Program to Check Identity Matrix in C Language
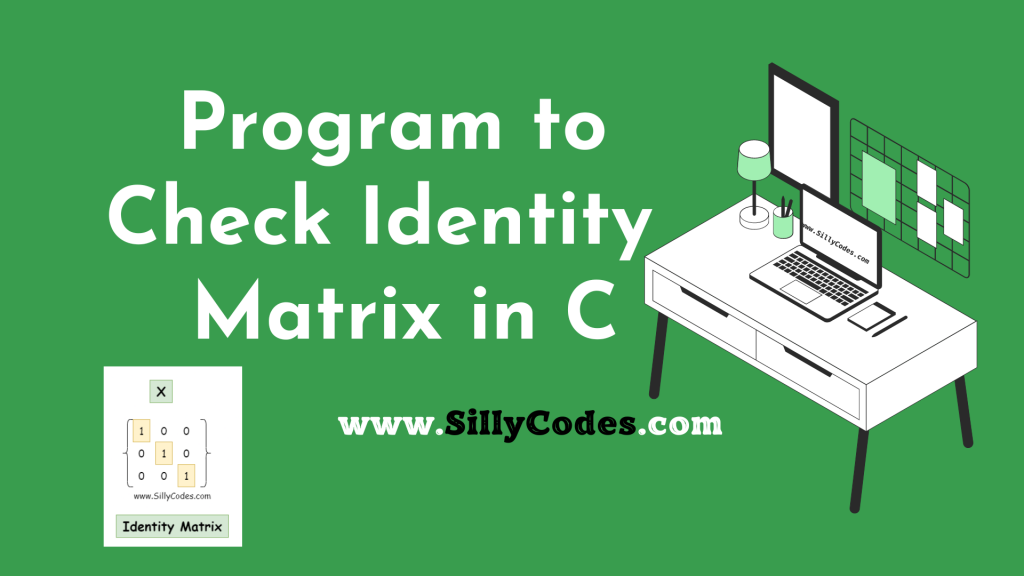
Program Description:
Write a Program to Check Identity Matrix in C programming. The program will accept a Matrix from the user and check if it is an Identity Matrix.
Prerequisites:
What is an Identity Matrix?
The Identity Matrix is a Square Matrix ( n x n) and the main diagonal elements of an Identity matrix are equal to 1 and the remaining elements are equal to Zero(0).
Here is an example of an Identity Matrix
1 2 3 |
1 0 0 0 1 0 0 0 1 |
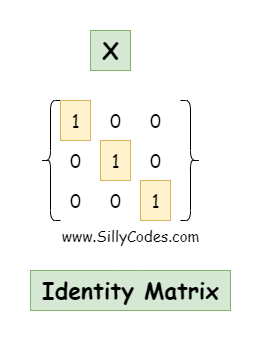
If you look at the above Identity Matrix, you will notice that all of the main diagonal elements have a value of 1 and the remaining elements have a value of 0.
The Identity Matrix is also called the Unit Matrix.
Program Excepted Input and Output:
Input:
1 2 3 4 5 6 |
Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 0 0 0 1 0 0 0 1 |
Output:
1 |
Given Matrix(X) is an Identity Matrix |
📢 This Program is Part of the Matrices Programs in C
- C Program to Read and Print Matrix or 2D Array
- C Program to demonstrate How to Pass 2D Array to Functions
- C Program to Multiplication of two Matrices
- C Program to Add Two Matrices
- C Program to Subtract Two Matrices
- C Program to Calculate the Transpose of Matrix
- C Program to Check Two Matrices are Equal
- C Program to Check Multiplicability of Two Matrices
- C Program to Check a Sparse Matrix
Check Identity Matrix in C Program Explanation:
Here is the step-by-step instructions for the Program to Check Identity Matrix in C
- Create two integer constants named ROWS and COLUMNS, Which holds the max number of rows and columns in the Matrix. (Change these values as per your requirement)
- Start the Program by declaring a Matrix (2D Arrays) called Matrix X. The row size and column size of the matrix is ROWS and COLUMNS constants respectively.
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively.
- An Identity Matrix is a Square Matrix, So the number of rows must be equal to columns. So check if the given matrix is a square matrix. ( Use (rows != columns))
- Check for array bounds using the (rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Input Matrix i.e Matrix-X, We need to use two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (that is for(i=0; i<rows; i++) ). At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). At each iteration of the Inner Loop
- Prompt the user to provide the Matrix element and Read the element using the scanf function and Update the X[i][j] element.
