Matrix Scalar Multiplication in C Language
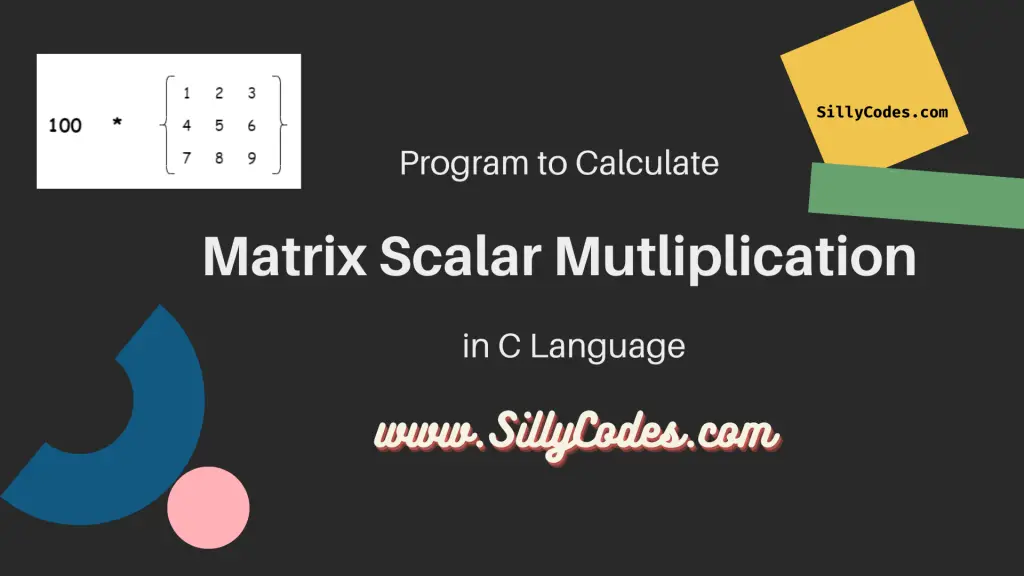
Program Description:
Write a Program to perform the Matrix Scalar Multiplication in C programming language. The program should accept a Matrix and a scaler value from the user and multiply the all elements of the matrix with the provided scaler number.
Here is the example input and output of the program.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 |
Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 2 3 4 5 6 7 8 9 Enter the Number to mutliply the matrix: 100 |
Output:
1 2 3 4 |
Resultant Maxtrix (Z = num * X) is : 100 200 300 400 500 600 700 800 900 |
Here is the Pictorial representation of the above Scaler and Matrix Multiplication Operation.
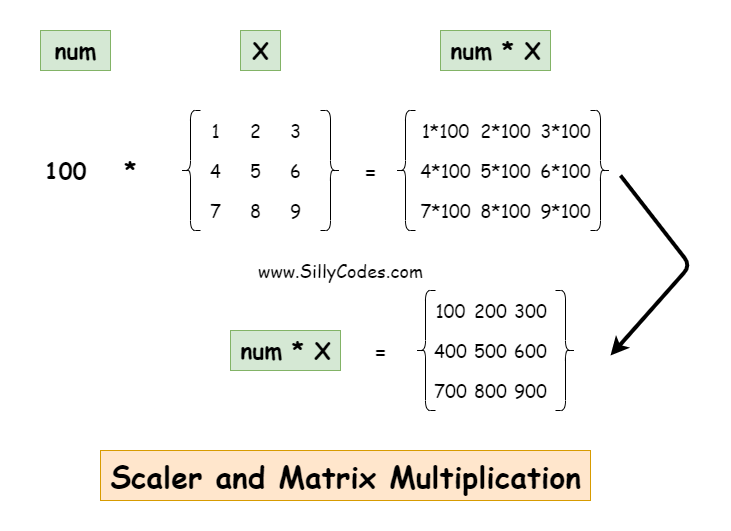
If you observe the above output, Each element in the input array has been multiplied by the scaler number ( 100).
