Find all Occurrences of Character in String in C Language
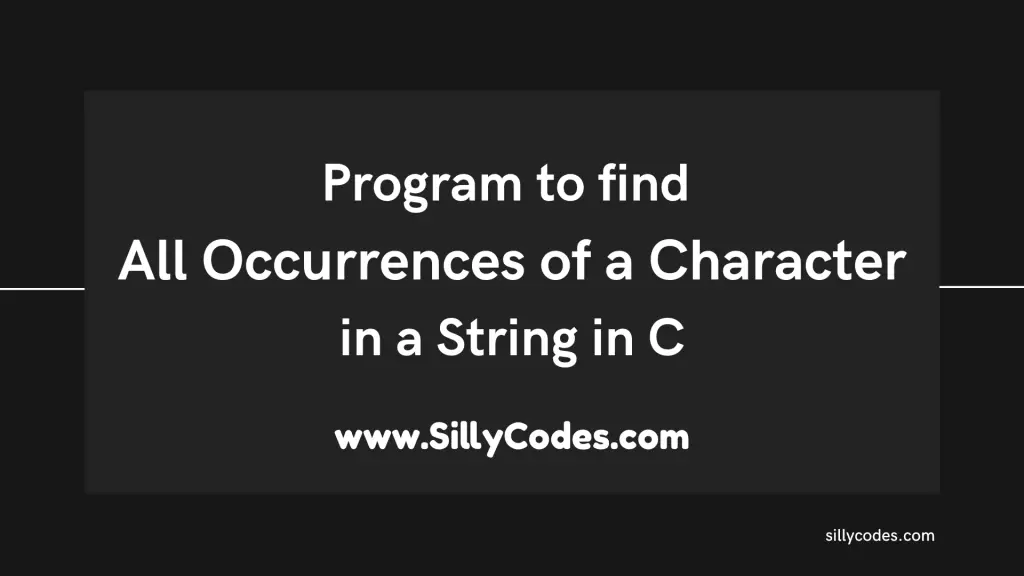
Program Description:
Write a Program to Find All Occurrences of a Character in String in C Programming language. The program should accept a string and a character from the user and find all occurrences of the given character in the input string.
📢 This Program is part of the C String Practice Programs series
We will look at a few methods to search for all occurrences of a character in the string program. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
Example Input and Output:
Input:
Enter a string : May you live every day of your life
Enter character to search in the string: y
Output:
FOUND: Character 'y' is found at 2 index
FOUND: Character 'y' is found at 4 index
FOUND: Character 'y' is found at 17 index
FOUND: Character 'y' is found at 21 index
FOUND: Character 'y' is found at 26 index
The character y is found multiple times in the input string. The program displayed all occurrences of y with indices.
📢 The program is case-sensitive. So the Uppercase characters and Lowercase characters are different.
Prerequisites:
It is recommended to know the C-Strings, C-Arrays, and Functions in C to better understand the following program.
We will look at the three different methods to find all occurrences of a character in a string in C Language.
- Iterative Method
- User-defined Functions
- Library Function ( strchr library function)
Find All Occurrences of a Character in a String in C Program Explanation:
- Start the program by defining a Macro to hold the max size of the array – #define SIZE 100
- Declare the input string( inputStr)
- Take the input string and character to search from the user and update the inputStr and ch variables. We use the gets function to read the string.
- Initialize a variable named isFound with the -1. The isFound variable is used to check if the character( ch) is found in the string.
- To find all occurrences of the character( ch) in a string( inputStr), Iterate over the string and compare each character with the target character( ch)
- Create a For loop by initializing variable i with 0. It will continue looping as long as the current character (
inputStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( inputStr[i]) is equal to ch- i.e if(inputStr[i] == ch)
- If the above condition is true, print the FOUND message with the current character index( i). Also, update the isFound variable with the current index( i). i.e isFound = i;
- Otherwise, i.e If the condition is false, Then continue to the next iteration.
- Increment control variable i by 1.
- Once the loop is completed, Check if the variable isFound is updated. If it is updated, Then we found the ch in the inputStr and printed all occurrences of character. Otherwise, i.e if the isFound == -1, Then the character( ch) is not found in the string( inputStr). So display the NOT FOUND message on the console and terminated the program.
