Swap Two Strings in C Language
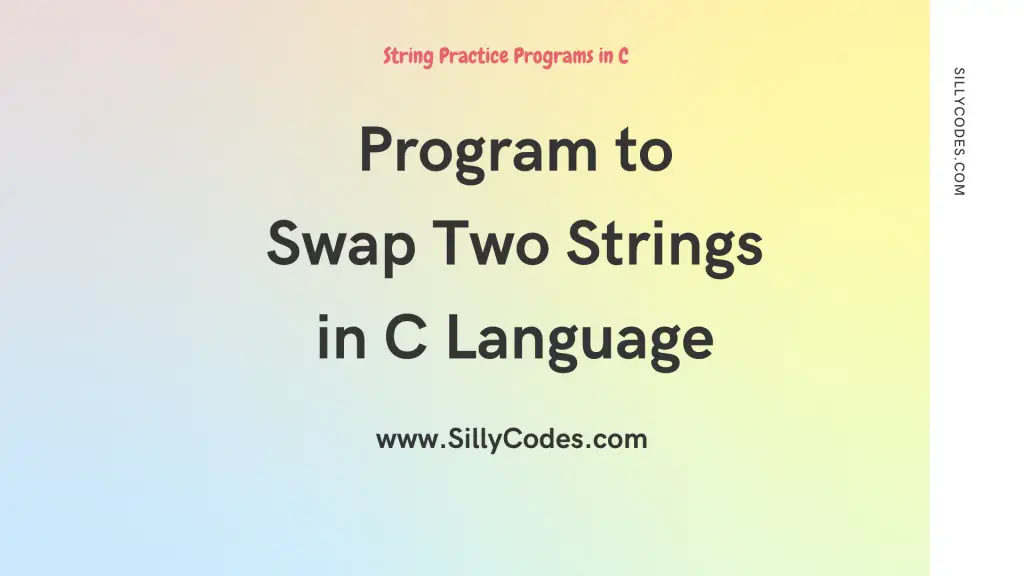
Program Description:
Write a Program to swap two strings in c programming language. The program should accept two strings from the user and swap the contents of the two strings.
📢 This Program is part of the C String Practice Programs series
We are going to look at a couple of methods to swap two strings in C and Each program will be followed by a detailed step-by-step explanation of the program and program output. We also provided instructions for compiling and running the program using your compiler.
Let’s look at the example input and output of the program.
Excepted Input and Output:
Program Input:
Enter the first string : Git
Enter the second string: SVN
Program Output:
Strings before Swapping: str1:'Git' and str2:'SVN'
Strings After Swapping: str1:'SVN' and str2:'Git'
The input strings content are swapped.
Prerequisites:
It is recommended to know the basics of the Strings and Functions in C language. Please go through the following articles if you want to refresh your concepts.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Swap Two Strings in C using string library functions:
In this method, We are going to use the strcpy library function to copy the strings. Let’s look at the step by step explanation of the program.
Program Explanation: Swap Two Strings in C:
- Start the program by creating a Macro named SIZE with the value 100. The SIZE macro holds the max size of the string.
- Declare two strings. Let’s say these strings as str1 and str2. The size of the strings is equal to the SIZE macro. We use these strings to store the input strings. – char str1[SIZE], str2[SIZE];
- Initialize another string called temp with the same size as the above strings. This temp string is used to swap the above two strings. char temp[SIZE] = {0};
- Take the two strings from the user and update the str1 and str2 variables. We are using the gets() function to read the strings. the gets function doesn’t check the boundaries. so be careful while using the gets function.
- To Swap Two Strings in C, We are going to use a temporary string. Here is the process to swap to strings.
- First of all, copy the str1 string to the temp string using strcpy function. This is the backup of str1 string.
- Then copy the contents of the str2 string to the str1 string. At this point, the characters of str1 will be overwritten by the str2 string. So Now the str1 is holding the str2.
- Finally, Copy the temp string to the str2 string. Now, str2 contains the initial str1 string.
- Once the above steps 6, 7, and 8 are completed, The strings str1 and str2 are swapped successfully.
- Print the swapped strings on the console using printf function.