Prerequisites:
It is recommended to know the basics of the Arrays and 2D Arrays and How to pass 2D arrays to function in order to better understand the following program
- C Arrays
- 2D Arrays in C
- Passing 2D Arrays to Functions
- Functions in C how to create and use functions
Matrix Scalar Multiplication in C Program Explanation:
Let’s look at the step-by-step instructions of the Matrix Scaler Multiplication program in C language.
- Create two integer constants called ROWS and COLUMNS with Value 100, The constant ROWS holds the max row size of the matrix and similarly, the COLUMNS constant holds the max size of the columns of the Matrix. ( Change this number if you want to use the large arrays)
- Declare two matrices
X[ROWS][COLUMNS], Z[ROWS][COLUMNS] with the max rows and columns. Also, declare the
num variable.
- The Matrix-X is the input matrix and The num is the scaler number. Finally the Matrix-Z stores the results of the Matrix and Scaler Multiplication – ( num * X)
- Prompt the user to provide the desired Row size and Column Size, and store them in the variables rows and columns respectively.
- Check for array bounds using the (rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) condition, And display an error message if the row/column sizeis beyond the present limits.
- Take the user input for Matrix-X, We need to create two For Loops, One For Loop will iterate over the rows, and the Second For Loop will iterate over the columns
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<rows (i.e for(i=0; i<rows; i++) ). At Each iteration of the Outer Loop,
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Prompt the user to provide the Matrix or 2D Array element and Read the element using the scanf function and Update the values[i][j] element.
- Repeat the above step for all elements in the 2D array or matrix
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<columns (i.e for(j=0; j<columns; j++) ). The Inner Loop will iterate over the columns. At each iteration of the Inner Loop
- Prompt the user to provide the value for the Scaler and store it in num variable.
- To Calculate the Scaler and Matrix Multiplication, We again need to use two nested For loops to iterate over the matrix elements.
- Create Outer For Loop ( iterates over rows) – for(i=0; i<rows; i++)
- Create Inner For loop (iterates over columns) – for(j=0; j<columns; j++)
- Multiply the scaler num with the array element X[i][j] and store the result in Z[i][j]
- which is equal to Z[i][j] = num * X[i][j];
- Create Inner For loop (iterates over columns) – for(j=0; j<columns; j++)
- Once the above step-9 is finished, The Matrix-Z contains the Resultant Matrix ( i.e Scaler and Matrix Multiplication)
- Display the results using by going through the Matrix-Z elements using another two for loops.
- Stop the program.
Program to perform Matrix Scalar Multiplication in C Language:
let’s write the C program to perform the Matrix and scaler multiplication.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/* Program to multiply scaler and Matrix in C SillyCodes.com */ #include <stdio.h> const int ROWS=100; const int COLUMNS=100; int main() { // Declare two matrices. // 'X' is Input matrice // 'Z' stores result of 'X' and scaler('num') multiplication int X[ROWS][COLUMNS], Z[ROWS][COLUMNS], num; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } printf("Enter the Number to mutliply the matrix: \n"); scanf("%d", &num); // Scaler Matrix multiplication num * X for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // muliply each elment with the 'num' Z[i][j] = num * X[i][j]; } } // Print the Result printf("Resultant Maxtrix (Z = num * X) is : \n"); for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", Z[i][j]); } printf("\n"); } return 0; } |
Program Output:
Compile and Run the Program using your favorite compiler. We are using GCC compiler.
$ gcc scaler-matrix-mul.c
Run the Program.
