Program to Find Lowest Frequency character in a string in C
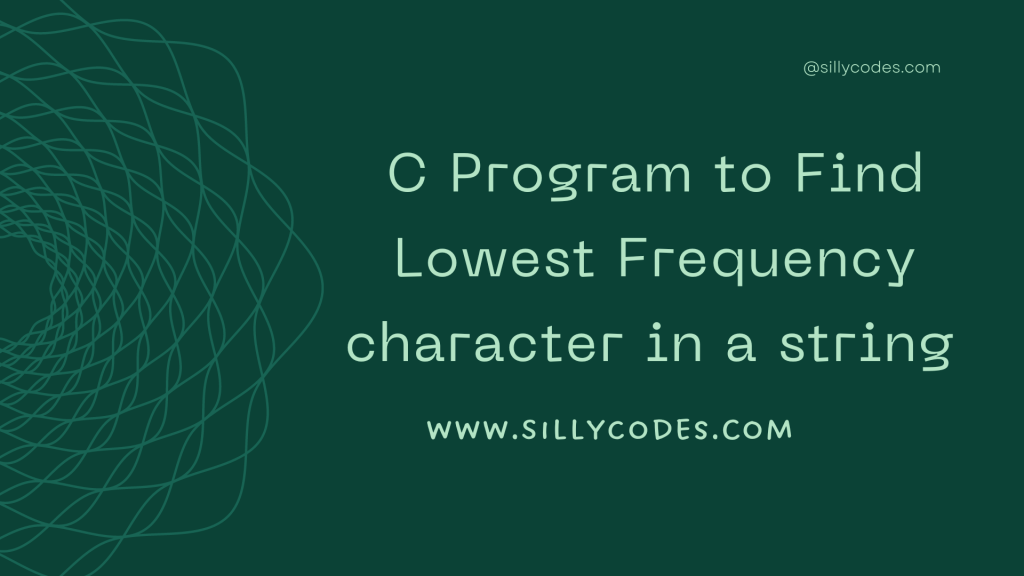
Program Description:
Write a Program to Find Lowest frequency character in a string in C programming language. The program will accept a string from the user and prints the least frequent character in the given string along with the character frequency.
📢 This Program is part of the C String Practice Programs series
We are going to look at a few methods to find the lowest frequency character or least frequent character in a string. Each program will be followed by a detailed step-by-step explanation and program output. We also provided instructions for compiling and running the program using your compiler.
Example Input and Output:
Input:
Enter a string : ABBCCDDD
Output:
Frequency of Characters in given string :
Character : 'A' - Frequency: 1
Character : 'B' - Frequency: 2
Character : 'C' - Frequency: 2
Character : 'D' - Frequency: 3
Character 'A' is the Low Frequency Character(Frequency: 1) in the given string
In the input string ABBCCDDD, The character A is the least frequent character or rarest character.
Prerequisites:
It is recommended to know the C-Strings, C-Arrays, and Functions in C to better understand the following program.
We will look at the two different methods to find the Lowest frequency character in a string in C Language.
- Iterative Method
- Using User-defined Functions
Find the Lowest Frequency character in a string in C Program Explanation:
- Create Two macros to store the string SIZE and ASCIISIZE with values 100 and 256 respectively.
- Declare a string to store the input with the size of SIZE – char inputStr[SIZE];
- Create another string to store the frequencies and initialize it with zeroes. – char frequency[ASCIISIZE] = {0};
- Prompt the user for the input string and read it using the gets function and update the inputStr string.
- To Calculate the Lowest Frequency Character, Iterate over the inputStr and update the frequency array with the frequency of each character in the string. Please look at the Count Frequency of characters in the string program for a detailed explanation.
- Use another loop to iterate over the frequency array and find the Lowest frequency character by updating the lowFreq variable – if(lowFreq > frequency[i]) is true update lowFreq = frequency[i]; and rarech = i
- Once the above two loops are completed, The lowFreq variable will contain the least frequency and rarech character will contain the least frequent character or the rarest character in the string.
- Print the results on the standard Output.
Program to Find Lowest Frequency character in a string in C:
Here is the program to find the rarest character in the string in the c programming language using an iterative method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
/* program to find Low frequency character in a string in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i, lowFreq = SIZE, rareCh = 0; // get the string from the user printf("Enter a string : "); gets(inputStr); // Create a frequency string - Stores count based on ASCII number of string characters char frequency[ASCIISIZE] = {0}; // Iterate through all characters of string // update the frequency array for(i = 0; inputStr[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[inputStr[i]]++; } // Print the Frequency of characters printf("Frequency of Characters in given string : \n"); for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { printf("Character : '%c' - Frequency: %d\n", i, frequency[i]); // check if the present frequency is lower than 'lowFreq' if(lowFreq > frequency[i]) { // update 'lowFreq' lowFreq = frequency[i]; rareCh = i; } } } printf("Character '%c' is the Low Frequency Character(Frequency: %d) in the given string\n", rareCh, lowFreq); return 0; } |
We are also printing the frequencies of all characters to better understand the program.