Program to Find all Occurrences of Character in String in C – Iterative Method:
Convert the above algorithm into the C Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
/*     program to Find all occurrences of a character in a string in c     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;     int i;      // variable to track if 'ch' is found     int isFound = -1;      // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // Iterate over the string     for(i = 0; str[i]; i++)     {         // check if the current character is equal to target('ch') character         if(str[i] == ch)         {             // Found the string.             printf("FOUND: Character '%c' is found at %d index\n", ch, i);             isFound = i;         }     }      // check if the 'isFound' is updated     if(isFound == -1)     {         // 'isFound' is not updated. So character not found.         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }      return 0; } |
Program Description:
Let’s compile and run the program.
Example 1:
1 2 3 4 5 6 7 8 9 10 |
$ gcc find-all-chars-string.c $ ./a.out Enter a string : May you live every day of your life Enter character to search in the string: y FOUND: Character 'y' is found at 2 index FOUND: Character 'y' is found at 4 index FOUND: Character 'y' is found at 17 index FOUND: Character 'y' is found at 21 index FOUND: Character 'y' is found at 26 index $ |
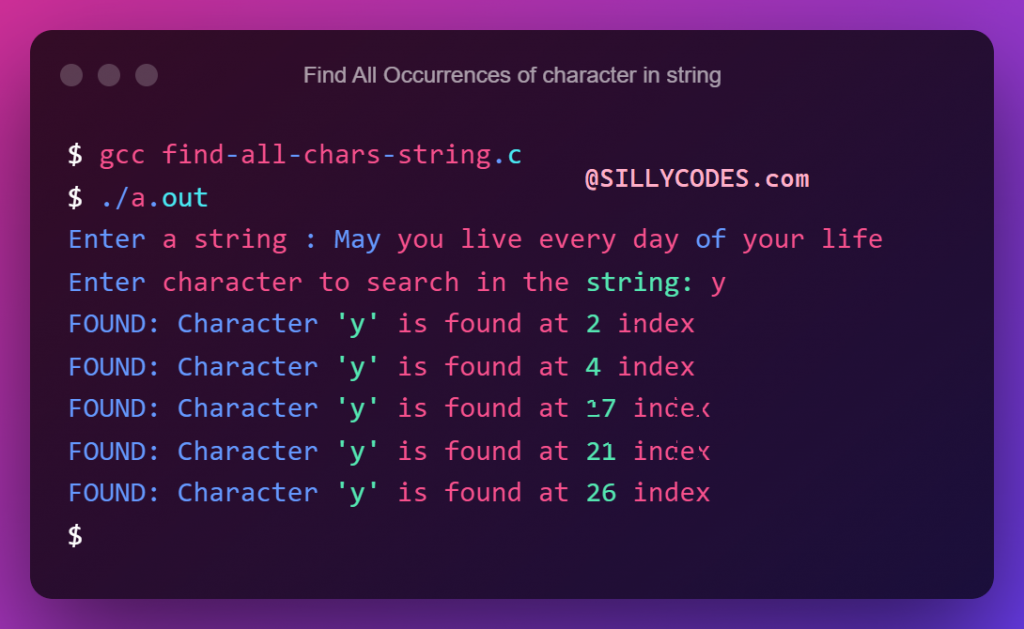
As we can see from the above example, The input string is May you live every day of your life and the character to be searched is y. The program is able to find all occurrences of the y in string and displayed it with indices.
Example 2:
1 2 3 4 5 |
$ ./a.out Enter a string : God Speed Enter character to search in the string: a NOT FOUND: Character 'a' is not found in the given string $ |
The character a is not found in the input string God Speed.
Find all Occurrences of Character in String using user-defined functions in C:
In the above program, We have written the complete program inside the main() function. It is advised to use functions to perform a specific task. Let’s rewrite the above program to use a function to print all occurrences of the character in a given string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the Advantages of functions in programming.