Test 1: Provide the Matrix elements and scaler value to the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ ./a.out Enter the Row size for Matrix(1-100): 3 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 3X3: 1 2 3 4 5 6 7 8 9 Enter the Number to mutliply the matrix: 100 Resultant Maxtrix (Z = num * X) is : 100 200 300 400 500 600 700 800 900 $ |
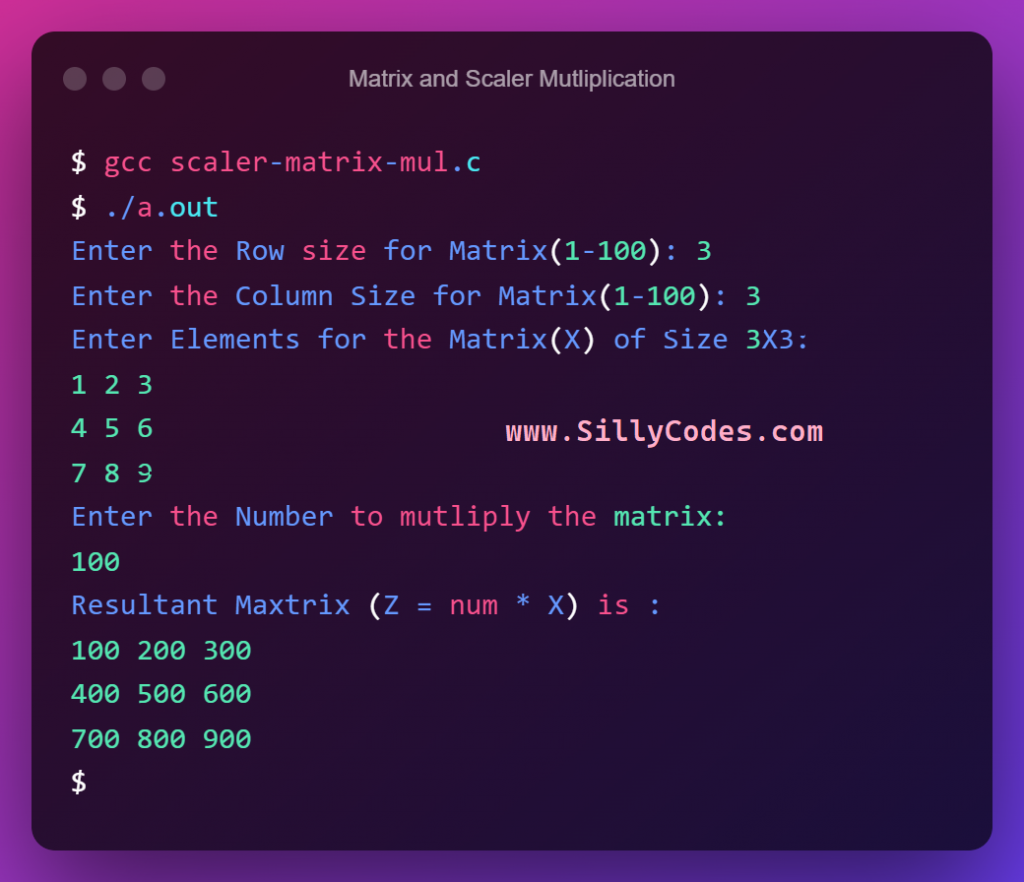
Let’s try another example.
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Enter the Row size for Matrix(1-100): 2 Enter the Column Size for Matrix(1-100): 3 Enter Elements for the Matrix(X) of Size 2X3: 5 10 15 20 25 30 Enter the Number to mutliply the matrix: 5 Resultant Maxtrix (Z = num * X) is : 25 50 75 100 125 150 $ |
As you can see from the above outputs, The program is generating the proper results.
Test 2: Check the error message.
Provide the invalid sizes ( i.e size > 100)
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 455 Enter the Column Size for Matrix(1-100): 9 Invalid Rows or Columns size, Please Try again $ ./a.out Enter the Row size for Matrix(1-100): 0 Enter the Column Size for Matrix(1-100): 234 Invalid Rows or Columns size, Please Try again $ |
As excepted, The Program is displaying the error message ( Invalid Rows or Columns size, Please Try again) whenever the size is out of the limits( 1-100).
Matrix Scalar Multiplication using Functions in C Language:
In the above program, We have used a single function( main()) to calculate the matrix scaler multiplication. So let’s divide the above program into the sub-functions.
