Right Rotation of Array in C Langauge – Right Rotate Array N Times
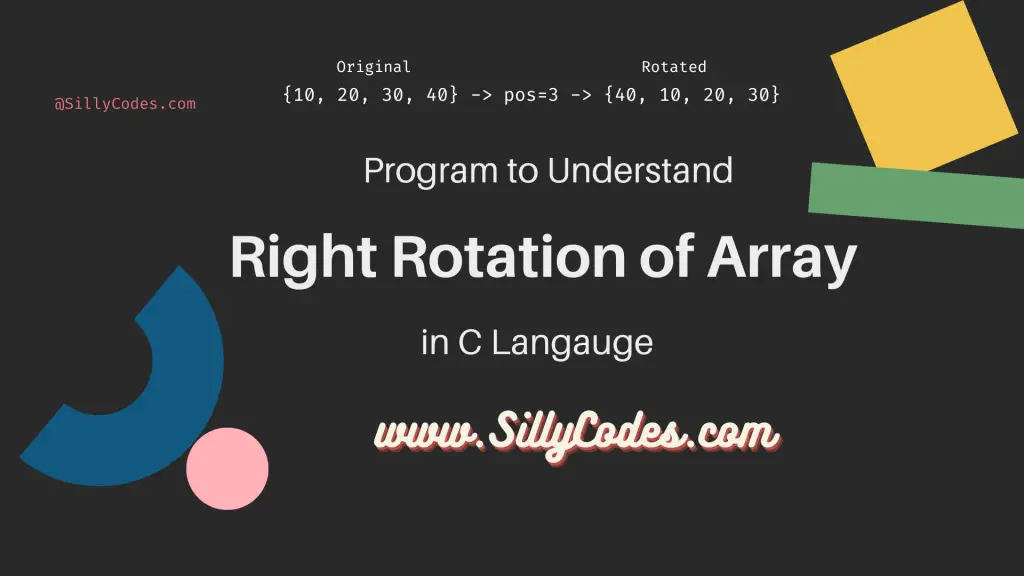
Program Description:
Write a Program to understand the Right Rotation of Array in C Programming Language. The user will input an array and the number of positions to be rotated to the right(Right Rotation). Then the program will rotate the specified number of elements in the array in the right direction.
Here is the example input and output of the program
Example Input and Output:
Input:
Enter desired size for array(1-100): 6
Please enter array elements(6) : 34 54 78 28 12 99
Enter the number of position to rotate the array: 2
Output:
Original Array: 34 54 78 28 12 99
Array After Right Rotating 2 Positions : 78 28 12 99 34 54
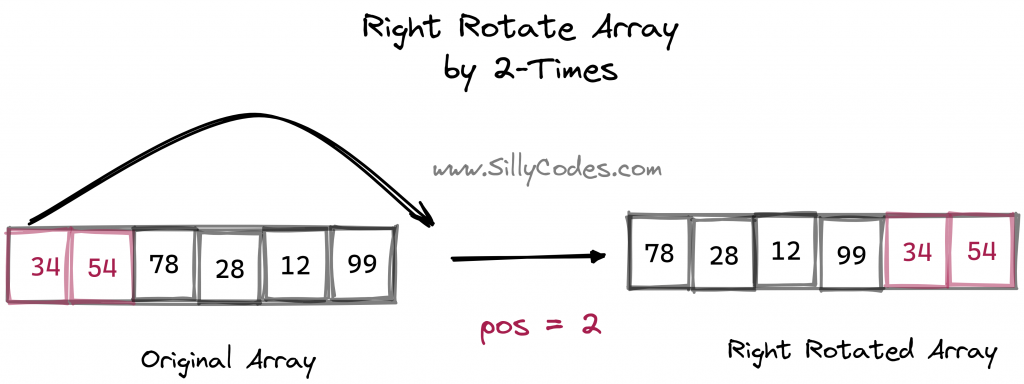
As we can see from the above output When the user provided the input array as 34 54 78 28 12 99 and the number of positions as 2, Then the program right rotated the array by 2 elements and displayed the resultant array 78 28 12 99 34 54 on console.
