Count Frequency of Each Element in Array in C Language
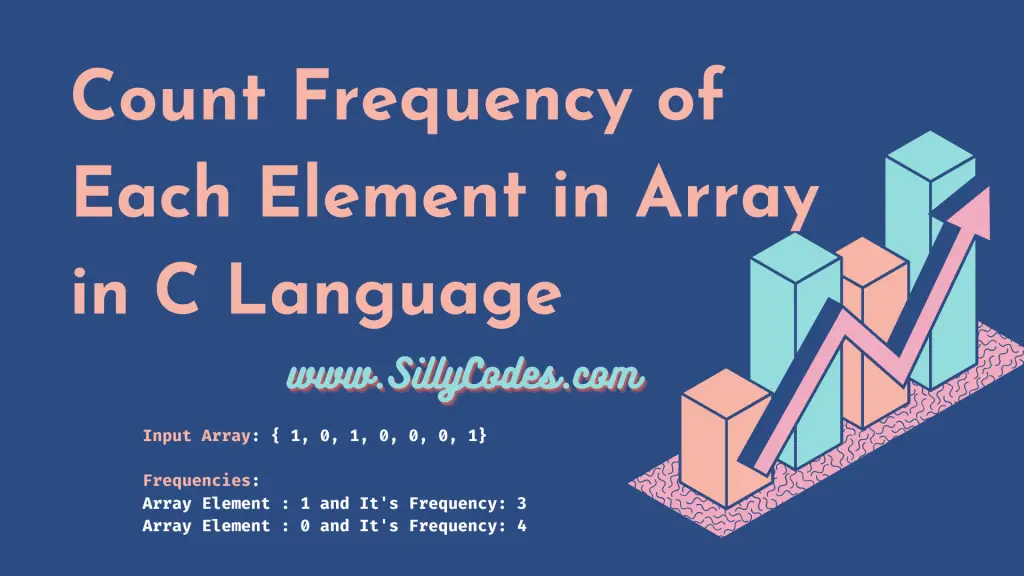
Program Description:
We have looked at the Linear Search in C program in earlier Posts, In today’s post, We will look at the Program to Count Frequency of Each Element in Array in C Programming language. The Program should accept an input array from the user and display the frequency of each element in an array.
Let’s look at the example input and output to better understand the program.
Example Input and Output:
Input:
Enter desired size for array(1-100): 7
Please enter array elements(7) : 1 0 1 0 0 0 1
Output:
Original Array: 1 0 1 0 0 0 1
Array Element : 1 and It's Frequency: 3
Array Element : 0 and It's Frequency: 4
As we can see from the above output, The element 1 is repeated three( 3) times in the input array, Similarly, The element Zero(0) has the frequency of 4 in the array.
Prerequisites:
To better understand the following program, We should know the basics of the Arrays and Functions.
Algorithm for Count Frequency of Each Element in Array in C:
- We are going to use a Counter Array ( countArr), which is going to store the frequency of the element. The counter Array ( countArr) should be the same size as the input array and initialize its elements with -1
- Iterate over that input array (
numbers) and at each iteration,
- Use another for loop to check the frequency of the current element
numbers[i].
- count the frequency of the current element ( count)
- If the countArr[i] == 0, Then we updated the frequency( count) of the current element( numbers[i]).
- Check if the countArr[i] is updated or not, Then update countArr[i] with the calculated frequency( count)
- Use another for loop to check the frequency of the current element
numbers[i].
- Display the results by going through the countArr array.
Let’s look at the step-by-step explanation of the Count Frequency of each element in an array in the C program.
Count Frequency of Each Element in Array in C Program Explanation:
- Declare the input array numbers[100] and the counter array counter[100]. Also, declare other supporting variables.
- Take the desired array size from the user and update the size variable.
- Check if the array size is out of bounds (max size is 100 – change this if you want to use large arrays), If the array is out of bounds, Display an error message and terminate the program using the return statement.