Program Output:
Let’s compile and run the program. We are using the GCC compile in Ubuntu OS. but you can use any C language compiler.
1 2 3 4 5 6 7 8 9 10 |
$ gcc low-freq.c $ ./a.out Enter a string : ABBCCDDD Frequency of Characters in given string : Character : 'A' - Frequency: 1 Character : 'B' - Frequency: 2 Character : 'C' - Frequency: 2 Character : 'D' - Frequency: 3 Character 'A' is the Low Frequency Character(Frequency: 1) in the given string $ |
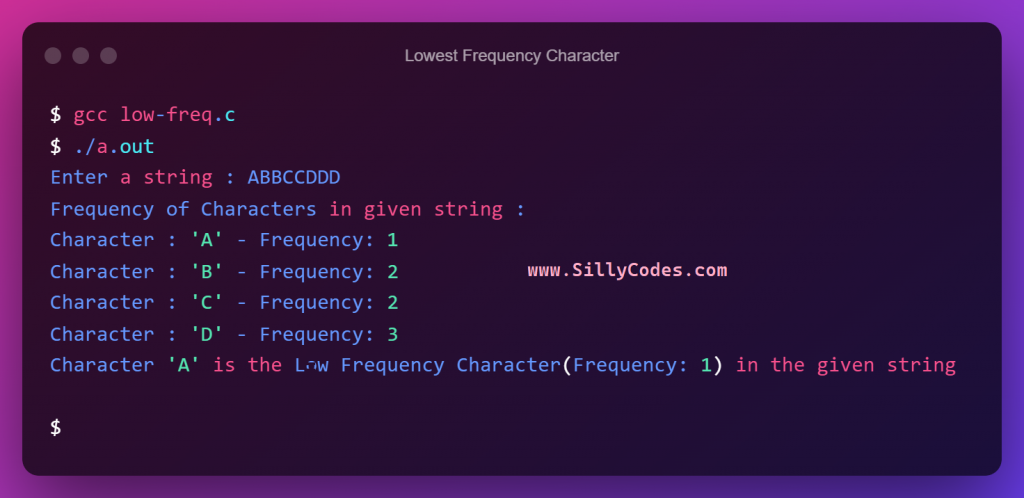
From the above program output, We can confirm that the program is properly calculating the least frequent character or the rarest character in a string.
Let’s try another example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ ./a.out Enter a string : www.SillyCodes.com Frequency of Characters in given string : Character : '.' - Frequency: 2 Character : 'C' - Frequency: 1 Character : 'S' - Frequency: 1 Character : 'c' - Frequency: 1 Character : 'd' - Frequency: 1 Character : 'e' - Frequency: 1 Character : 'i' - Frequency: 1 Character : 'l' - Frequency: 2 Character : 'm' - Frequency: 1 Character : 'o' - Frequency: 2 Character : 's' - Frequency: 1 Character : 'w' - Frequency: 3 Character : 'y' - Frequency: 1 Character 'C' is the Low Frequency Character(Frequency: 1) in the given string $ |
If multiple characters have the same frequency, Then the character with the lowest ASCII value will be returned.
In the above www.SillyCodes.com example, The character C (capital ‘C’)’s ASCII value is the Smallest one Compared to other characters with the frequency of One(1).
Find Lowest Frequency character in a string using user-defined functions in C:
In this method, We will move the logic to find the least frequent character in a string to a new user-defined function. This function will take a string as the input and returns the least frequent character.
Let’s modify the above program to use a custom function named getLowFreqChar to find the lowest frequency character in the string.
