Arithmetic Operations in C using functions
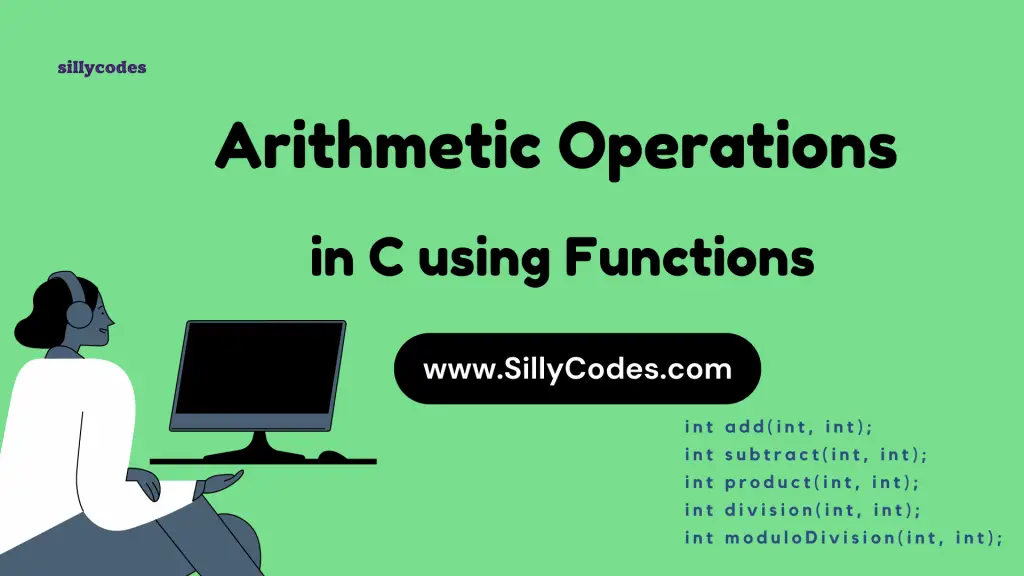
Program Description:
Write a Program to calculate all Arithmetic Operations in C using functions, The program should accept two integer numbers from the user and perform all arithmetic operations like addition, Subtraction, Multiplication, Division, and modulo division on given numbers.
We are going to create five functions, one for each arithmetic operation, The goal of the program is to familiarize ourselves with the functions. As we have written the arithmetic operations programs earlier without using functions.
Example Program Input and Output:
Input:
Enter two Integers: 50 3
Output:
Sum of 50+3 = 53
subtraction of 50-3 = 47
Multiplication of 50*3 = 150
Division of 50/3 = 16
ModuloDivision of 50%3 = 2
Pre-Requisites:
Arithmetic Operations in C using functions Program Explanation:
As specified earlier, We are going to create a function for each arithmetic operation. So we will have five functions in the program.
Here is the prototype of the functions.
int add(int, int);
int subtract(int, int);
int product(int, int);
int division(int, int);
int moduloDivision(int, int);
As you can see from the above prototypes, All functions take two integer numbers as input and return an integer number.
Algorithm:
- Take two numbers from the user and store them in variables number1 and number2.
- Call all functions one by one. Pass the
number1 and
number2 as the parameters and collect the return value.
- For example, We used add(number1, number2) to call the add function and passed the number1 and number2 parameters.
- The add function calculated the sum of the given number and returned the sum. ( return num1+num2;)
- Here, We are printing the result directly using the printffunction.
Arithmetic Operations in C using functions Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
/*     Program to perform arithmetic operations using functions */  #include<stdio.h> // Function declarations int add(int, int); int subtract(int, int); int product(int, int);  /*     function_name: division     arguments : (int, int)     return value: int     Description: Calculates division of 'num1' and 'num2' */ int division(int num1, int num2) {     return num1/num2; }  /*     Description: calculates moduloDivision of 'num1' and 'num2' */ int moduloDivision(int num1, int num2) {     return num1%num2; }  int main() {     // Take input from the user.     int number1, number2;     printf("Enter two Integers: ");     scanf("%d%d", &number1, &number2);      printf("Sum of %d+%d = %d \n", number1, number2, add(number1, number2));     printf("subtraction of %d-%d = %d \n", number1, number2, subtract(number1, number2));     printf("Multiplication of %d*%d = %d \n", number1, number2, product(number1, number2));     printf("Division of %d/%d = %d \n", number1, number2, division(number1, number2));     printf("ModuloDivision of %d%%%d = %d \n", number1, number2, moduloDivision(number1, number2));      return 0; }  /*     Description: calculates addition of 'num1' and 'num2' */ int add(int num1, int num2) {     return num1+num2; }  /*     Description: Calculates Subtractions of 'num1' and 'num2' */ int subtract(int num1, int num2) {     return num1-num2; }  /*     Description: calculates Product of 'num1' and 'num2' */ int product(int num1, int num2) {     return num1*num2; } |
Program Output – Arithmetic Operations in C using functions:
Compile and run the program using your favorite compiler, We are using the GCC compiler under Linux OS here.
