Program to Reverse print array in C language
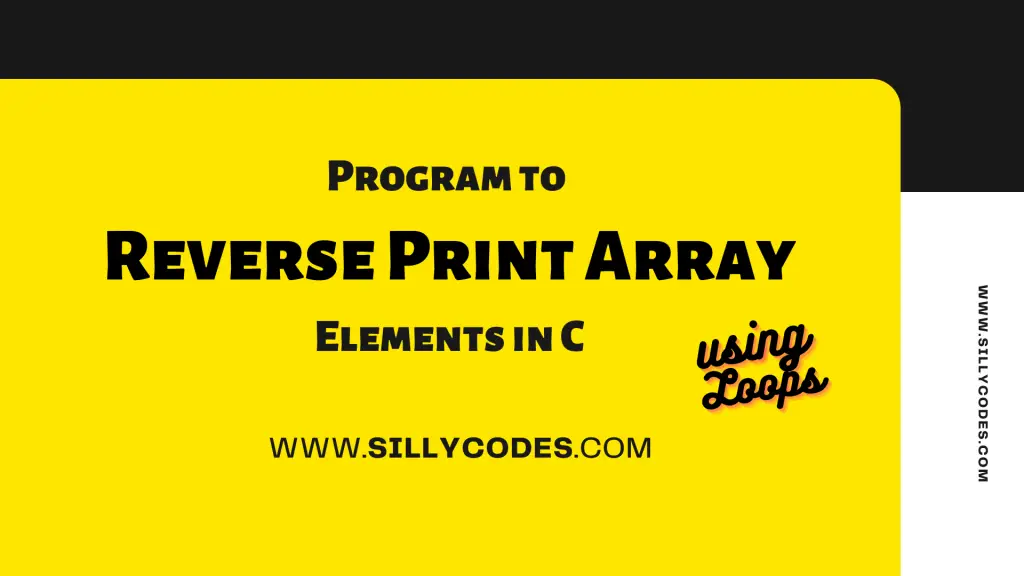
Program Description:
Write a C Program to Reverse print array in C programming language. Please note we are not reversing the array, We are just printing it in reverse order. The program will accept all elements in the array from the user and print them in reverse order.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Please provide the input data[0] : 0 data[1] : 1 data[2] : 2 data[3] : 3 data[4] : 4 data[5] : 5 data[6] : 6 data[7] : 7 data[8] : 8 data[9] : 9 |
Output:
1 2 |
Array (Original) : 0 1 2 3 4 5 6 7 8 9 Array (Reverse)Â Â : 9 8 7 6 5 4 3 2 1 0 |
Prerequisites:
It is recommended to go through the following articles to better understand the reverse array program
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
Reverse print array in C Program Explanation:
- Create a global constant named SIZE and initialize it with 10. (we can also use the macro)
- Declare a array named data with the size of SIZE variable. i.e 10.
- Let’s utilize a for loop to capture user input and store it in array elements. . To do that we need to loop through the array elements and update each array element using the scanf function – scanf("%d", &data[i]);
- Use another for loop to print the array in given order.
- To print the array in reverse order, Start the loop from the
SIZE-1(i=SIZE-1) and decrement the
i until it reaches
zero(0). – for(i=SIZE-1; i>=0; i–)
- At each iteration, Print the value of the data[i]
Reverse Print Array Program Algorithm:
- Declare an integer array data of size SIZE and an integer variable i
- Prompt the user to input the values for the array
- FOR
i = 0 to
SIZE-1 ( Loop )
- Read the value for arr[i]
- FOR
i = 0 to
SIZE-1 ( Loop )
- Print the value of arr[i]
- FOR
i = SIZE-1 to
Zero(0) ( Loop )
- Print the value of arr[i]
Reverse print array in C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program to Reverse print the array     Author: SillyCodes.com */ #include <stdio.h> const int SIZE = 10; int main() {     int i;     // declare an array 'data'     int data[SIZE];      printf("Please provide the input\n");     // Read the input from the user     for(i=0; i<SIZE; i++)     {         printf("data[%d] : ", i);         scanf("%d", &data[i]);     }      // Print the original