Implement Custom atoi function in C Language
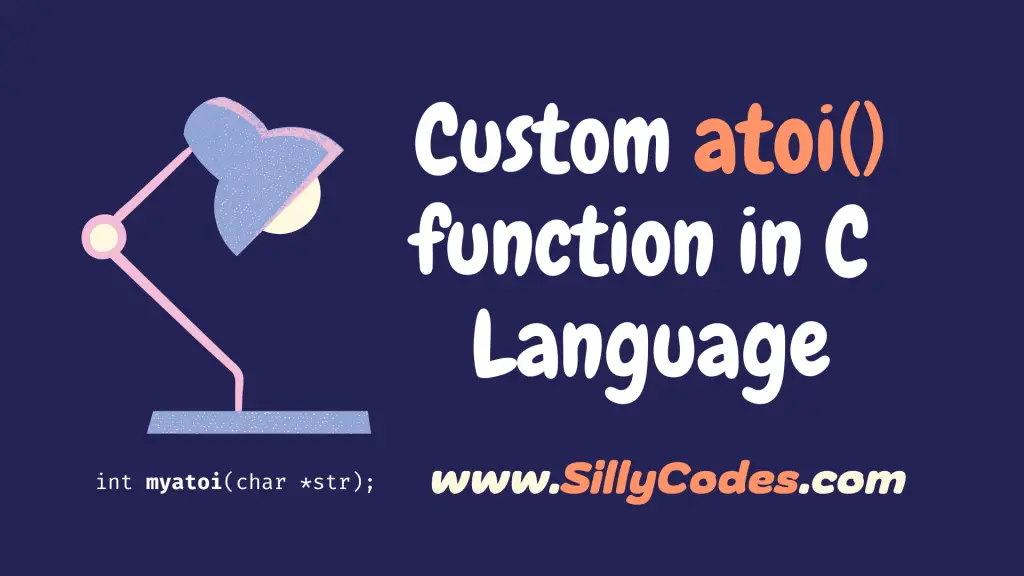
Custom atoi function in C Program Description:
Write a Program to implement custom atoi function in c programming language. We are going to write our own atoi function in c. The function should take an ASCII string from the user and convert it into an Integer Number.
If the user enters an invalid ASCII string, Then the function should return Zero(0). If the ASCII string is valid, Then it should convert the string into an integer number.
The program should also take the sign into the consideration.
Here are the expected input and output of the program.
Excepted Input and Output:
Input:
Enter a string : -8434
Output:
Input String: '-8434'
Atoi Converted Integer Value: -8434
The input string contains the negative sign and the output integer is also a negative number.
Prerequisites:
It is recommended to know the basics of the C-Strings, C-Arrays, and Functions in C to better understand the following program.
Algorithm to Implement custom atoi function in C Language:
We have defined a function called myatoi() in the following program. Here is the syntax of the myatoi() function.
int myatoi(char *str);
This function takes a string as the input and returns the integer equivalent of it.
Let’s look at the Algorithm to implement the custom atoi function in c:
- Create the necessary variables, The result variable will store the converted string. Initialize it with Zero(0). The sign variable is used to update the resultant number sign, So initialize it with 1. Also, create the variable i which is used to traverse through the string. initialize it with Zero(0)
- Check if the first character in the input string str is a sign i.e -. If so, Then update the sign variable with -1. Increment the i by 1.
- Start traversing the string element by element using the while loop,
- At Each iteration, Check if the str[i] is a valid digit, If not, Then return Zero(0). use (str[i] < ‘0’ || str[i] > ‘9’) condition to check for digit.
- Update the result variable by removing the ASCII Value of the Zero(0) from the str[i]. – i.e result = result * 10 + str[i] – ‘0’;
- Finally, Increment the i.
- Once the above Step-3 is completed, The resultwill contain the converted integer ( ASCII to Integer converted value). Finally, update the sign of the result by multiplying it with sign variable – i.e result = result * sign;
- Return the result back to the caller.
Now, Call the myatoi() function from the main() function and pass the input string. Store the return value in the result variable.
int result = myatoi(str);
Finally, Check the return value of the myatoi() function, Do note that, The myatoi() function returns Zero(0), If the input string contains any invalid characters like non-digit characters.
So check if the result is equal to Zero(0). If it is true, Then the input string str is not valid, Display the error message on the console.
Otherwise, The myatoi() function is able to convert the given ASCII String into an Integer number. Display the resulton the console.
Program to Implement custom atoi function in c Language:
Here is the Program to convert the ASCII string into an integer number using the custom/own atoi() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
/* program implement your own atoi function in c sillycodes.com */ #include <stdio.h> // Max Size of string #define SIZE 100 /** * @brief - Convert string to Integer * * @param str - Input string * @return int - On success returns coverted string, 0 on failure */ int myatoi(char *str) { int result = 0; int sign = 1; int i = 0; // check first character if(str[i] == '-') { // update the sign sign = -1; i++; } // Iterate over the string while(str[i]) { if(str[i] < '0' || str[i] > '9') { return 0; } // update the result result = result * 10 + str[i] - '0'; // increment 'i' i++; } // finally, update sign result = result * sign; return result; } int main() { // declare input strings with 'SIZE' char str[SIZE]; // Take the string from the user printf("Enter a string : "); gets(str); printf("Input String: '%s'\n", str); // call 'myatoi' function int result = myatoi(str); // check results and print results if(result == 0) { // Invalid input or Zero value printf("Invalid String or Zero. Please enter valid string to convert\n"); } else { // Success: Converted integer printf("Atoi Converted Integer Value: %d\n", result); } return 0; } |
Program Output:
Let’s compile and Run the program using your favorite compiler. We are using the GCC compiler on Ubuntu Linux.
