Find Last Occurrence of a Character in String in C Language
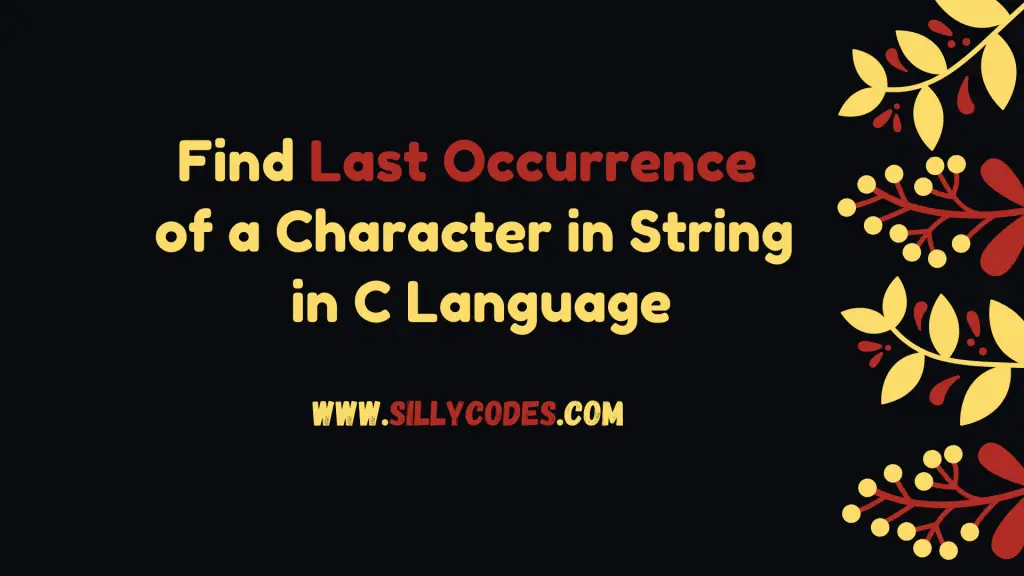
Program Description:
Write a Program to Find the last occurrence of a character in string in c Programming language. The program should accept a string and a character from the user and find the last occurrence of the given character in the string.
This program is case-sensitive. So the uppercase characters and Lowercase characters are different.
📢 This Program is part of the C String Practice Programs series
We will look at a few methods to search for the last occurrence of a character in the string program. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
Here are the excepted input and output.
Example Input and Output:
Input:
Enter a string : proproproprogrammer
Enter character to search in the string(Last Occurance): o
Output:
FOUND: Last Occurrence of 'o' character is found at 11 index in given string
The last occurrence of the character o is at the index 11 in the input string proproproprogrammer
Prerequisites:
It is recommended to go through the following articles to understand the program better.
- Strings in C Language
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Declare and Use Functions
We will look at the three different methods to find the last occurrences of the character in a string in C Language.
- Iterative Method
- User-defined Functions
- Library Function ( strrchr library function)
Find Last Occurrence of a Character in String in C Program Explanation:
- Start the program by defining a Macro to hold the max size of the array – #define SIZE 100
- Declare the input string( inputStr) with the SIZE and the character to search (ch).
- Initialize a variable named lastIndex with the -1. The lastIndex variable is used to track the last occurrence of the character.
- Prompt the user to provide the input string( inputStr) and Read it using the gets function.
- Also, take the character to be searched and store the character in ch variable.
- To search for the Last occurrence of the character( ch) in a string( inputStr), Iterate over the string and check all characters in the string.
- Create a For loop by initializing i to 0. It will continue looping as long as the current character (
inputStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( inputStr[i]) is equal to ch- i.e if(inputStr[i] == ch)
- If the above condition is true, Update the lastIndex variable with the current index( i) – i.e lastIndex = i;
- If the condition is false, Then continue to the next iteration.
- Increment control variable i by 1.
- Once the above loop is completed, Check if the lastIndex variable still has the -1 value ( lastIndex == -1), If the lastIndex value is -1, Then the character ch is NOT FOUND in the given string( inputStr).
- If the lastIndex value is updated, Then the character ch is FOUND in the string and the last occurrence index is stored in the lastIndex variable. Display the FOUND message on the console along with the last occurrence index.
- Stop the program.
Method 1: First Occurrence of a Character in String in C Program (Iterative Method):
Here is the program to search for a last occurrence character in a string in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/* program to Search for a Last Occurrence of a character in a string in C sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 int main() { // declare a string with 'SIZE' char str[SIZE]; char ch; int i; // create a variable to store last Occurrence int lastIndex = -1; // Take the string from the user printf("Enter a string : "); gets(str); // Also take the search character printf("Enter character to search in the string(Last Occurrence ): "); scanf(" %c", &ch); // Iterate over the string for(i = 0; str[i]; i++) { // check if the current character is equal to target('ch') character if(str[i] == ch) { // update the 'lastIndex' to store the last character Occurrence lastIndex = i; } } // check if the 'lastIndex' is updated if(lastIndex == -1) { // 'lastIndex' is not updated. So character not found. printf("NOT FOUND: Character '%c' is not found in the given string\n", ch); } else { // Found the character printf("FOUND: Last Occurance of '%c' character is found at %d index in given string\n", ch, lastIndex); } return 0; } |
Program Output:
Compile the program using your compiler. We are using the GCC compiler on Ubuntu (Linux) Operating system. Here are the instructions to compile and run the programs in Linux using the GCC compiler.
gcc search-last-char.c
Run the program.