Here is the modified version of the above Matrix Scaler Multiplication program with User-Defined functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 |
/* Program to multiply scaler and Matrix in C using functions SillyCodes.com */ #include <stdio.h> const int ROWS=100; const int COLUMNS=100; // function declaration 'scalerMul' void scalerMul(int rows, int columns, int num, int X[rows][columns], int Z[rows][columns]); /** * @brief - Read Matrix from the user and update 'X' * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'X' * @param X - 'X' is Matrix or 2D array */ void readMatrix(int rows, int columns, int X[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { //printf("X[%d][%d]: ", i, j); scanf("%d", &X[i][j]); } } } /** * @brief - Print the elements of the Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'X' * @param X[][] - 'X' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int X[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // Print Elements printf("%d ", X[i][j]); } printf("\n"); } } int main() { // Declare two matrices. // 'X' is Input matrice // 'Z' stores result of 'X' and scaler('num') multiplication int X[ROWS][COLUMNS], Z[ROWS][COLUMNS], num; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrix(1-%d): ", ROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrix(1-%d): ", COLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= ROWS || rows <= 0 || columns >= COLUMNS || columns <= 0) { printf("Invalid Rows or Columns size, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'X' printf("Enter Elements for the Matrix(X) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, X); printf("Enter the Number to mutliply the matrix: \n"); scanf("%d", &num); // Scaler Matrix multiplication num * X scalerMul(rows, columns, num, X, Z); // Print the Result printf("Resultant Maxtrix (Z = num * X) is : \n"); displayMatrix(rows, columns, Z); return 0; } /** * @brief - Multiply scaler('num') with Matrices pointed by 'X' and Store the result in 'Z' * * @param rows - Rows in the Matrices * @param columns - Columns in the Matrices * @param num - scaler Num * @param X - Matrix * @param Z - Resultant Matrix * @return void */ void scalerMul(int rows, int columns, int num, int X[rows][columns], int Z[rows][columns]) { int i, j; for(i=0; i<rows; i++) { for(j=0; j<columns; j++) { // muliply each elment with the 'num' Z[i][j] = num * X[i][j]; } } } |
We have defined three user-defined functions in the above program (except the main() function), The functions are
- The
readMatrix() function – Used to read the elements from the user and update the given matrix
- Prototype of readMatrix function – void readMatrix(int rows, int columns, int X[rows][columns])
- The readMatrix() function takes three formal arguments which are rows, columns, and Matrix X.
- This function uses two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &X[i][j]);
- As the arrays are passed by the address/reference, All the changes made to the Matrix-X in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
displayMatrix() function is used to print the Matrix elements on the Standard Output.
- The prototype of displayMatrix function is void displayMatrix(int rows, int columns, int X[rows][columns])
- Similar to the readMatrix() function, The displayMatrix() function also takes three formal arguments. i.e rows, columns, and X[][] (2D Array)
- The displayMatrix() function uses Two For loops to iterate over the X matrix and print the elements of the matrix. – printf("%d ", X[i][j]);
- The
scalerMul() function – This function multiples the scaler(
num) with Matrix pointed by
X and Store the result in Matrix
Z
- Prototype – void scalerMul(int rows, int columns, int num, int X[rows][columns], int Z[rows][columns])
- As you can see from the above prototype, This function takes Five Formal Arguments which are rows, columns, num, matrix X, and matrix Z.