Here is the rewritten version of the program to print all occurrences of a character in a string using a user-defined function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 |
/*     program to Find all occurrences of a character in a string in c     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Find All Occurrences of character in a string and print the results * * @param str - input string * @param ch  - character to be searched in 'str' * @return int - return -1 if the character is not found, Otherwise returns the last index. */ int findAllOccurrences(char * str, char ch) {     int i, isFound = -1;      // Iterate over the string     for(i = 0; str[i]; i++)     {         // check if the current character is equal to target('ch') character         if(str[i] == ch)         {             // Found the string.             printf("FOUND: Character '%c' is found at %d index\n", ch, i);             isFound = i;         }     }      return isFound; }  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;     int i;      // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // call 'findAllOccurrences' function with 'str' and 'ch'     int result = findAllOccurrences(str, ch);      // if 'result' is -1, Then 'ch' is not found     if(result == -1)     {         // 'result' is not updated. So character not found.         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }      return 0; } |
We have defined a function called findAllOccurrences(). This function takes two formal arguments, They are the string( str) and character( ch). We need to search for all occurrences of the character( ch) in the string( str).
Here is the prototype of the findAllOccurrences() function.
int findAllOccurrences(char * str, char ch)
The findAllOccurrences() function also returns an integer back to the caller. If the character(ch) is found in the string(str), Then it will return an integer value greater than the Zero(0). to be precise it returns the character( ch)’s last index. If the character( ch) is not found then this function returns -1.
We use the same logic as the above to print all occurrences of the string.
In the main() function, Call the findAllOccurrences() function with the input string and the character.
1 2 |
// call 'findAllOccurrences' function with 'str' and 'ch' int result = findAllOccurrences(str, ch); |
Finally, Store the return value in result variable and print the notice on the screen based on the result variable.
Program Output:
Compile and Run the program using GCC compiler (Any compiler)
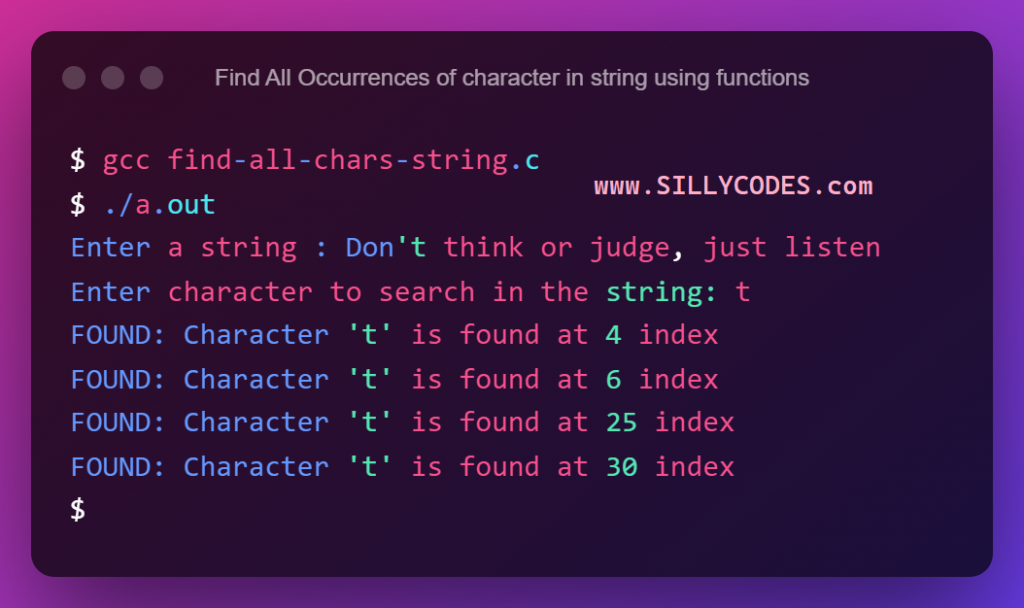
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc find-all-chars-string.c  $ ./a.out Enter a string : Don't think or judge, just listen Enter character to search in the string: t FOUND: Character 't' is found at 4 index FOUND: Character 't' is found at 6 index FOUND: Character 't' is found at 25 index FOUND: Character 't' is found at 30 index $ $ ./a.out Enter a string : energy Enter character to search in the string: x NOT FOUND: Character 'x' is not found in the given string $ |
As we can see from the above output, The program is properly printing all occurrences of the character.
