Even or Odd number in C using function
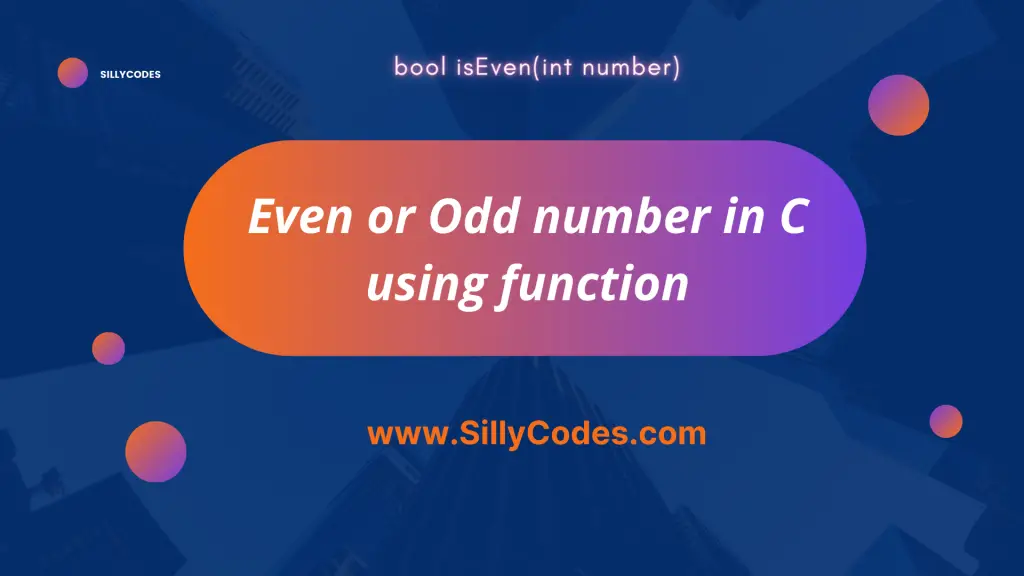
Even or Odd number in C using function Program Description:
Write a Program to check whether the given number is an Even or Odd number in C using function. The program should accept a number from the user and detect if it is an even number or an odd number.
We can create a function called isEven (or isOdd), Which detects if the given number is an even number or an odd number.
Excepted Input and Output:
Input:
Enter a Number : 30
Output:
30 is a Even Number
Pre-Requisites:
Even or Odd number in C using function Algorithm:
- Take the input from the user and store it in a variable called number
- Create a function called
isEven, Which takes the
number as the input and check if the
numberis Even number.
- The isEven function returns boolean
- If the number is an Even number, The isEven function returns true, Otherwise it will return False.
- Call the isEven function from the main function and pass the number as the input.
- Check the return value of
isEven and print the result accordingly.
- If the return value is true, Then number is an Even Number.
- If the return value is false, Then number is an Odd Number.
- Stop the program.
Program to check Even or Odd Number in C using Function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
/*     Program to check Even or Odd number using function */ #include<stdio.h> #include<stdbool.h>  /** * @brief Check if the 'number' is even number * * @param number * @return true if 'number' is even * @return false if 'number' is Odd */ bool isEven(int number) {     // check if the 'number' is even     return ((number % 2) == 0); }  int main() {     int number;     printf("Enter a Number : ");     scanf("%d", &number);      // call the isEven() function     if(isEven(number))     {         printf("%d is a Even Number\n", number);     }     else     {         printf("%d is an Odd Number\n", number);     }      return 0; } |
Prototype details of the isEven function.
- <strong>bool</strong> <strong>isEven</strong>(int number)
- function_name : isEven
- arguments_list: int
- return_type : bool
Program Output:
Compile the program.
$ gcc even-odd.c
Run the executable file.
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number : 30 30 is a Even Number $ ./a.out Enter a Number : 15 15 is an Odd Number $ ./a.out Enter a Number : 1000 1000 is a Even Number $ |
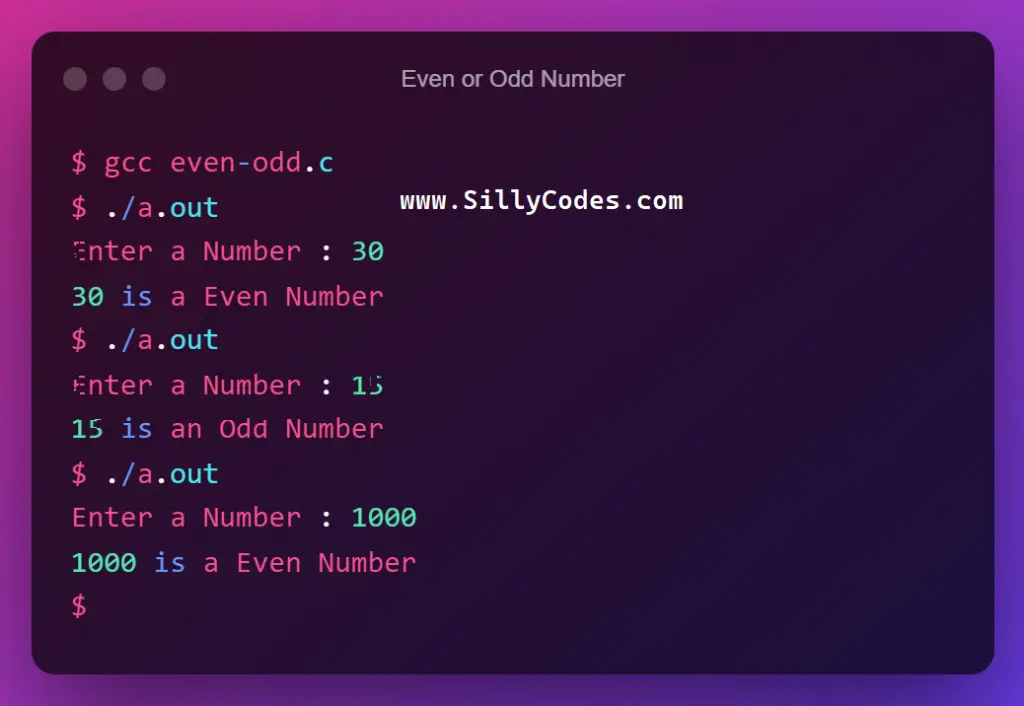
As we can see from the above output, We are getting the desired results.
Related Programs:
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of numberÂ
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
- C Program to Generate Nth Armstrong Number
- C Program to Generate Multiplication Tables
- Star and Number Pattern Programs [ Triangles, Pyramids, Rhombus, etc ]
2 Responses
[…] Program to Check Even or Odd number using Function […]
[…] two variables to track the count of the total number of even numbers and odd numbers. So Initialize the nEven and nOdd variables with […]