array     printf("Array (Original) : ");     for(i=0; i<SIZE; i++)     {         printf("%d ", data[i]);     }     printf("\n");      // Reverse print Array Elements     printf("Array (Reverse)  : ");     for(i=SIZE-1; i>=0; i--)     {         printf("%d ", data[i]);     }     printf("\n");      return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ gcc reverse-print-array.c $ ./a.out Please provide the input data[0] : 0 data[1] : 1 data[2] : 2 data[3] : 3 data[4] : 4 data[5] : 5 data[6] : 6 data[7] : 7 data[8] : 8 data[9] : 9 Array (Original) : 0 1 2 3 4 5 6 7 8 9 Array (Reverse)Â Â : 9 8 7 6 5 4 3 2 1 0 $ |
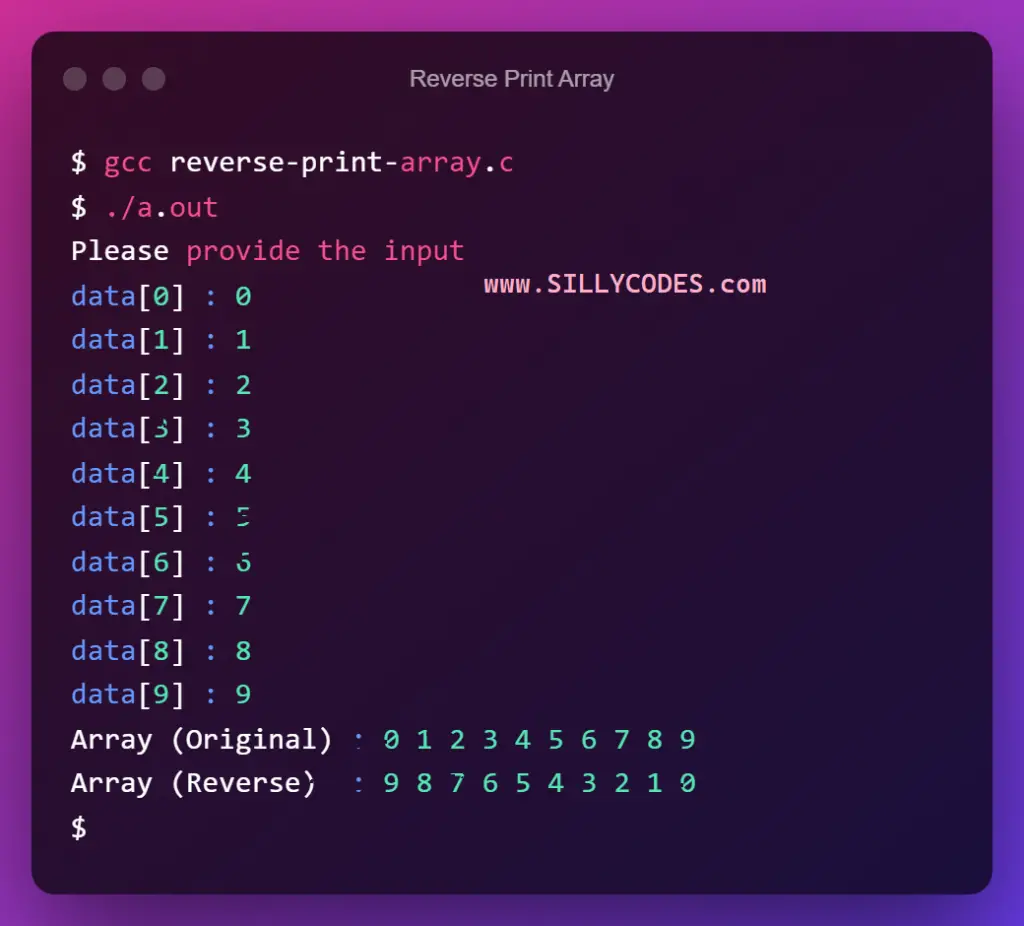
Reverse Print using Array using functions in C:
In the above program, We have written all our code in the main() function. Let’s use the functions to read and print the array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 |
/*     Program to Reverse print the array     Author: SillyCodes.com */ #include <stdio.h> const int SIZE = 5;  /** * @brief  - Read the elements from INPUT * * @param data - 'data' Array * @param size - size of the array */ void read(int *data, int size) {     int i;     printf("Please provide the Array elements\n");     // Read the input from the user     for(i=0; i<size; i++)     {         printf("data[%d] : ", i);         scanf("%d", &data[i]);     } }  /** * @brief  - Print the array elements * * @param data - 'data' array * @param size - size of the 'data' array */ void print(int *data, int size) {     int i;     for(i = 0; i<size; i++)     {         printf("%d ", data[i]);     }     printf("\n"); }  /** * @brief  - Reverse Print the array elements * * @param data  - 'data' array * @param size  - Size of the 'array' */ void reversePrint(int *data, int size) {     int i;     for(i=size-1; i>=0; i--)     {         printf("%d ", data[i]);     }     printf("\n"); }  int main() {     int i;     // declare an array 'data'     int data[SIZE];      // Take the input from the user     read(data, SIZE);      // Print the original array     printf("Array (Original) : ");     print(data, SIZE);      // Reverse print Array Elements     printf("Array (Reverse)  : ");     reversePrint(data, SIZE);      return 0; } |
Reverse print array using Program Explanation:
- Create a global variable SIZE and assign the integer 5.
