Find Last Occurrence of substring in string in C
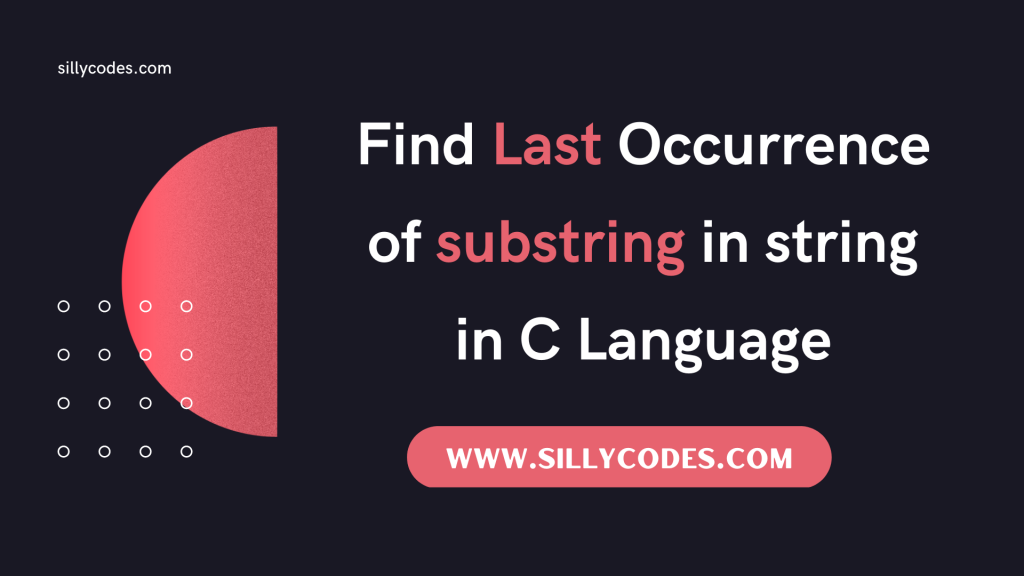
Program Description:
Write a Program to find last occurrence of substring in string in c programming language. The program should accept two strings from the user, one for the main string and one for the substring. The program must then look for the last occurrence of the substring within the main string and return the index of the last occurrence.
📢 This Program is part of the C String Practice Programs series
We are going to look at a few methods to find the last substring and each program will be followed by a detailed step-by-step explanation of the program and program output. We also included the instructions to compile and run the program.
📢 The program is case-sensitive. As a result, upper-case characters will differ from lower-case characters.
Here is the example input and output of the program.
Expected Input and Output:
Input:
Enter a main string : go go go go go lewis
Enter the substring(String to search in main string): go
Output:
FOUND! Last Occurrence of Substring:'go' is found at 12 index in Main String
The last occurrence of the substring go is found in the main string.
Prerequisites:
It is recommended to know the basic of the C-Strings, C-Arrays, and Functions in C to better understand the following program.
We will look at two methods to search for the substring in the main string. They are
- Manual Approach(Iterative) – Where we use an iterative method to search for the sub-string in the main string
- User-defined function – We use a custom user-defined to find the last occurrence of the target string in the main string.
Let’s look at the step-by-step explanation for the iterative program.
Algorithm to Find Last Occurrence of substring in string in C (Manual Method):
- Start the main() function by declaring the two strings and naming them as mainStr and subStr. The size of both strings is equal to SIZE macro.
- Take two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings.
- Initialize a variable called lastStrIdx with the value -1. This variable is used to track the index of the last occurrence of the substring in the main string.
- To search for the last occurrence of substring( subStr) in the main string( mainStr), Iterate through the main string( mainStr), looking for the first character of the substring( subStr[0]). If we found the first character of the substring, we should then determine whether all other characters of the substring match. If that’s the case, we found the substring( subStr) in the main string( mainStr). Similar way we need to look for all occurrences of the substring.
- Create a For loop by initializing variable i with 0 to iterate over the
mainStr. It will continue looping as long as the current character (
mainStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( mainStr[i]) is equal to the first character of the substring( subStr[0]) - i.e if(mainStr[i] == subStr[0])
- If the above condition is
true, Then search for all characters of the
subStr in the
mainStr. We need to use another for loop to iterate over the contents of the
subStr – i.e
for(j=0; subStr[j]; j++).
- Check if the mainStr[k] (where k = i) is not matching to subStr[j], If so then the subString is not found. Otherwise, continue to check all elements of the subStr.
- Once the above loop is completed, Check if we iterated complete subStr ( use j == strlen(substr) ). If we iterated the complete substring( subStr), then we found the match. So update the lastStrIdxvariable with the current index( i). – i.e lastStrIdx = i;
- Continue to the next iteration to search for remaining occurrences of the substring ( subStr).
- If step 5 is completed, Check the value of the
lastStrIdx variable.
