Program to Remove Leading Whitespace in String in C Language
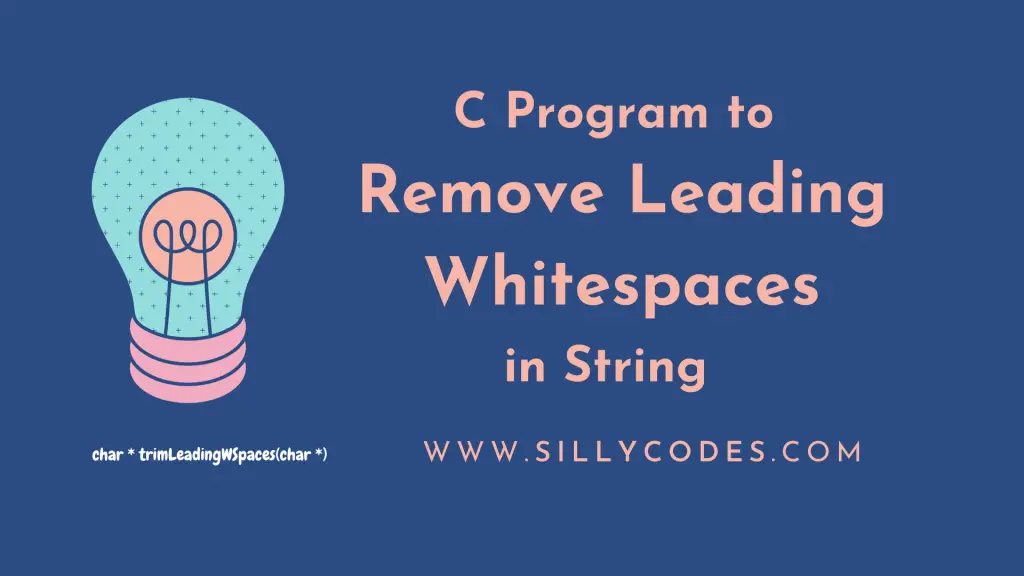
Program Description:
Write a Program to Remove Leading Whitespace in C programming language. The program should accept a string from the user and Trim the Leading whitespace or Remove the Leading whitespace from the input string. Let’s look at the example input and output of the program.
📢 This Program is part of the C String Practice Programs series
Example Input and Output:
Input:
Enter a string : Lot of Leading spaces
Output:
String after removing Leading Whitespaces:Lot of Leading spaces
The input string contains a few whitespace characters at the beginning, but the program removed all leading whitespaces, So the output string contains no leading white spaces.
Prerequisites:
We are going to use the C-Strings and Functions, So please go through the following articles to learn more about these topics.
- Strings in C Language with Example Programs
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
Let’s look at the step-by-step explanation of the program to trim leading white spaces in C.
Remove Leading Whitespaces in String in C Program Explanation:
- Create an integer constant named SIZE with the value 100. This is the max size of the character array. ( Change this value if you want to use large strings)
- Create a character array i.e string to store the user input. Let’s say we created a inputStr string with size of SIZE elements.
- Take the input string( inputStr) from the user and Read it using the gets function. We can also use other functions like scanf, fgets, etc. Also, note that the gets function doesn’t do the boundary checks.
- Create variable called start and initialize it with Zero(0). This variable holds the index of the first non-whitespace character in the inputStr
- Create a While Loop and Iterate over the string from the
start and check if the character at the
start index is a white space. We use isspace() function from the
ctype.h header file.
- If the character at the index start is white space, Then increment the start by 1 – start++
- repeat this step till we find the first non-whitespace character in the string.
- Once Step 5 is completed, The start index will be pointing to the first non-whitespace character in the string.
- To Remove the Leading whitespace from the string, Shift the valid characters(non-whitespace characters) to the start(Zeroth Index) of the string.
- Create a For loop and Shift the character’s(non-whitespace) start of the string. –
for(i = 0; inputStr[i]; i++)
- At each iteration, Replace the element at the index i with the character at the index i+start – i.e inputStr[i] = inputStr[i+start];
- Finally, Add the terminating NULL Character ( '\0') to the inputStr – inputStr[i+1] = '\0';
- Print the Resultant string on Console.
Program to Remove Leading Whitespace in C Language:
Here is the Program to Remove Leading Whitespace in C using Iterative method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/* program to Remove leading white spaces in a String in C sillycodes.com */ #include<stdio.h> #include<ctype.h> // Max Size of string const int SIZE = 100; int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter a string : "); gets(inputStr); // Create a variable 'start' and assign 'Zero' int start = 0; // Create a loop and check for leading white spaces while(isspace((unsigned char)inputStr[start])) { // found leading whitespace // increment 'start' start++; } // If there are any leading white spaces, Shift the valid characters to start of the string(Zeroth index). if(start != 0) { // Shift the characters to start of the string. for(i = 0; inputStr[i]; i++) { // Replace element at index 'i' with 'i+start' inputStr[i] = inputStr[i+start]; } // add terminating NULL Character inputStr[i+1] = '\0'; } // Print Results printf("String after removing Leading Whitespaces:%s\n", inputStr); return 0; } |
Program Output:
Compile and Run the program using your favorite IDE. We are using the GCC compiler in this example.