Program to Find all Occurrences of Character in String using strrchr library function:
In this method, Let’s use the strchr library function to find all occurrences of the character in string in the C programming language.
Syntax of the strchr function.
1 |
char * strchr(const char *str, int ch); |
The strchr() function searches for the character ch in the input string str.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     program to Find all occurrences of a character in a string in c using strchr     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;     char *ptr;      // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // Store the 'str' in 'tempPtr'     char * tempPtr = str;      // Use 'strchr' with 'tempPtr' to find all occurences of 'ch'     while((tempPtr = strchr(tempPtr, ch)) != NULL)     {         printf("FOUND: Character '%c' is found at %ld index\n", ch, tempPtr-str);         tempPtr++;     }      return 0; } |
📢Make sure to include the string.h header file to use the strchr() function.
Initialize the tempPtr with the input string str. We use the tempPtr pointer to traverse the input string( str) and search for the character( ch).
As we have specified earlier, the strchr() function searches for the first occurrence of character ‘ch’ in the input string tempPtr i.e str. If the character ch is found, then the strchr() function returns a pointer to the first occurrence of ch in tempPtr. If ch is not found, then the function returns a NULL Pointer.
If the character is found, Then inside the while loop, we print the FOUND message and the loop will continue to run and search for more occurrences of a character ch in string tempPtr, until the strchr() function returns a NULL pointer. This means we found all occurrences of the character ch in the input string( tempPtr).
Program Output:
Compile and Run the program and observe the output.
1 2 3 4 5 6 7 8 9 10 |
$ gcc find-all-strchr.c $ ./a.out Enter a string : Go Go Go George boy Enter character to search in the string: o FOUND: Character 'o' is found at 1 index FOUND: Character 'o' is found at 4 index FOUND: Character 'o' is found at 7 index FOUND: Character 'o' is found at 11 index FOUND: Character 'o' is found at 17 index $ |
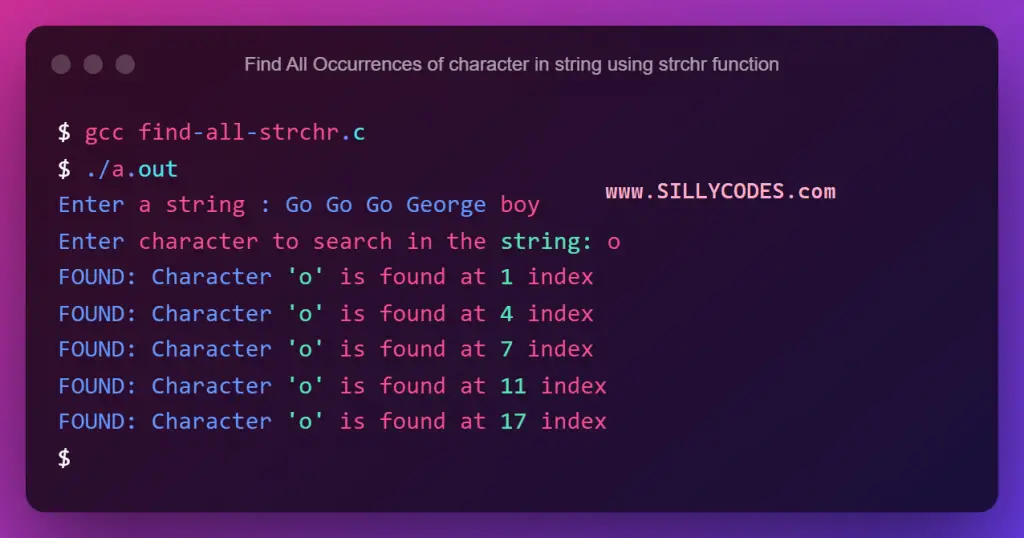
As we can see from the above output, The program is generating the desired results.
Related Programs:
- C Tutorials Index
- C Programs Index
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string