- If it is equal to the -1, Then we haven’t found the substring( str) in the main string.
- If the lastStrIdxis updated, Then we found the substring in the main string and the last occurrence of the substring is updated in the lastStrIdx variable.
- Display the results based on the above value.
- Stop the program.
Find Last Occurrence of substring in string in C – Iterative Method:
Here is the program to find the last occurrences of a substring in a main string in C programming language.
Include the string.h header file to access the strlen() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
/*     program to Search for a last occurrence substring in a string in C     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare two strings with 'SIZE'     char mainStr[SIZE], subStr[SIZE];     int i, j, k;     int lastStrIdx = -1;      // Take the main string from the user     printf("Enter a main string : ");     gets(mainStr);      // take the sub string     printf("Enter the substring(String to search in main string): ");     gets(subStr);      // Iterate over the main string     for(i = 0; mainStr[i]; i++)     {         // search for the first character in substring         if(mainStr[i] == subStr[0])         {             // The first character of substring is matched.             // Check if all characters of substring matching.             k = i;             for(j=0; subStr[j]; j++)             {                 if(mainStr[k] != subStr[j])                 {                     // Not matched                     break;                 }                 k++;             }              // check if the 'j' is equal to length of the 'subString', Then we found the match             if(j == strlen(subStr))             {                 lastStrIdx = i;             }          }     }      // check the 'lastStrIdx'     if(lastStrIdx == -1)     {         // 'subStr' is not found         printf("NOT FOUND! Substring:'%s' is NOT found in Main String\n", subStr);     }     else     {         // 'subStr' is found in 'mainStr'         printf("FOUND! Last Occurrence of Substring:'%s' is found at %d index in Main String\n", subStr, lastStrIdx);     }      return 0; } |
Program Output:
Let’s Compile and Run the Program.
Test Case 1: Positive Cases:
1 2 3 4 5 6 |
$ gcc search-last-string.c $ ./a.out Enter a main string : Internet is very very very fast      Enter the substring(String to search in main string): very FOUND! Last Occurrence of Substring:'very' is found at 22 index in Main String $ |
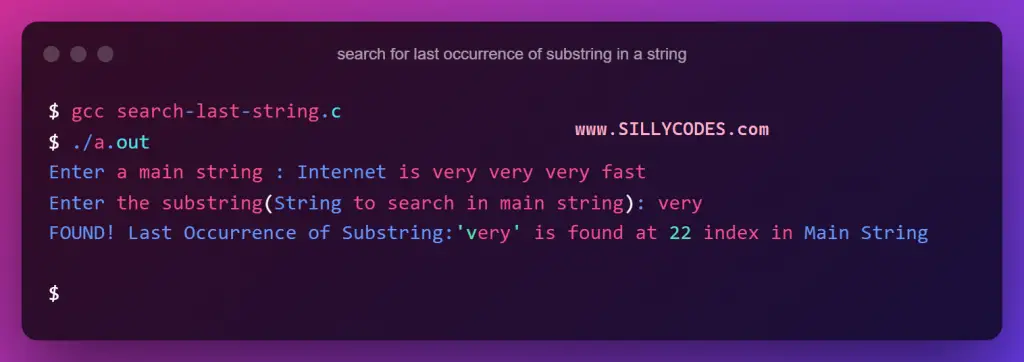
In the above example, The last occurrence of the substring very is found at the 22nd index in the main string. i.e Internet is very very very fast
Let’s look at another example.
1 2 3 4 5 |
$ ./a.out Enter a main string : go go go go go lewis Enter the substring(String to search in main string): go FOUND! Last Occurrence of Substring:'go' is found at 12 index in Main String $ |
As we can see the go substring is found in the main string and the program proerply returned the last occurrence index.
Test case 2: Negative Case:
1 2 3 4 5 6 |
// Negative Case $ ./a.out Enter a main string : Will george be world champion? Enter the substring(String to search in main string): max NOT FOUND! Substring:'max' is NOT found in Main String $ |
In this case, The substring max is not found in the main string Will george be world champion?
Program to Find Last Occurrence of a substring in string using a user-defined function:
In this method, Let’s utilize the C functions to divide the above program into sub-sections. So let’s create a function to find the last occurrence of the substring in the main string. By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the Advantages of the functions in programming.