- Repeat the above step for all elements in the Matrix-X
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). At each iteration of the Inner Loop
- To check if the given Matrix is an Identity Matrix, We need to go through all elements of the Matrix and check all if all diagonal elements are equal to One and other elements are equal to Zero.
- Create Outer For Loop ( Iterates over
rows) – for(i=0; i<rows; i++)
- Then Create the Inner For loop (Iterates over
columns) – for(j=0; j<columns; j++)
- Check if the diagonal elements are equal to One using ( (i==j) && (X[i][j] != 1) ) condition, If any element is not equal to One(1), Then Matrix is not an Identity matrix.
- Similarly, Check if all other elements are equal to Zero using ( (i!=j) && (X[i][j] != 0) ) condition.If any element is not equal to Zero(0), Then Matrix is not an Identity matrix.
- Then Create the Inner For loop (Iterates over
columns) – for(j=0; j<columns; j++)
- If the step-9 is completed, Then the Matrix is an Identity Matrix. Print the result on the console.
Program to Check Identity Matrix in C Language:
Let’s convert the above algorithm into the C Code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
/* Program to Check if the Matrix is Identity Matrix in C SillyCodes.com */ #include <stdio.h> // Define MAX ROWS and COLUMNS const int ROWS=100; const int COLUMNS=100; int main() { // Declare two matrices. // 'X' is Input matrice int X[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // Check if the matrix is square if(rows != columns) { printf("Indentity Matrix is Square Matrix, Rows and Columns Must be Equal \n"); return 0; } else if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { // Matrix is out of bounds printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } // Check if the Matrix is Identity Matrix for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // main diagonal elements must be equal to 1 if( (i==j) && (X[i][j] != 1) ) { printf("Given Matrix(X) is Not an Identity Matrix\n"); return 0; } else if ( (i!=j) && (X[i][j] != 0) ) { // Others must be 0, Otherwise Not an Identity Matrix printf("Given Matrix(X) is Not an Identity Matrix\n"); return 0; } } } // Matrix is an Indentity Matrix printf("Given Matrix(X) is an Identity Matrix\n"); return 0; } |
Program Output:
Compile and Run the Program.
Test 1: Normal Cases:
1 2 3 4 5 6 7 8 9 10 |
$ gcc check-identity-matrix.c $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 0 0 0 1 0 0 0 1 Given Matrix(X) is an Identity Matrix $ |
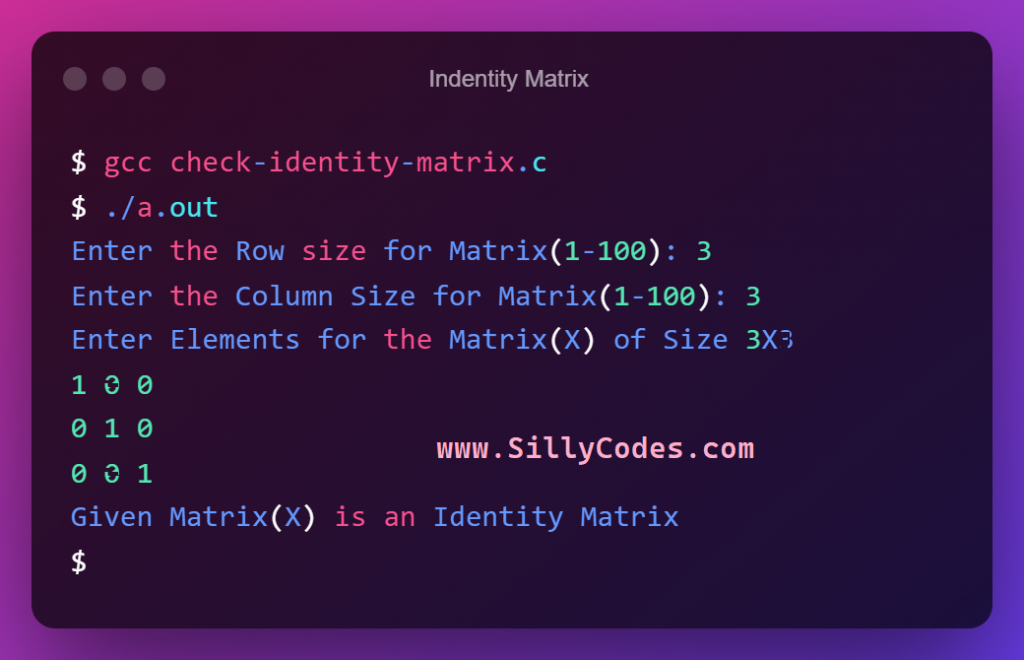
The above matrix X is an Identity Matrix. All diagonal elements are equal to One and the remaining elements are equal to Zero.