1 2 3 4 5 |
$ ./a.out Enter a string : www.sillycodes.com Enter character to search in the string(Last Occurrence): l FOUND: Last Occurrence of 'l' character is found at 7 index in given string $ |
As we can see from the above output, The character l is found multiple times in the input string www.sillycodes.com. The program properly detected the last occurrence of the character l. i.e Last occurrence of the character l is found at the index 7.
Let’s check another example.
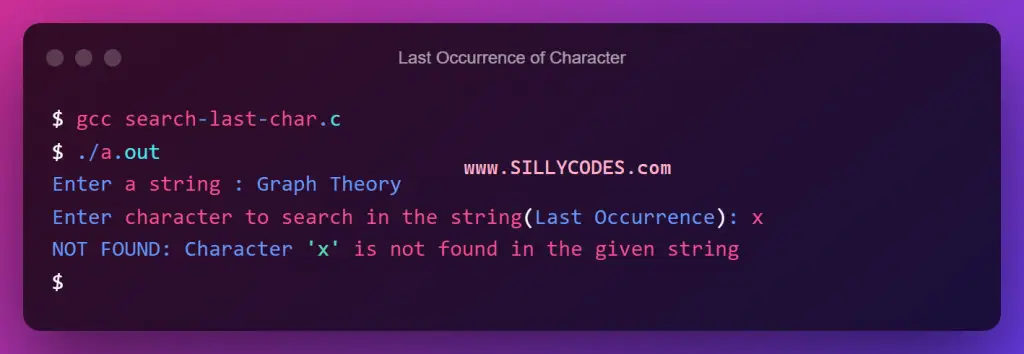
1 2 3 4 5 |
$ ./a.out Enter a string : Graph Theory Enter character to search in the string(Last Occurrence): x NOT FOUND: Character 'x' is not found in the given string $ |
In the above example, The character x is not found in the input string.
Method 2: Search for Last Occurrence of a Character in String using user-defined functions in C:
In the previous method, We have written the complete program inside the main() function. It is not a recommended method, Let’s rewrite the above program to use a function to search for the last occurrence of the character in a string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the rewritten version of the program to search for the last occurrence of a character in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/* program to Search for a Last Occurrence of a character in a string in C sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 /** * @brief - Search the for a character and return last Occurrence of character index * * @param str - Input String * @param ch - Character to search * @return int - If 'ch' is found, Returns index of last Occurrence. Otherwise, Returns -1 */ int searchLastChar(char *str, char ch) { int i; int lastIndex = -1; // Iterate over the string for(i = 0; str[i]; i++) { // check if the current character is equal to target('ch') character if(str[i] == ch) { // update the 'lastIndex' to store the last character Occurrence lastIndex = i; } } // return the 'lastIndex' return lastIndex; } int main() { // declare a string with 'SIZE' char str[SIZE]; char ch; // Create a variable to store last Occurrence int lastIndex = -1; // Take the string from the user printf("Enter a string : "); gets(str); // Also take the search character printf("Enter character to search in the string(Last Occurance): "); scanf(" %c", &ch); // call the 'searchLastChar' with 'str' and 'ch' to search for last Occurrence of the 'ch' lastIndex = searchLastChar(str, ch); // check if the 'lastIndex' if(lastIndex == -1) { // 'lastIndex' is not updated. So character not found. printf("NOT FOUND: Character '%c' is not found in the given string\n", ch); } else { // Found the character printf("FOUND: Last Occurrence of '%c' character is found at %d index in given string\n", ch, lastIndex); } return 0; } |
We have defined a function called searchLastChar() to find the last occurrence of the character. Here is the prototype of the searchLastChar function
int searchLastChar(char *str, char ch)
This function takes two formal arguments and returns a integer value.
- The first argument is a character pointer( char *) which pointers to the input string.
- The second argument is character( char) to be searched.
It returns
- -1 if the character ch is not found in the input string str
- last index of the character ch, If it is found in the input string str.
Finally, Call the searchLastChar() function with the input string and character to be searched and display the result.