- Create a
read function to read input numbers from the user. The
read() function takes an integer pointer
data and its
size as formal arguments –
void read(int *data, int size)
- The read function uses a for loop to prompt the user for input and updates the array elements.
- Define
print function to print the array elements in given order. The
print function takes
*data and
size as formal arguments –
void print(int *data, int size)
- Iterate over each array element using for Loop and print it using the data[i]
- Define another function
reversePrint to print the array in reverse order. This function also takes an integer array and it’s size as arguments.
- The reversePrint function iterates over the array in reverse order. Starts from SIZE-1 and comes to zero(0).
- At each iteration, Print the data[i] element.
- Call the read(), print(), and reversePrint() functions from the main() function.
Program Output:
Compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc reverse-print-array.c $ ./a.out Please provide the Array elements data[0] : 5 data[1] : 4 data[2] : 3 data[3] : 2 data[4] : 1 Array (Original) : 5 4 3 2 1 Array (Reverse)Â Â : 1 2 3 4 5 $ |
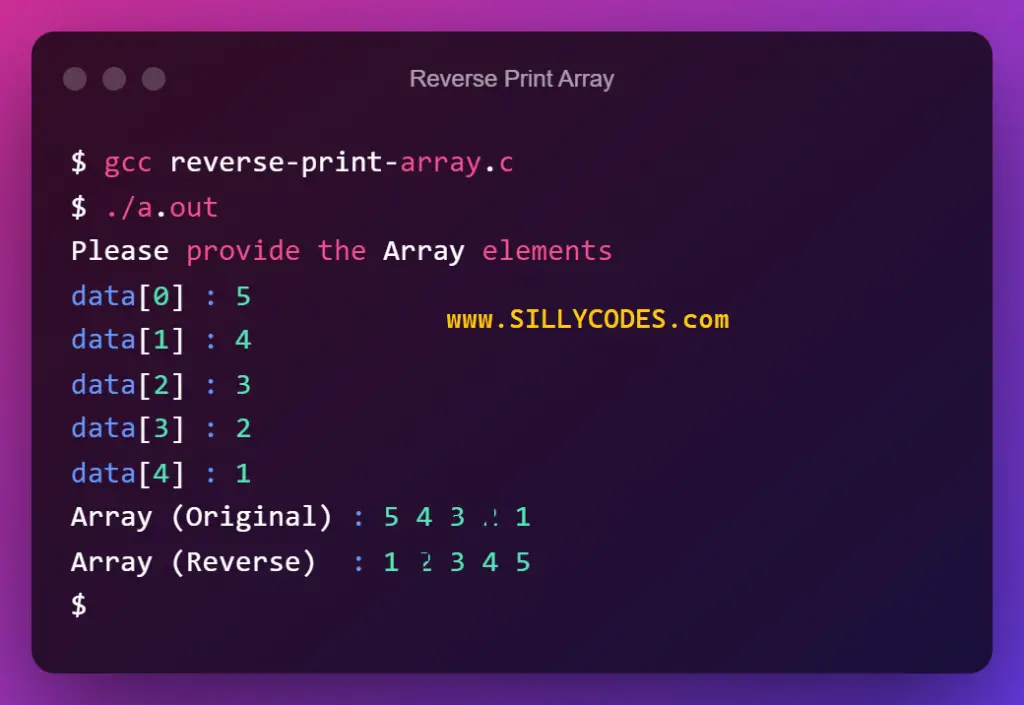
As we can see from the above output, We are getting the expected output.
Related Programs:
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
- C Program to Generate Nth Armstrong Number
- C Program to Generate Multiplication Tables
- Star and Number Pattern Programs [ Triangles, Pyramids, Rhombus, etc ]
1 Response
[…] Reverse Print Array Program in C […]