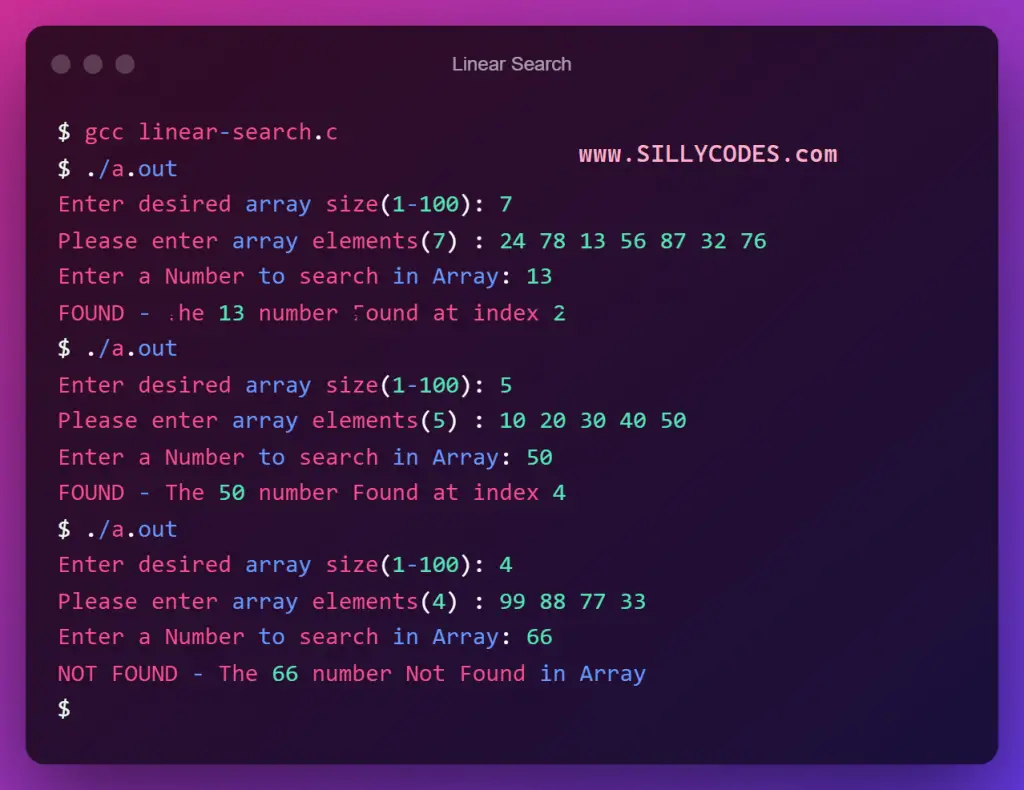
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 4 Please enter array elements(4) : 99 88 77 33 Enter a Number to search in Array: 66 NOT FOUND - The 66 number Not Found in Array $ |
In the above case, The target number 66 is not found in the input array( 99 88 77 33). So the program displayed the NOT FOUND - The 66 number Not Found in Array notice message.
Related Array Practice Programs:
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
2 Responses
[…] have looked at the Linear Search in C program in earlier Posts, In today’s post, We will look at the Program to Count Frequency of Each Element […]
[…] Linear Search in C Language with Example Programs […]