Program to Swap two strings using strcpy function:
Let’s convert the above algorithm to the c program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     program Swap to strings in c     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare two input strings with 'SIZE'     char str1[SIZE], str2[SIZE];      // declare and intialize a temparory string     char temp[SIZE] = {0};      // Take the first string from the user     printf("Enter the first string : ");     gets(str1);      // Take the second string from user     printf("Enter the second string: ");     gets(str2);      printf("Strings before Swapping: str1:'%s' and str2:'%s'\n", str1, str2);      // copy first string('str1') to 'temp'     strcpy(temp, str1);     // copy second string 'str1' to first string 'str1'     strcpy(str1, str2);     // copy 'temp' to second string 'str2'     strcpy(str2, temp);      printf("Strings After  Swapping: str1:'%s' and str2:'%s'\n", str1, str2);      return 0; } |
Program Output:
Let’s compile and run the program. We are using the GCC compiler on Ubuntu Linux.
1 2 3 4 5 6 7 8 |
$ gcc swap-two-strings.c $ $ ./a.out Enter the first string : Investor Enter the second string: Trader Strings before Swapping: str1:'Investor' and str2:'Trader' Strings After  Swapping: str1:'Trader' and str2:'Investor' $ |
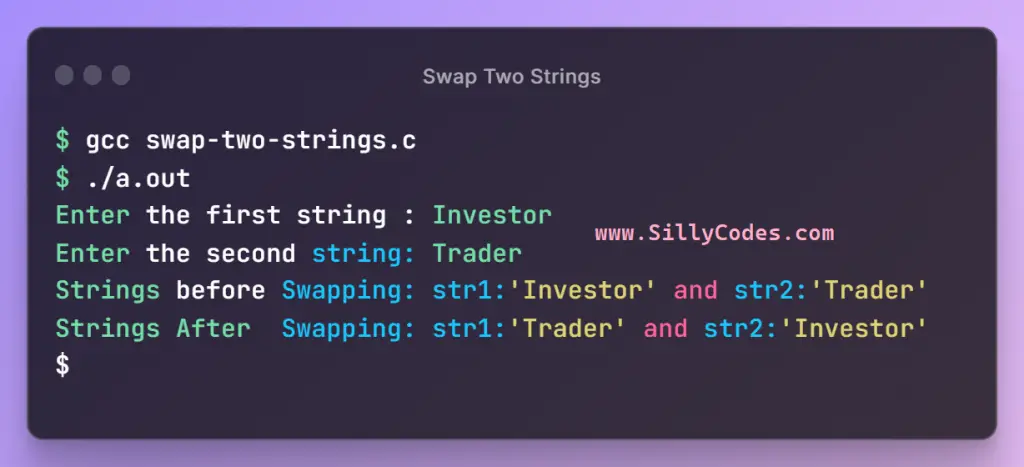
As we can see from the above output, The input strings Investor and Trader has been swapped successfully.
Let’s look at another example.
1 2 3 4 5 6 |
$ ./a.out Enter the first string : Lewis Hamilton Enter the second string: George Russell Strings before Swapping: str1:'Lewis Hamilton' and str2:'George Russell' Strings After  Swapping: str1:'George Russell' and str2:'Lewis Hamilton' $ |
The input strings Lewis Hamilton and George Russell have been swapped and str1 contains George Russell and str2 contains Lewis Hamilton.
Program to Swap Two String in C without using library Functions:
We are not going to use library functions to swap the strings in this method. So we need to implement our own string copy function. We have already discussed the custom string copy function in our earlier posts.
Swap Two strings without using library function program explanation:
Create a function called mystrcpy to copy a string to another string. Here is the prototype of the function.
char * mystrcpy(char *dest, char *src)
The mystrcpy() function takes two formal arguments. The destination string(dest) and source string(src). This function copies the src string to the dest string.
It also returns the copied string back to the caller.
This function Iterates over the Original String using a For Loop and Copy elements from source string to destination string.