📢This Program is part of the Array Practice Programs Series
Prerequisites:
It is recommended to go through the following articles to learn more about the C Array and C Functions.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
Algorithm for Right Rotation of Array in C:
Let’s look at the Algorithm for Right Rotating an Array in the C language
Let’s say the input array is numbers array and the total number of the array elements are equal to size. and The variable positioncontains the number of elements that need to be rotated in the Right side direction.
- Array is numbers
- The size of the array is size
- The number of positions to be rotated is position
To Rotate the array by positionelements,
- Create a Temporary Array( tempArr) with the same size as the input array numbers
- Now, We need to iterate over the input array ( numbers) and insert the elements in the temporary array( tempArr) at the correct index after rotation of the position number of elements.
- The correct position after rotating the array by
position position can be calculated using the formula –
(i + size - position) % size.
- Where, i is the present element index ( for loop index)
- position is the number of positions to be shifted in the Right direction
- size is the total size of the input array.
- Once the above loop is completed, The temporary array ( tempArr) contains the Array which is rotated by position number of elements.
- Replace the Input array ( numbers) elements with the Temporary array ( tempArr) elements.
- Now, The numbers array contains the Right Rotated array.
Now, Let’s look at the step-by-step explanation of the Array Right Rotation Program in C language
Right Rotation of Array in C Program Explanation:
- Start the program by declaring two arrays. Input Array which is numbers and Temporary Array which is tempArr. The max size of both arrays is equal to 100. ( Change the 100 max size, if you want to use large arrays – Use can also use a macro or const int to hold the 100 value, For brevity we are directly using the number).
- Take the desired array size from the user and store it in the size variable.
- Check if the size is going beyond the array bounds using – (size >= 100 || size <= 0) condition. Display an error message if the array is going out of bounds.
- We define two helper functions named
read() function and
display() function to read and print array elements
- The read() function is used to read the input elements from the user and update the numbers array
- The display() function is used to print the array elements on the Standard Output
- Now Call the read() function with numbers array and size as the parameter to Take user input and update the numbers array. – read(numbers, size);
- Make another function call to display() function to print the array elements on the console. – display(numbers, size);
- To Right Rotate the array of elements by position number of elements,
- Once the above loop is completed, The tempArr contains the Right Rotated Array.
- Replace the original array elements with the
tempArr elements.
- Use another For loop start from i=0 to i<size
- Copy tempArr[i] to the numbers[i] – numbers[i] = tempArr[i];
- Display the Right rotated array on the console by calling the display() function from the main() function. – display(numbers, size);
- Stop the program.
Program to Understand the Right Rotation of Array in C Language:
Convert the above algorithm into the C Program. Here is the Program to Right Rotate the array elements by ‘position’ positions in C language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
/*     Program Right Rotate Array 'n' positions or 'position' positions     Author: SillyCodes.com */  #include <stdio.h>  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }   int main() {      // declare the 'numbers' array     int numbers[100], tempArr[100] = {0};     int i, position=0, size;      // Take the Array1('numbers') details from the user     printf("Enter desired size for array(1-100): ");     scanf("%d", &size);     // check for array bounds     if(size >= 100 || size <= 0)     {         printf("Invalid Size, Try again \n");         return 0;     }     // User input for array elements     read(numbers, size);      printf("Enter the number of position to rotate the array: ");     scanf("%d", &position);      // Print the 'numbers' array     printf("Original Array: ");     display(numbers, size);      // Store the rotated values of the original array in a temparory array     // store at ((i+size-position) % size) index in the new array     for(i = 0; i<size; i++)     {         // 'numbers[i]' goes to '(i+size-position) % size' index of 'tempArr'         tempArr[((i+size-position) % size)] = numbers[i];     }         // Replace the original array with elements with 'tempArr' elements     for ( i = 0; i < size; i++)     {         numbers[i] = tempArr[i];     }         // Print the merged Array     printf("Array After Right Rotating %d Positions : ", position);     display(numbers, size);      return 0; } |
Program Output:
Compile and Run the program using GCC compiler (Any Compiler)
$ gcc right-rotate-arr.c
Run the executable file.
