Remove all Occurrences of Character in String in C Language
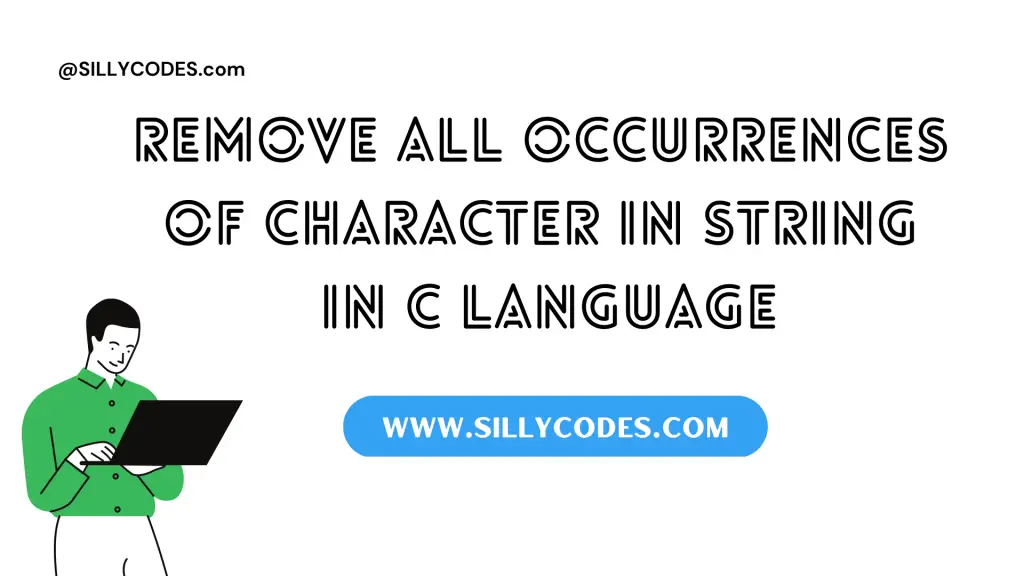
Program Description:
Write a Program to Remove all occurrences of character in string in C Programming Language. The program should accept a string and a character from the user and remove all occurrences of the said character from the string.
📢 This Program is part of the C String Practice Programs series
We will look at two methods to Remove all occurrences of a character in the string in C Language. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
Before going to look at the program, Let’s quickly check the excepted input and output of the program
Example Input and Output:
Input:
Enter a string : go go go go george
Enter character to remove from the string: g
Output:
String Before Removing All occurrences of character : go go go go george
String After Removing All Occurrences of character('g') : o o o o eore
The program removed all occurrences of the character g and returned the resultant string.
📢The program is case-sensitive. So the Uppercase characters and Lowercase characters are different.
Prerequisites:
It is recommended to know the basics of the string, function and how to pass string to functions. Please go through the following tutorials to learn more about these topics.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
We will look at the two different methods to Remove all occurrences of character in a string in C Language.
- Iterative Method to Remove all occurrence of a character from string
- User-defined Functions to remove characters
Remove All Occurrences of Character in String in C Program Explanation:
Here are the step-by-step explanation for the program.
- Start the program by creating a macro named SIZE. This macro holds the max size of the string- #define SIZE 100
- Declare the input string( inputStr) and character to search( ch).
- Take the input string and character to search from the user and update the inputStr and ch variables respectively. We use the gets function to read the string.
- Calculate the length of the input string inputStr and store it in length variable.
- Now, To Remove all occurrences of the character( ch) in a string( inputStr), Traverse over the input string, and find the position or index( i) of each occurrence of the character in the string. For each occurrence move all characters from the right side of the index i to one position left. ( i.e shift all characters to one place left)
- Create a Loop to find all occurrences of the character in the string. The For loop starts by initializing variable i to 0. It will continue looping as long as the present character (
inputStr[i]) is not a
NULLcharacter (
'\0').
- At each iteration, Check if the current character is equal( inputStr) to target character( ch). – i.e if(inputStr[i] == ch)
- If the above condition is
true, Then move all characters from the right side of the
iindex to one position left. To do this, Create a While loop and run it till the j < strlen(inputStr) condition is
true. (where
j = i;)
- At each iteration of the while loop, Copy the character at the index j+1 to index j- i.e inputStr[j] = inputStr[j+1];
- Increment the index j by 1. – j++;
- Otherwise, i.e if(inputStr[i] == ch) condition is false, Then continue to the next iteration to find the next occurrence of the character ch.