1 2 3 4 5 6 7 8 9 |
$ gcc arithmetic-operations-function.c $ ./a.out Enter two Integers: 200 10 Sum of 200+10 = 210 subtraction of 200-10 = 190 Multiplication of 200*10 = 2000 Division of 200/10 = 20 ModuloDivision of 200%10 = 0 $ |
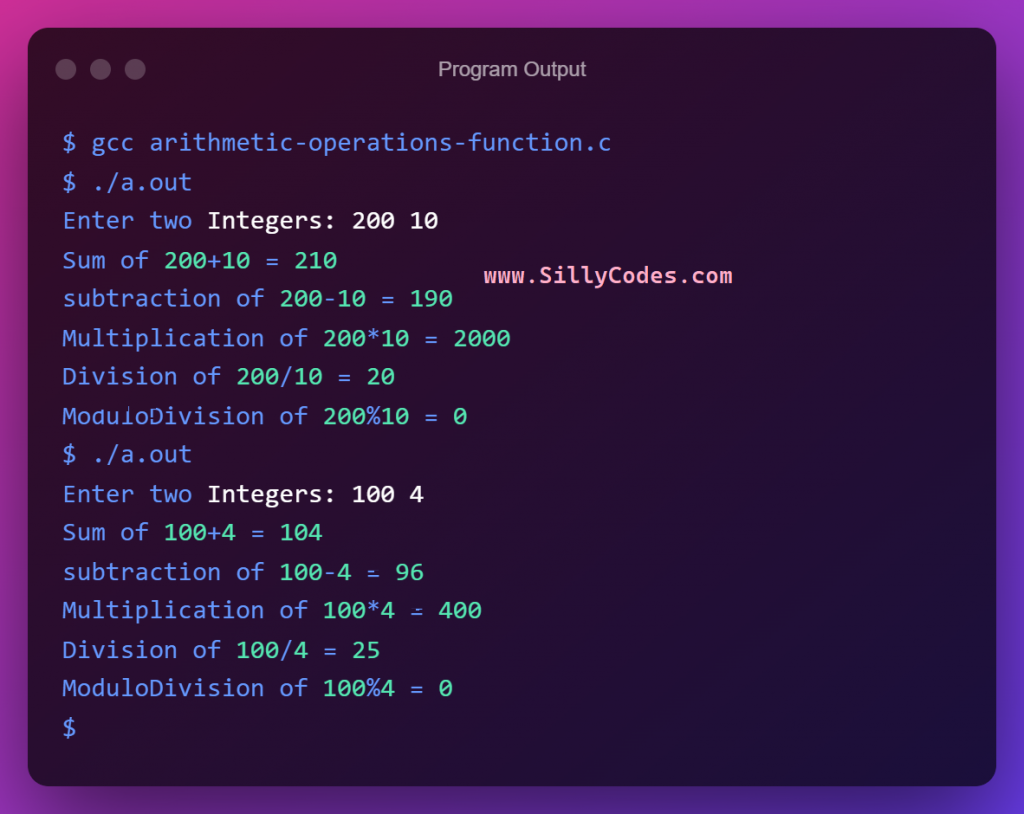
Let’s try a couple of tests
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ ./a.out Enter two Integers: 100 4 Sum of 100+4 = 104 subtraction of 100-4 = 96 Multiplication of 100*4 = 400 Division of 100/4 = 25 ModuloDivision of 100%4 = 0 $ ./a.out Enter two Integers: 50 3 Sum of 50+3 = 53 subtraction of 50-3 = 47 Multiplication of 50*3 = 150 Division of 50/3 = 16 ModuloDivision of 50%3 = 2 $ |
As we can see from the above output, We are getting the desired output.
Related Programs:
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of numberÂ
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
- C Program to Generate Nth Armstrong Number
- C Program to Generate Multiplication Tables
- Star and Number Pattern Programs [ Triangles, Pyramids, Rhombus, etc ]
5 Responses
[…] Arithmetic Operations using functions […]
[…] Arithmetic Operations using functions […]
[…] Arithmetic Operations using functions […]
[…] Arithmetic Operations using functions […]
[…] C Program to Perform Arithmetic Operations using functions […]