Here is program to Swap two strings using custom user defined strcpy function (i.e mystrcpy)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
/*     program Swap to strings in c using custom strcpy function     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  /** * @brief  - Custom strcpy function - Copy the 'src' string to 'dest' string * * @param dest - destination string(pointer) * @param src  - Pointer pointing to source string * @return char* - Copied String ('dest' string) */ char * mystrcpy(char *dest, char *src) {     int i = 0;     // Iterate through the 'src' string and copy character by character to 'dest'     for(i=0; src[i] != '\0'; i++)     {         // copy the character from 'src' to 'dest'         dest[i] = src[i];     }     // Finally add the terminating NULL character     dest[i] = '\0';     // return the 'dest' string     return dest; }  int main() {      // declare two input strings with 'SIZE'     char str1[SIZE], str2[SIZE];      // declare and intialize a temparory string     char temp[SIZE] = {0};      // Take the first string from the user     printf("Enter the first string : ");     gets(str1);      // Take the second string from user     printf("Enter the second string: ");     gets(str2);      printf("Strings before Swapping: str1:'%s' and str2:'%s'\n", str1, str2);      // Call the custom string copy function 'mystrcpy'     // copy first string('str1') to 'temp'     mystrcpy(temp, str1);     // copy second string 'str1' to first string 'str1'     mystrcpy(str1, str2);     // copy 'temp' to second string 'str2'     mystrcpy(str2, temp);      printf("Strings After  Swapping: str1:'%s' and str2:'%s'\n", str1, str2);      return 0; } |
Finally, We replaced the strcpy() function calls in the main() function with the mystrcpy() function.
1 2 3 4 5 6 7 |
// Call the custom string copy function 'mystrcpy' // copy first string('str1') to 'temp' mystrcpy(temp, str1); // copy second string 'str1' to first string 'str1' mystrcpy(str1, str2); // copy 'temp' to second string 'str2' mystrcpy(str2, temp); |
Program Output:
Compile the program.
gcc swap-two-strings-func.c
Run the program.
1 2 3 4 5 6 |
$ ./a.out Enter the first string : USA Enter the second string: UK Strings before Swapping: str1:'USA' and str2:'UK' Strings After  Swapping: str1:'UK' and str2:'USA' $ |
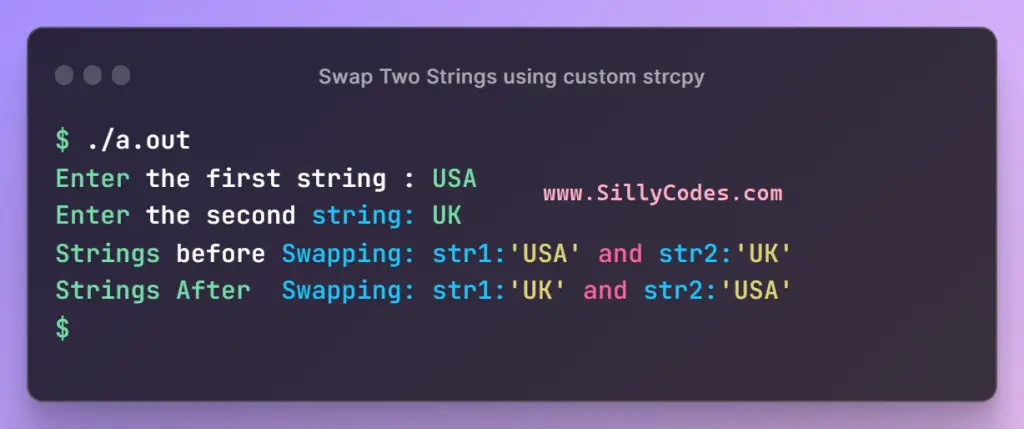
As we can see from the above output, The input string USA and UK are swapped.
1 2 3 4 5 6 |
$ ./a.out Enter the first string : Git Enter the second string: SVN Strings before Swapping: str1:'Git' and str2:'SVN' Strings After  Swapping: str1:'SVN' and str2:'Git' $ |
The program is properly SWAPPING the given strings.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string
- C Program to Find All Occurrences of a character in string
- C Program to Count All Occurrences of character in string
- C Program to Remove First Occurrence of character from string
- C Program to Remove Last Occurrence of character from string
- C Program to Remove All Occurrences of character from string
1 Response
[…] an earlier article, We looked at how to Swap two strings in C. In today’s article, We will look at the anagram program in c language. The program should accept […]