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 0 0 1 1 0 0 1 1 Given Matrix(X) is Not an Identity Matrix $ |
Here diagonal elements are one but one of the other elements ( X[2][1]) also has a value as One, So it is not an Identity Matrix.
Test 2: Negative Test Cases:
When a user enters a rectangular matrix.
1 2 3 4 5 |
$ ./a.out Enter the Row size for Matrix(1-100): 4 Enter the Column Size for Matrix(1-100): 3 Indentity Matrix is Square Matrix, Rows and Columns Must be Equal $ |
The Identity Matrix is a Square Matrix, So the row size and column size should match, Otherwise, program displays an error message Indentity Matrix is Square Matrix, Rows and Columns Must be Equal
Test 3: Out of bounds
1 2 3 4 5 |
$ ./a.out Enter the Row size for Matrix(1-100): 5000 Enter the Column Size for Matrix(1-100): 5000 Invalid Rows or Columns size, Please Try again $ |
As we can see from the above output, The given row and column sizes are out of present limits. So program displayed an error message Invalid Rows or Columns size, Please Try again
Program to Check Identity Matrix using Functions in C:
We used only one function (i.e main() function) to take the user input and check the identity matrix. It is recommended to use sub-functions for each specific task. As the function improves readability and code debuggability. Here are the advantages of using functions in the program
Let’s rewrite the above program to use functions for each specific task.
Here is the rewritten version of the Check Identity Matrix program in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 |
/* Program to Check if the Matrix is Identity Matrix in C using Functions SillyCodes.com */ #include <stdio.h> // Define MAX ROWS and COLUMNS const int ROWS=100; const int COLUMNS=100; // Function definition. int isIdentityMatrix(int rows, int columns, int X[rows][columns]); /** * @brief - Read Matrix from the user and update 'X' * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'X' * @param X - 'X' is Matrix or 2D array */ void readMatrix(int rows, int columns, int X[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { //printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } } int main() { // Declare two matrices. // 'X' is Input matrice int X[ROWS][COLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // Check if the matrix is square if(rows != columns) { printf("Indentity Matrix is Square Matrix, Rows and Columns Must be Equal \n"); return 0; } else if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { // Matrix is out of bounds printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, X); // call the 'isIdentityMatrix' to check if the matrix is Identity Matrix if(isIdentityMatrix(rows, columns, X)) { // Matrix is an Indentity Matrix printf("Given Matrix(X) is an Identity Matrix\n"); } else { // Not an Indentity Matrix printf("Given Matrix(X) is Not an Identity Matrix\n"); } return 0; } /** * @brief - check if the matrix 'X' is Identity Matrix * * @param rows - Matrix Rows * @param columns - Matrix Columns * @param X - Matrix 'X' * @return int - 1 if the 'X' is an Identity Matrix, Otherwise 0 */ int isIdentityMatrix(int rows, int columns, int X[rows][columns]) { int i, j; // Check if the Matrix is Identity Matrix for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // main diagonal elements must be equal to 1 if( (i==j) && (X[i][j] != 1) ) { return 0; } else if ( (i!=j) && (X[i][j] != 0) ) { // Others must be 0, Otherwise Not an Identity Matrix return 0; } } } // If we reach here, Then Matrix is Identity Matrix return 1; } |
We have defined two custom functions in the above program(except main() function)
- The
readMatrix() function – Used to read the elements from the user and update the Matrix
X
- Function Prototype – void readMatrix(int rows, int columns, int X[rows][columns])
- The readMatrix() function takes three formal arguments which are rows, columns, and Matrix X.
- This function uses Two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &X[i][j]);
- The readMatrix() function doesn’t return anything back to the caller. So return type is void.
- As the arrays are passed by the address/reference, All the changes made to the Matrix-X in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
isIdentityMatrix() function is used to check if the given matrix is an Identity matrix.
- Function Prototype : int isIdentityMatrix(int rows, int columns, int X[rows][columns])
- The isIdentityMatrix() function takes three formal arguments similar to above readMatrix() function, Arguments include rows, columns, and matrix X.