Compile the Program.
$ gcc atoi-custom.c
The above command generates the a.out executable file, Run the executable file.
Test Case 1: When the input string is valid:
1 2 3 4 5 |
$ ./a.out Enter a string : 3992 Input String: '3992' Atoi Converted Integer Value: 3992 $ |
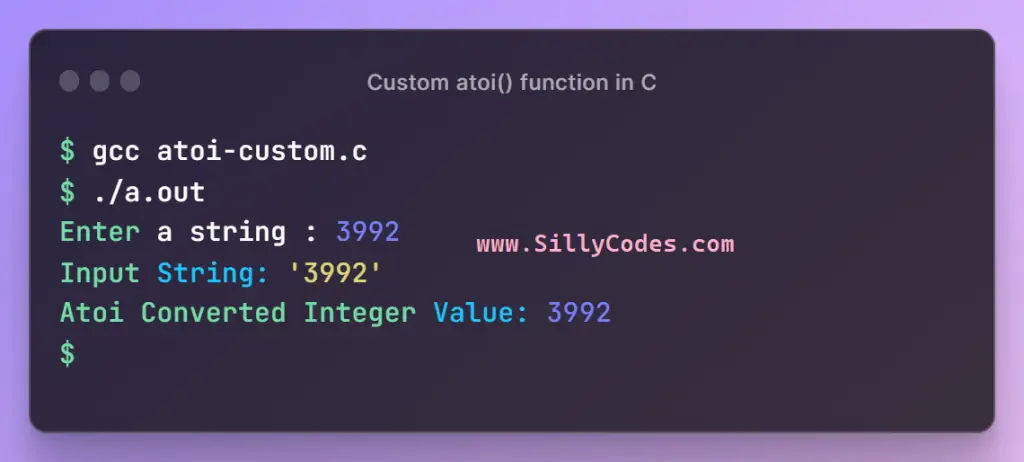
As we can see from the above output, The user provided the input ASCII string as "3992", and the program is able to convert the ASCII string to an Integer number and returned the result as 3992 ( Integer).
Let’s look at another example.
1 2 3 4 5 |
$ ./a.out Enter a string : 123456 Input String: '123456' Atoi Converted Integer Value: 123456 $ |
As we can see from the above output, The input string “ 123456” has been converted successfully to an integer 123456
Test Case 2: When the input string has a sign
1 2 3 4 5 6 |
// Test 2 $ ./a.out Enter a string : -5679 Input String: '-5679' Atoi Converted Integer Value: -5679 $ |
In this case, The input has the negative sign and the program is converted the string with the sign properly and returned the negative integer number ( -5679).
Test Case 3: Invalid string:
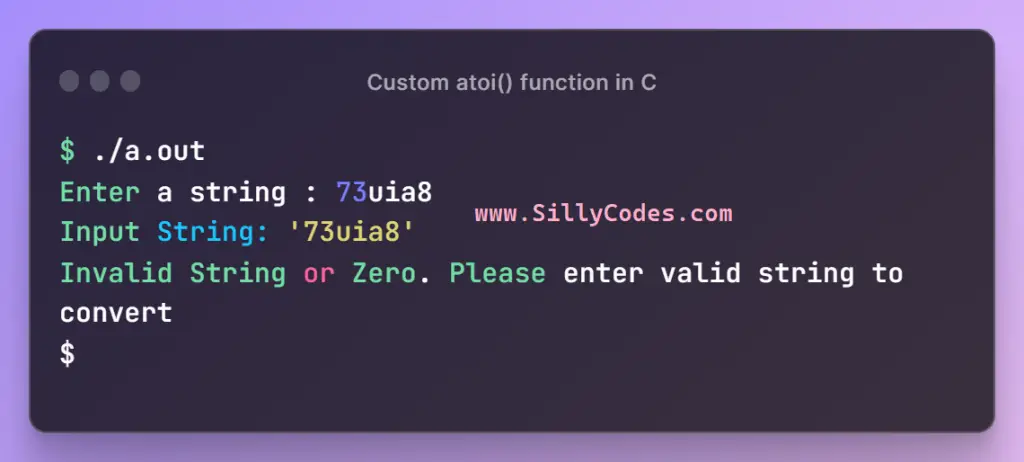
In this case, The user provided the invalid ASCII string, i.e the ASCII string has non-digit characters. So myatoi() function returned the Zero(0), which means the invalid input.
Similar String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
- C Program to Find All Occurrences of a character in string
- C Program to Count All Occurrences of character in string
- C Program to Remove First Occurrence of character from string
- C Program to Remove Last Occurrence of character from string
- C Program to Remove All Occurrences of character from string
- C Program to Swap two strings
- C Program to Count Number of Words in a String