- Once the above Step 6 is completed, Then all occurrences of the character ch will be removed from the input string inputStr. Display the resultant string on the console.
- Stop the program.
Program to Remove All Occurrences of Character in String in C – Iterative Method:
Program to remove a characters from the string in the c programming language.
We need to include the string.h header file to use the strlen() library function. We can also implement custom string length function as well.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
/*     program to remove all occurrences of character from a string in C     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     char ch;     int i, j;      // Take the string from the user     printf("Enter a string : ");     gets(inputStr);     // Also take the search character     printf("Enter character to remove from the string: ");     scanf(" %c", &ch);      printf("String Before Removing All occurrences of character : %s \n", inputStr);      // calculate the string length.     int length = strlen(inputStr);      // Iterate over the string     for(i = 0; inputStr[i]; i++)     {         // check if the current character is equal to target('ch') character         if(inputStr[i] == ch)         {                         // Got the first character at index 'i'             // Move all characters from right side of 'i' to one position 'left'             j = i;             while (j < length)             {                 // copy 'j+1' to 'j' index                 inputStr[j] = inputStr[j+1];                 j++;             }              // reduce string 'length' and 'i' by 1             length--;             i--;         }     }      // Print the resultant string     printf("String After Removing All Occurrences of character('%c') : %s \n", ch, inputStr);      return 0; } |
Program Output:
Compile the program using your favorite compiler. We are using the GCC compiler on ubuntu linux OS.
Here are the instructions to Compile and Run the C Programs in Linux.
$ gcc remove-all.c
Run the program. The above command generates an exectuable file called a.out on linux OS and .exe file on windows OS. Run the executable file.
1 2 3 4 5 6 |
$ ./a.out Enter a string : go go go go george Enter character to remove from the string: g String Before Removing All occurrences of character : go go go go george String After Removing All Occurrences of character('g') : o o o o eore $ |
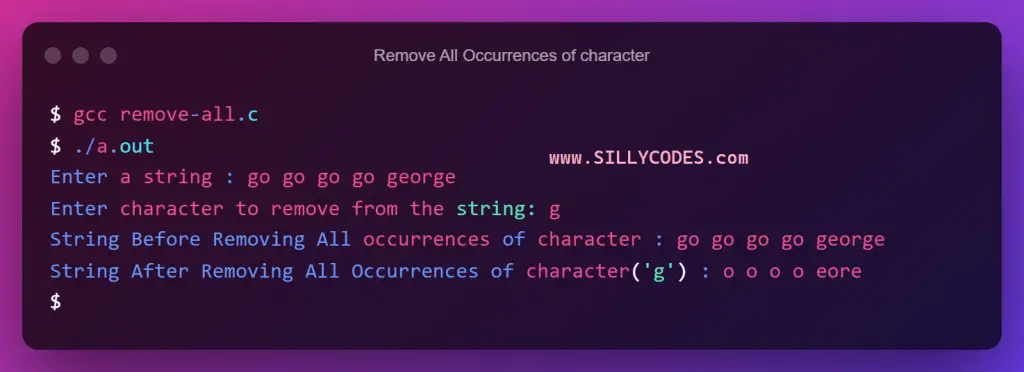
As we can see from the above output, The all occurrences of the character o is removed from the input string. Let’s look another example.