Here is the prototype of the getLowFreqChar function
char getLowFreqChar(char * str)
This function takes a input string( str) as a formal argument and computes the least frequency character in string( str) and returns it to the caller.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 |
/* program to find Low frequency character in a string in c sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 #define ASCIISIZE 256 /** * @brief Get the Low Frequency Character in input string(str) * * @param str - Input String * @return char - Returns Minimum or Low frequency character in string ('str') */ char getLowFreqChar(char * str) { int i, lowFreq = SIZE, rareCh = 0; // Create a frequency string - Stores count based on ASCII number of string characters char frequency[ASCIISIZE] = {0}; // Iterate through all characters of string // update the frequency array for(i = 0; str[i]; i++) { // Update the frequency Array. // frequency['a'] = frequency[97] = element at 97th index will be incremented. frequency[str[i]]++; } // Print the Frequency of characters printf("Frequency of Characters in given string : \n"); for(i = 0; i<ASCIISIZE; i++) { if(frequency[i] != 0) { printf("Character : '%c' - Frequency: %d\n", i, frequency[i]); // check if the present frequency is lower than 'lowFreq' if(lowFreq > frequency[i]) { // update 'lowFreq' lowFreq = frequency[i]; rareCh = i; } } } return rareCh; } int main() { // declare a string with 'SIZE' char str[SIZE]; int i,rareCh = 0; // get the string from the user printf("Enter a string : "); gets(str); rareCh = getLowFreqChar(str); printf("Character '%c' is the Low Frequency Character in the given string\n", rareCh); return 0; } |
In the main() function, Take the input string from the user and update it in the str string. Then to find the rarest character in the string, Call the getLowFreqChar() function with the input string ( str).
rarech = getLowFreqChar(str);
The getLowFreqChar() function will calculate the least frequent character in the str and returns it. We are storing the return value in the rarech variable.
Print the rarech character on the console.
Program Output:
Compile and Run the Program
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc low-freq-func.c $ ./a.out Enter a string : AA BBB CCCC DDDDD Frequency of Characters in given string : Character : ' ' - Frequency: 3 Character : 'A' - Frequency: 2 Character : 'B' - Frequency: 3 Character : 'C' - Frequency: 4 Character : 'D' - Frequency: 5 Character 'A' is the Low Frequency Character in the given string $ |
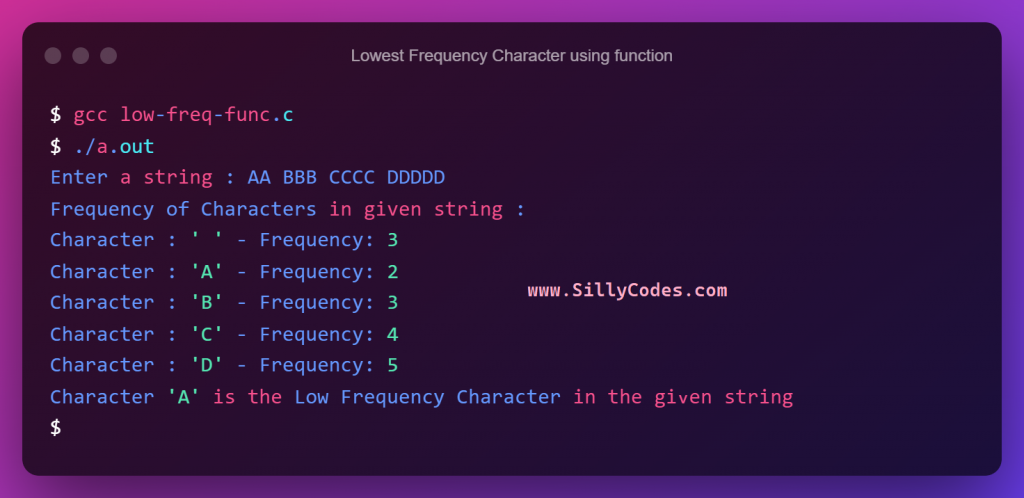
Here are a few examples of the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ ./a.out Enter a string : learn programming Frequency of Characters in given string : Character : ' ' - Frequency: 1 Character : 'a' - Frequency: 2 Character : 'e' - Frequency: 1 Character : 'g' - Frequency: 2 Character : 'i' - Frequency: 1 Character : 'l' - Frequency: 1 Character : 'm' - Frequency: 2 Character : 'n' - Frequency: 2 Character : 'o' - Frequency: 1 Character : 'p' - Frequency: 1 Character : 'r' - Frequency: 3 Character ' ' is the Low Frequency Character in the given string $ ./a.out Enter a string : CProgramming Frequency of Characters in given string : Character : 'C' - Frequency: 1 Character : 'P' - Frequency: 1 Character : 'a' - Frequency: 1 Character : 'g' - Frequency: 2 Character : 'i' - Frequency: 1 Character : 'm' - Frequency: 2 Character : 'n' - Frequency: 1 Character : 'o' - Frequency: 1 Character : 'r' - Frequency: 2 Character 'C' is the Low Frequency Character in the given string $ |
As we can see from the above input strings and program output, The program is generating the desired results and finding the rarest character in the string properly.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Compare Two Strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
2 Responses
[…] C Program to Find Lowest Frequency Character in a String […]
[…] C Program to Find Lowest Frequency Character in a String […]