Test 1: Test the normal cases:
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 10 Please enter array elements(10) : 1 2 3 4 5 6 7 8 9 10 Enter the number of position to rotate the array: 4 Original Array: 1 2 3 4 5 6 7 8 9 10 Array After Right Rotating 4 Positions : 5 6 7 8 9 10 1 2 3 4 $ |
Let’s try a few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter desired size for array(1-100): 6 Please enter array elements(6) : 34 54 78 28 12 99 Enter the number of position to rotate the array: 2 Original Array: 34 54 78 28 12 99 Array After Right Rotating 2 Positions : 78 28 12 99 34 54 $ ./a.out Enter desired size for array(1-100): 4 Please enter array elements(4) : 10 20 30 40 Enter the number of position to rotate the array: 3 Original Array: 10 20 30 40 Array After Right Rotating 3 Positions : 40 10 20 30 $ |
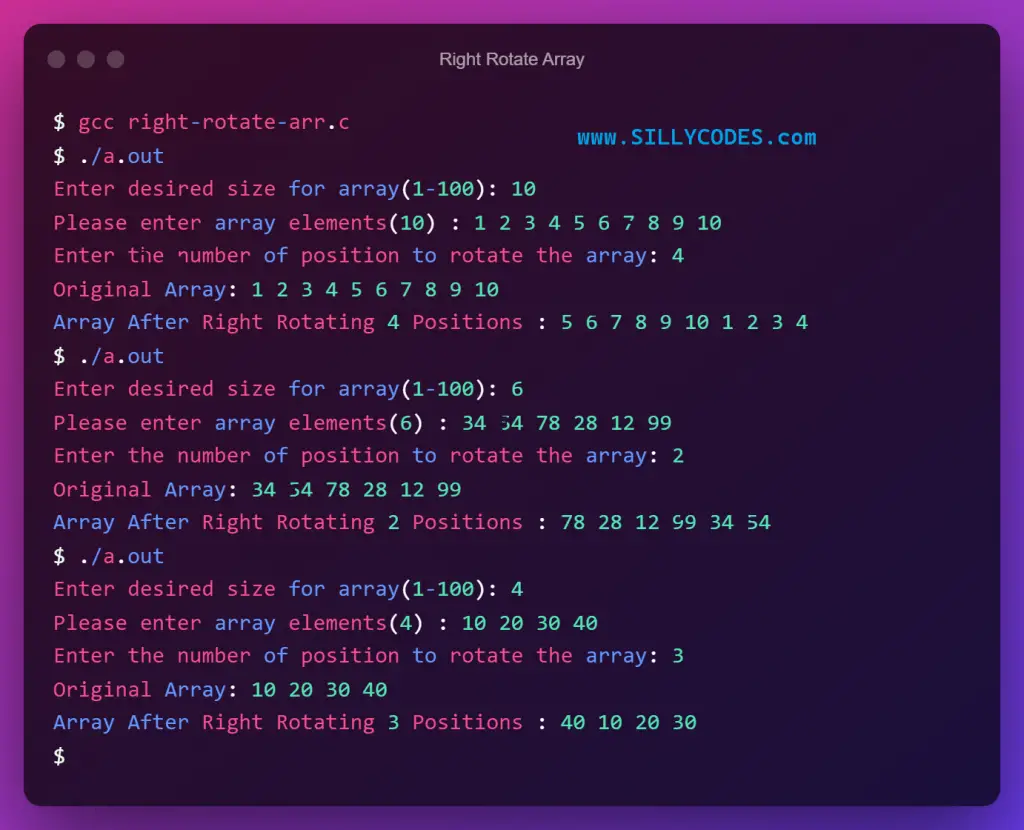
As we can see from the above outputs, The program is properly Right Rotating the elements.
For example, When the user provided the 10 20 30 40 array as input and 3as the positions to rotate, The program right-rotated the array and provided the 40 10 20 30 array as the output.
Test 2: When the user enters invalid Sizes
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 0 Invalid Size, Try again $ ./a.out Enter desired size for array(1-100): 455 Invalid Size, Try again $ |
As excepted, when the user enters an invalid size, The program is displaying an error message ( Invalid Size, Try again).
Related Array Programs:
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times