- Take the user input for the
numbers array. Also, initialize all elements of the
countArr with
-1.
- Use a For loop to iterate over the array element and update numbers[i] and countArr[i]
- Print the Input Array Elements on the console by calling the display() helper function.
- Now, To Count the Frequency of Each Element in an Array in C language, We need to traverse through the array elements one by one and check the frequency using another array.
- Create A For Loop (Let’s call it Outer Loop) to iterate over the Input array. ( for(i = 0; i<size; i++) ), At each iteration,
- Update the count = 1 The count variable holds the current element ( numbers[i]) frequency.
- Create another For Loop(Inner Loop) to count the frequency of the current element. for(int j = i+1; j<size; j++),
- Check if the numbers[i] is equal to numbers[j], If it is true, Then increment the count variable.
- In the Outer Loop, If the countArr[i] is not updated ( if(countArr[i] != 0)), Then update the countArr[i] with current element frequency – countArr[i] = count;
- Repeat the above steps till i reaches the size-1
- Once the above two loops are completed, Then the countArr will contain the frequencies of each element in the array.
- Display the results on the console.
Program to Count Frequency of Each Element in Array in C Language:
Here is the program to count frequency of each element in array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 |
/*     Program to Count frequency of each element of the array in c     Author: SillyCodes.com */  #include <stdio.h>  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }  int main() {     // declare the 'numbers' array     int numbers[100], countArr[100] = {0};     int i, count=0, size;      // Take the Array1('numbers') details from the user     printf("Enter desired size for array(1-100): ");     scanf("%d", &size);         // check for array bounds     if(size >= 100 || size <= 0)     {         printf("Invalid Size, Max Array size reached, Try again \n");         return 0;     }      // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);         countArr[i] = -1;     }      // Print the 'numbers' array     printf("Original Array: ");     display(numbers, size);      // Iterate over the Original Array and      for(i = 0; i<size; i++)     {         count = 1;         for(int j = i+1; j<size; j++)         {             if(numbers[i] == numbers[j])             {                 // increment the 'count'                 count++;                  // Avoid counting same number frequency, Again                 countArr[j] = 0;             }         }          // update the current element frequency         if(countArr[i] != 0)         {             countArr[i] = count;         }     }         // Display the frequencies     for(i=0; i<size; i++)     {         if(countArr[i] != 0)         {             printf("Array Element : %d and It's Frequency: %d \n", numbers[i], countArr[i]);         }     }      return 0; } |
In the above program, We have used display() function to print the array elements on the console.
Also note, That while printing the frequencies of all array elements, We are considering the numbers and countArr elements.
1 |
printf("Array Element : %d and It's Frequency: %d \n", numbers[i], countArr[i]); |
Program Output:
Let’s Compile and Run the program using GCC compiler (Any Compiler)
Test 1: Provide arrays with duplicate elements.