1 2 3 4 5 6 |
$ ./a.out Enter a string : AABBCCBBCCAABBAA Enter character to remove from the string: A String Before Removing All occurrences of character : AABBCCBBCCAABBAA String After Removing All Occurrences of character('A') : BBCCBBCCBB $ |
In this example, The input string is AABBCCBBCCAABBAA and the character to remove is A. So the program removed all occurrences of the character B in the input string and returned the resultant string as BBCCBBCCBB
Remove All Occurrences of Character in String using a user-defined function in C:
In the above program, We have written the complete program inside the mainfunction. It is not a recommended method, Let’s rewrite the above program to use a function to remove all occurrences of given character from the string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the Rewritten C program to remove all occurrences of the character from string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
/*     program to remove all occurrences of character from a string in C using functions     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string #define SIZE 100  /** * @brief  - Remove All occurences of 'ch' from 'inputStr' * * @param inputStr - Input String * @param ch      - Character to remove */ void removeAll(char * inputStr, char ch) {     int i, j;      // calculate the string length.     int length = strlen(inputStr);      // Iterate over the string     for(i = 0; inputStr[i]; i++)     {         // check if the current character is equal to target('ch') character         if(inputStr[i] == ch)         {                         // Got the first character at index 'i'             // Move all characters from right side of 'i' to one position 'left'             j = i;             while (j < length)             {                 // copy 'j+1' to 'j' index                 inputStr[j] = inputStr[j+1];                 j++;             }              // reduce string 'length' and 'i' by 1             length--;             i--;         }     }  }  int main() {      // declare a string with 'SIZE'     char inputStr[SIZE];     char ch;      // Take the string from the user     printf("Enter a string : ");     gets(inputStr);     // Also take the search character     printf("Enter character to remove from the string: ");     scanf(" %c", &ch);      printf("String Before Removing All occurrences of character : %s \n", inputStr);      // call the 'removeAll' with 'inputStr' and 'ch' to remove all occurrences     removeAll(inputStr, ch);      // Print the resultant string     printf("String After Removing All Occurrences of character('%c') : %s \n", ch, inputStr);      return 0; } |
We have defined a function called removeAll() to remove all occurrences of the character. Here is the prototype/syntax of the function
void removeAll(char * inputStr, char ch)
The removeAll() function takes two formal arguments, They are inputStr(character pointer) and ch(character). The inputStr points to the string and the ch variable holds the character to be removed.
This function go through the all elements of the inputStr string and removes or deletes all occurrences of the character ch from it.
Inside the main()function, Make a call to the removeAll() function with the inputStr and ch to remove all occurrences of the ch from inputStr
1 2 |
// call the 'removeAll' with 'inputStr' and 'ch' to remove all occurrences removeAll(inputStr, ch); |
Display the resultant string on the screen.
Program Output:
Let’s Compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc remove-all-func.c $ ./a.out Enter a string : We few, we happy few, we band of brothers. Enter character to remove from the string: w String Before Removing All occurrences of character : We few, we happy few, we band of brothers. String After Removing All Occurrences of character('w') : We fe, e happy fe, e band of brothers. $ $ ./a.out Enter a string : Roger Federer Enter character to remove from the string: e String Before Removing All occurrences of character : Roger Federer String After Removing All Occurrences of character('e') : Rogr Fdrr $ |
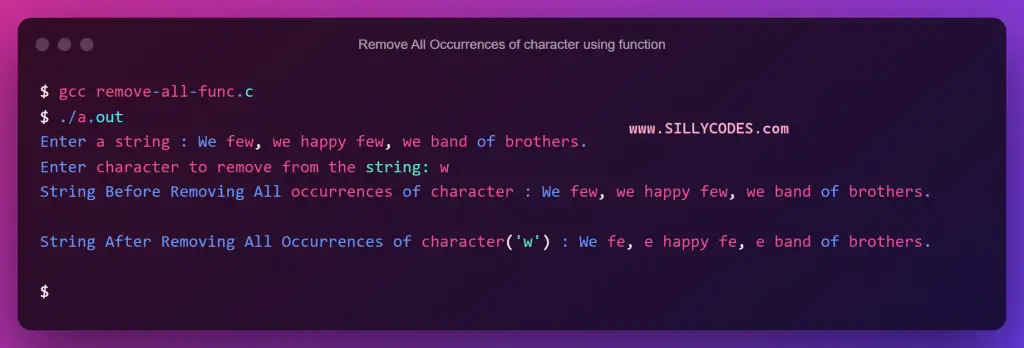
As we can see from the above outputs, The program is properly removing the all occurrences of the given character from the input string.
For example, All occurrences of the character e has been removed from the input string Roger Federer and the resultant string is Rogr Fdrr.
More String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Calculate Length of the string
- C Program to Copy a String to new string
- C Program to Concatenate Two strings
- C Program to Compare Two Strings
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String