1 |
lastIndex = searchLastChar(str, ch); |
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 |
$ gcc search-last-char-func.c $ ./a.out Enter a string : motor sports Enter character to search in the string(Last Occurrence): r FOUND: Last Occurrence of 'r' character is found at 9 index in given string $ |
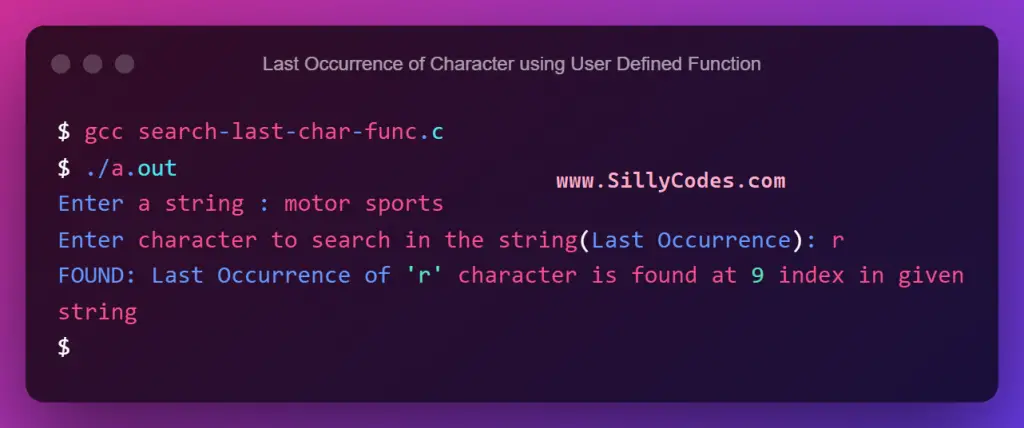
The character r is found in the input string and the last occurrence index is 9.
1 2 3 4 5 |
$ ./a.out Enter a string : programming Enter character to search in the string(Last Occurrence): y NOT FOUND: Character 'y' is not found in the given string $ |
The character y is not found in the given string( programming).
Method 3: Last Occurrence of a Character in String using strrchr library function in C:
In the above method, We used a custom function to find the last occurrence of the character in a string. But the Standard C library provides a library function strrchr() to find the last occurrence of the character.
Here is the syntax of the strrchr function
1 |
[crayon-6692450bc51d9224143646 inline="true" ]char *strrchr(const char *<em>s</em>tr, int <em>c</em>h); |
Here is the program to find the last occurrence of character in a string using the strrchr() function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/* program to Search for a Last Occurrence of a character in a string in C using strrchr function sillycodes.com */ #include<stdio.h> #include<string.h> // Max Size of string const int SIZE = 100; int main() { // declare a string with 'SIZE' char str[SIZE]; char ch; // Create a variable to store last Occurrence int lastIndex = -1; // Take the string from the user printf("Enter a string : "); gets(str); // Also take the search character printf("Enter character to search in the string(Last Occurrence): "); scanf(" %c", &ch); // call the 'strrchr' with 'str' and 'ch' to search for last Occurrence of the 'ch' char * res = strrchr(str, ch); // check if the 'res' if(res == NULL) { // 'res' is NULL. So character not found. printf("NOT FOUND: Character '%c' is not found in the given string\n", ch); } else { // Found the character printf("FOUND: Last Occurrence of Character '%c' is Found in the '%s' string \n", ch, str); printf("Result is : %s \n", res); } return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc search-last-strrchr.c $ ./a.out Enter a string : go go go george Enter character to search in the string(Last Occurrence): o FOUND: Last Occurrence of Character 'o' is Found in the 'go go go george' string Result is : orge $ $ ./a.out Enter a string : formula 1 Enter character to search in the string(Last Occurrence): 2 NOT FOUND: Character '2' is not found in the given string $ |
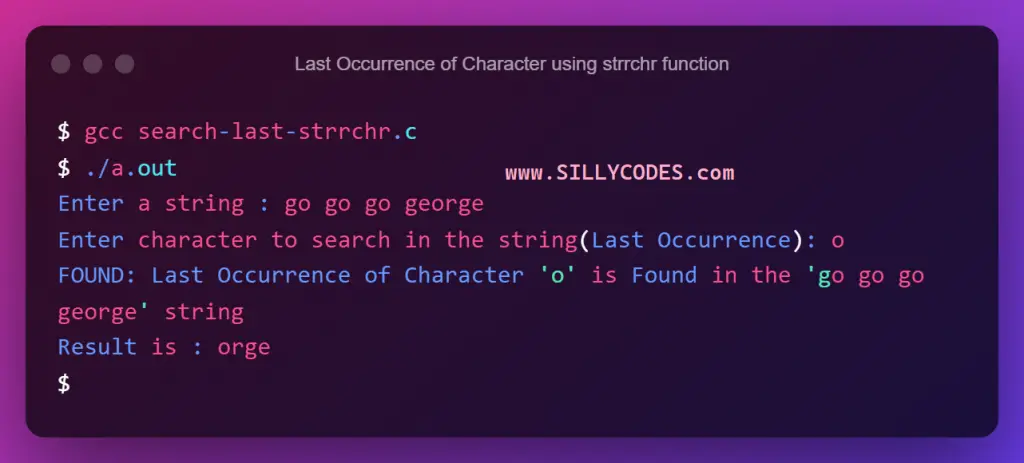
As we can see from the above output, The program is generating the desired results.
Related String Programs:
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
1 Response
[…] C Program to Find Last Occurrences of a character in a string […]