The Modified program finds the index of the last occurrence of a substring in the string using a user-defined function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
/*     program to Search for a last occurrence substring in a string in C using user defined function     sillycodes.com */  #include <stdio.h> #include <string.h>  // Function declaration int getLastSubStr(char *mainStr, char *subStr);  // Max Size of string #define SIZE 100  int main() {      // declare two strings with 'SIZE'     char mainStr[SIZE], subStr[SIZE];       // Take the main string from the user     printf("Enter a main string : ");     gets(mainStr);      // take the sub string     printf("Enter the substring(String to search in main string): ");     gets(subStr);      // call the 'getLastSubStr' function with 'mainStr' and 'subStr'     int idx = getLastSubStr(mainStr, subStr);      // check the 'idx'     if(idx == -1)     {         // 'subStr' is not found         printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr);     }     else     {         // 'subStr' is found in 'mainStr'         printf("FOUND! Last Occurrence of Substring:'%s' is found at %d index in main string\n", subStr, idx);     }      return 0; }  /** * @brief Get the Index of Last occurrence of SubString(subStr) in main string(mainStr) * * @param mainStr - Main String * @param subStr  - Sub String * @return int    - If 'subStr' found, Then returns Index of last occurrence of 'subStr' in 'mainStr', Otherwise -1 */ int getLastSubStr(char *mainStr, char *subStr) {      int i, j, k;     int lastStrIdx = -1;      // Iterate over the main string     for(i = 0; mainStr[i]; i++)     {         // search for the first character in substring         if(mainStr[i] == subStr[0])         {             // The first character of substring is matched.             // Check if all characters of substring matching.             k = i;             for(j=0; subStr[j]; j++)             {                 if(mainStr[k] != subStr[j])                 {                     // Not matched                     break;                 }                 k++;             }              // check if the 'j' is equal to length of the 'subString', Then we found the match             if(j == strlen(subStr))             {                 lastStrIdx = i;             }          }     }      // return 'lastStrIdx'     return lastStrIdx; } |
We have defined a function named getLastSubStr() function in the above program. This function is used to find the index of the last occurrence of the substring in the main string. Here is the prototype of the function.
1 2 3 4 5 6 7 8 9 |
/** * @brief Get the Index of Last occurrence of SubString(subStr) in main string(mainStr) * * @param mainStr - Main String * @param subStr  - Sub String * @return int    - If 'subStr' found, Then returns Index of last occurrence of 'subStr' in 'mainStr', Otherwise -1 */  int getLastSubStr(char *mainStr, char *subStr) |
The getLastSubStr function takes two character pointers( char *) as the formal arguments. They are mainStr and subStr respectively. It searches for the occurrences of the subStr in the mainStr
This function also returns a Integer value( int) back to the caller. If the subStr is found in the mainStr, It returns the index of the last occurrence of subStr, If the subStr is not found int he mainStr, It returns -1.
In the main() function, Call the getLastSubStr() function with the two strings ( mainStr and subStr) and capture the return value in a variable.
1 2 |
// call the 'getLastSubStr' function with 'mainStr' and 'subStr' int idx = getLastSubStr(mainStr, subStr); |
Finally, display the results on the console based on the return value of the function.
1 2 3 4 5 6 7 8 9 10 11 |
// check the 'idx'     if(idx == -1)     {         // 'subStr' is not found         printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr);     }     else     {         // 'subStr' is found in 'mainStr'         printf("FOUND! Last Occurrence of Substring:'%s' is found at %d index in main string\n", subStr, idx);     } |
Program Output:
Compile and Run the program using GCC compiler (Any compiler)
1 2 3 4 5 6 |
$ gcc search-last-string-func.c $ ./a.out Enter a main string : red lorry yellow lorry Enter the substring(String to search in main string): lorry FOUND! Last Occurrence of Substring:'lorry' is found at 16 index in main string $ |
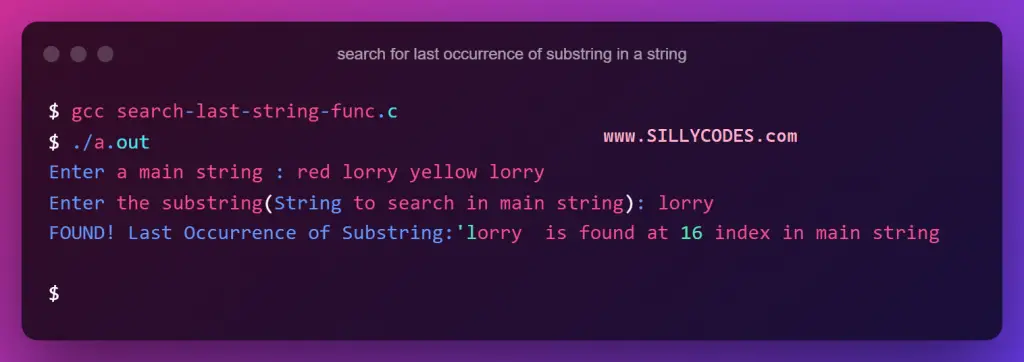
As we can see from the above output, The substring lorry is found multiple times in the main string red lorry yellow lorry. The getLastSubStr function properly calculated the last occurrence index and returned to the main() function.
Similar String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
1 Response
[…] C Program to Find Last Occurrence of Substring/Word in a String […]