- The scalerMul() function uses two for loops to iterate over the elements of the Matrices X and Mutliplies the scaler num with the all elements of the Matrix-X and stores the results in the Matrix-Z – Z[i][j] = num * X[i][j];
Program Output:
Let’s compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
$ gcc scaler-matrix-mul-func.c $ ./a.out Enter the Row size for Matrix(1-100): 4 Enter the Column Size for Matrix(1-100): 4 Enter Elements for the Matrix(X) of Size 4X4: 11 22 33 44 55 66 77 88 99 111 122 133 144 155 166 177 Enter the Number to mutliply the matrix: 2 Resultant Maxtrix (Z = num * X) is : 22 44 66 88 110 132 154 176 198 222 244 266 288 310 332 354 $ $ ./a.out Enter the Row size for Matrix(1-100): 2 Enter the Column Size for Matrix(1-100): 2 Enter Elements for the Matrix(X) of Size 2X2: 10 20 30 40 Enter the Number to mutliply the matrix: 1000 Resultant Maxtrix (Z = num * X) is : 10000 20000 30000 40000 $ |
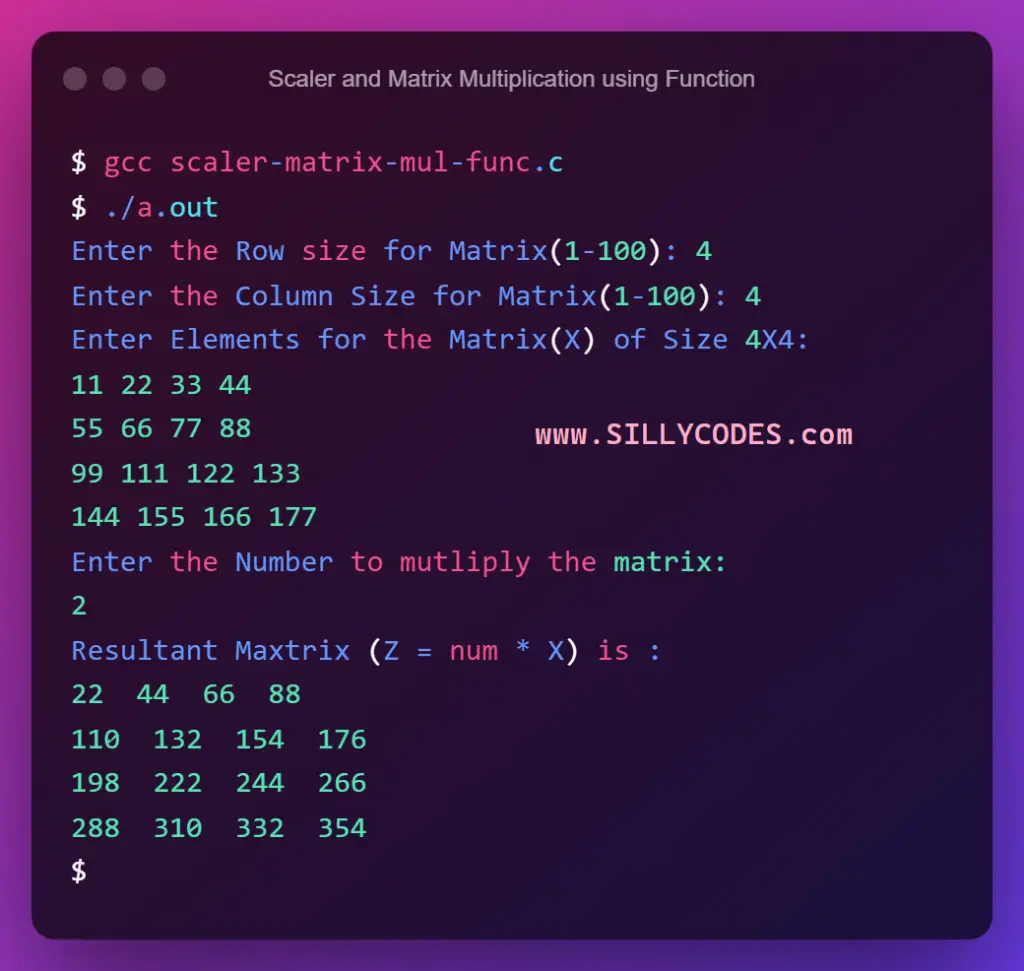
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter the Row size for Matrix(1-100): 3345 Enter the Column Size for Matrix(1-100): 0 Invalid Rows or Columns size, Please Try again $ ./a.out Enter the Row size for Matrix(1-100): 8 Enter the Column Size for Matrix(1-100): 0 Invalid Rows or Columns size, Please Try again $ |
The program is generating the excepted results.
Related Array Programs:
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
2 Responses
[…] Matrix and Scaler Multiplication Program in C […]
[…] Matrix and Scaler Multiplication Program in C […]