1 2 3 4 5 |
$ gcc leading-trim.c $ ./a.out Enter a string : Lot of Leading spaces String after removing Leading Whitespaces:Lot of Leading spaces $ |
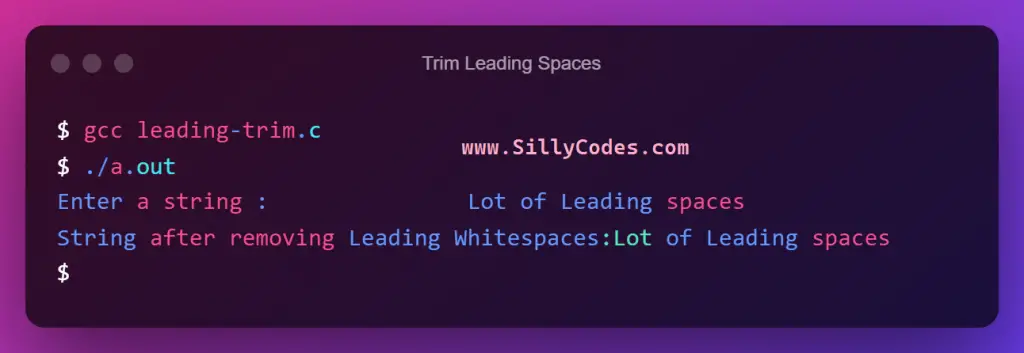
Let’s try with the Tabs
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a string : With Tabs String after removing Leading Whitespaces:With Tabs $ $ ./a.out Enter a string : Learn Programming String after removing Leading Whitespaces:Learn Programming $ |
As we can see from the above user input strings and Output strings, The program is able to remove the leading whitespaces successfully.
Remove Leading Whitespace in String in C using a user-defined function:
We used only a single function to do all the tasks in the above program. Let’s divide the above program and create a function to remove the leading whitespaces in the given string.
The function should accept a string from the caller and remove the leading whitespaces and return the resultant string back to the caller.
Here is the modified version of the above program, Where we created a user-defined function named trimLeadingWSpaces to trim or Remove the leading whitespace in the input string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/* program to Remove leading white spaces in a String in C using user-defined functions. sillycodes.com */ #include<stdio.h> #include<ctype.h> // Max Size of string const int SIZE = 100; /** * @brief - Trim / Remove Leading white spaces in the given string. * * @param str - Input String * @return char* - Returns resultant string */ char * trimLeadingWSpaces(char * str) { // Create a variable 'start' and assign 'Zero' int start = 0; int i; // Create a loop and check for leading white spaces while(isspace((unsigned char)str[start])) { // found leading whitespace // increment 'start' start++; } // If there are any leading white spaces, Shift the valid characters to start of the string(Zeroth index). if(start != 0) { // Shift the characters to start of the string. for(i = 0; str[i]; i++) { // Replace element at index 'i' with 'i+start' str[i] = str[i+start]; } // add terminating NULL Character str[i+1] = '\0'; } return str; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter a string : "); gets(inputStr); // call the 'trimLeadingWSpaces' with 'inputStr' to remove the the leading spaces trimLeadingWSpaces(inputStr); // Print Results printf("String after removing Leading Whitespaces:%s\n", inputStr); return 0; } |
We have defined the trimLeadingWSpaces() function in the above program.
- Prototype of the Function : char * trimLeadingWSpaces(char * str)
- This function takes a Formal Argument i.e the input string (str) and removes all leading characters from the str and returns the resultant string str back to the caller.
In the main() function, we called the trimLeadingWSpaces(inputStr) with the inputStr as the actual argument.
Program Output:
let’s compile and Run the program.
1 2 3 4 5 6 7 8 9 |
$ gcc leading-trim-fun.c $ ./a.out Enter a string : Nothing is Permanent String after removing Leading Whitespaces:Nothing is Permanent $ $ ./a.out Enter a string : learn programming at sillycodes.com String after removing Leading Whitespaces:learn programming at sillycodes.com $ |
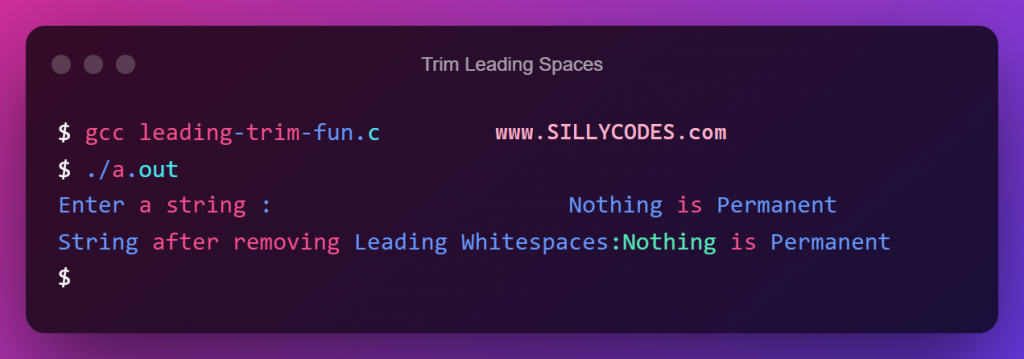
The program is providing the desired results.
Related String practice programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
2 Responses
[…] C Program to Remove Leading Whitespaces in a String […]
[…] C Program to Remove Leading Whitespaces in a String […]