- This function checks the all elements in the Matrix-X and returns One(1) if the Matrix-X is an Identity Matrix, Otherwise, it returns Zero(0)
Call the isIdentityMatrix() function from the main() function and display the output based on the return value of the function.
Program Output:
Let’s compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
$ gcc check-identity-matrix-func.c $ ./a.out Enter the Row size for Matrix(1-100): 5 Enter the Column Size for Matrix(1-100): 5 Enter Elements for the Matrix(X) of Size 5X5: 1 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 1 0 0 0 0 0 1 Given Matrix(X) is an Identity Matrix $ $ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 2 3 4 1 5 0 0 1 Given Matrix(X) is Not an Identity Matrix $ |
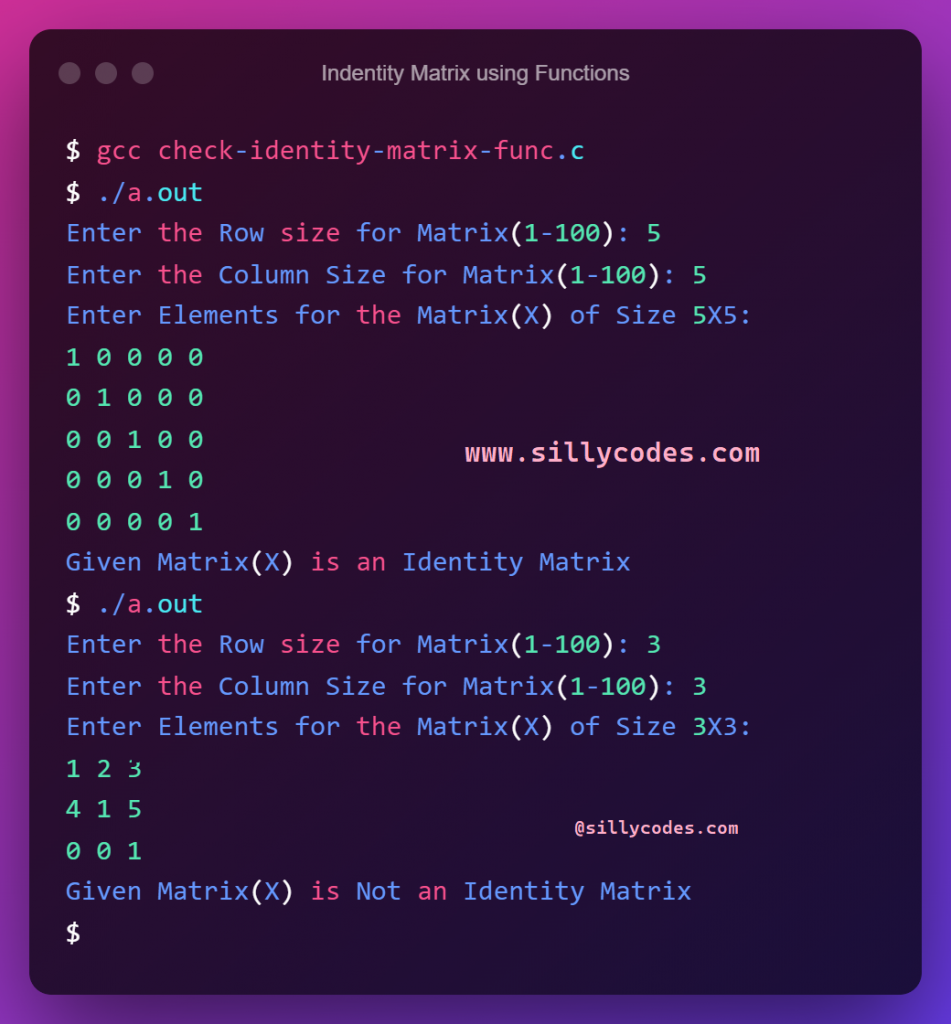
Let’s try a few more examples (including negative cases)
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 5 Enter the Column Size for Matrix(1-100): 4 Indentity Matrix is Square Matrix, Rows and Columns Must be Equal $ ./a.out Enter the Row size for Matrix(1-100): 6000 Enter the Column Size for Matrix(1-100): 6000 Invalid Rows or Columns size, Please Try again $ |
The program is generating the excepted output.
Related Array Programs:
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
- Linear Search in C Language with Example Programs
- C Program to Count Frequencies of Each Element in the Array
- C Program to Search element in an Array