1 2 3 4 5 6 7 8 9 10 |
$ gcc freq-ele.c $ ./a.out Enter desired size for array(1-100): 10 Please enter array elements(10) : 1000 6000 2000 3000 1000 6000 3000 1000 6000 1000 Original Array: 1000 6000 2000 3000 1000 6000 3000 1000 6000 1000 Array Element : 1000 and It's Frequency: 4 Array Element : 6000 and It's Frequency: 3 Array Element : 2000 and It's Frequency: 1 Array Element : 3000 and It's Frequency: 2 $ |
From the above output, We can see the input array is 1000 6000 2000 3000 1000 6000 3000 1000 6000 1000 and the frequency of the each elements are
1 2 3 4 |
Array Element : 1000 and It's Frequency: 4 Array Element : 6000 and It's Frequency: 3 Array Element : 2000 and It's Frequency: 1 Array Element : 3000 and It's Frequency: 2 |
Let’s try few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ ./a.out Enter desired size for array(1-100): 5 Please enter array elements(5) : 1 2 3 4 5 Original Array: 1 2 3 4 5 Array Element : 1 and It's Frequency: 1 Array Element : 2 and It's Frequency: 1 Array Element : 3 and It's Frequency: 1 Array Element : 4 and It's Frequency: 1 Array Element : 5 and It's Frequency: 1 $ ./a.out Enter desired size for array(1-100): 7 Please enter array elements(7) : 1 0 1 0 0 0 1 Original Array: 1 0 1 0 0 0 1 Array Element : 1 and It's Frequency: 3 Array Element : 0 and It's Frequency: 4 $ |
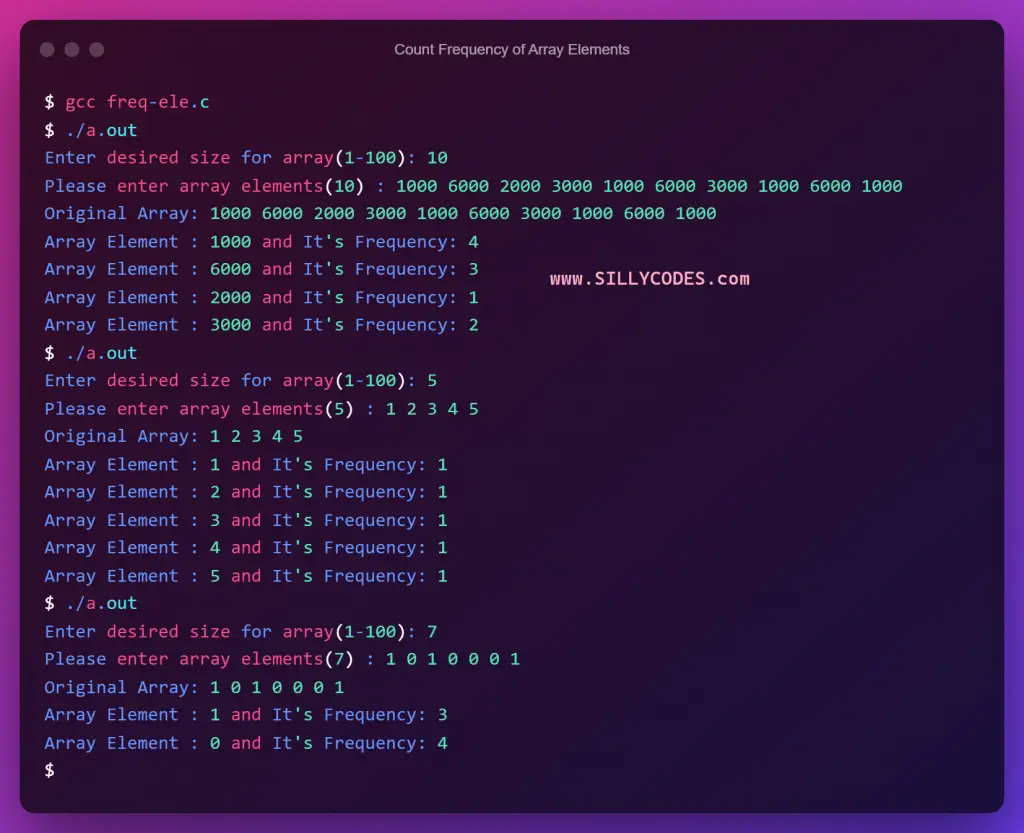
Test 2: Negative tests – with Invalid Size:
1 2 3 4 5 6 7 8 |
// Negative Tests $ ./a.out Enter desired size for array(1-100): 340 Invalid Size, Try again $ ./a.out Enter desired size for array(1-100): 0 Invalid Size, Try again $ |
As excepted Program is displaying an error message( Invalid Size, Try again) if the size goes beyond the limits.
Related Array Programs:
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
- Linear Search in C Language with Example Programs