Program to Check Multiplicability of Two Matrices in C
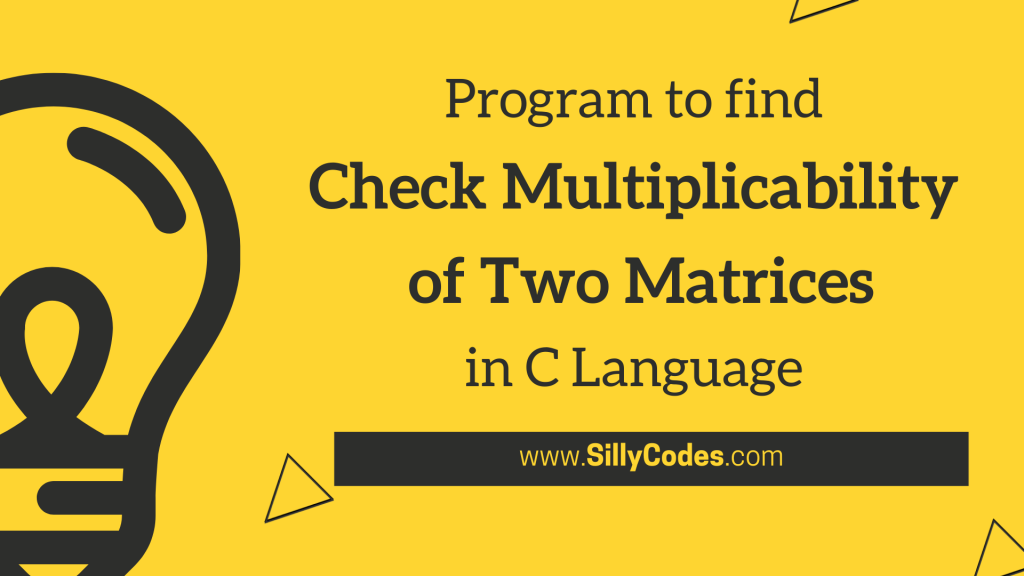
Program Description:
Write a Program to Check Multiplicability of Two Matrices in C programming language. The program should take the Two matrices’ row sizes and column sizes and check if the matrices can be multiplied.
Before going to look at the program to check Multiplicability of Two Matrices, Let’s understand what is meant by the Multiplicability of matrices.
What is meant by Multiplicability of Two Matrices?
Let’s say we have two matrices X and Y. The dimensions of the first matrix X is m x n. where m is equal to the number of rows in the X and n is equal to the number of columns in the X.
The dimensions of the second matrix Y is n x q.
The two matrices can be multiplicable only if the number of columns in the first Matrix(X) is equal to the number of rows in the second matrix(Y). This is called the Multiplicability of two matrices.
In the above example, The X matrix has dimensions as m x n and Y Matrix has dimensions as n x q. So the Matrices X and Y satisfy the condition and we can multiply the Matrix X and Matrix Y. The multiplication of the Matrix X and Matrix Y generates a new matrix called Matrix Z ( Z = X * Y). The matrix Z will have dimensions as m x q.
📢 Please look at the Matrix Multiplication Program Where we explained matrix multiplication in detail.
Example Input and Output:
Input:
1 2 3 4 |
Enter the Row size for First Matrix(1-100): 2 Enter the Column Size for First Matrix(1-100): 3 Enter the Row size for Second Matrix(1-100): 3 Enter the Column Size for Second Matrix(1-100): 2 |
Output:
1 2 |
SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(2X3) * Matrix-Y(3X2) Generates Matrix-Z(2X2) Size |
Program Prerequisites:
- Multi-Dimensional Arrays in C
- Read and Print 2D Arrays in C
- Passing 2D Arrays to Functions
- One dimensional Arrays in C
Check Multiplicability of Two Matrices in C Explanation/Algorithm:
- As we are not going to create any Matrices in the program, We no need to define any arrays.
- Declare four variables named rows1, columns1, rows2, and columns2.
- Prompt the user to provide the Row size and Column size of the First Matrix. And store the provided values in the rows1 and columns1 variables respectively.
- Similarly, Take the Second Matrix row size and column size from the user and update the rows2 and column2 variables.
- To check the Multiplicability of above two matrices, Compare the
columns1 of the first Matrix and
rows2 of the second matrix
- If the (columns1 != rows2) is true, which means, first matrix column and second matrix rows are not equal, So we can’t Multiply the matrices. Display the result on the console – ERROR: Can't Multiply Matrices, Please try again\n
- If the above condition is false, Then the First Matrix columns and second matrix rows are equal, So we can multiply the two matrices. Display the success message on the console – SUCCESS: We can Mutliply the Matrices X and Y
- Stop the program.
📢 This program is part of the Matrices Practice Programs
Program to Check Multiplicability of Two Matrices in C Language using Loops:
Here is the C Program to check if we can multiply two matrices.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/*     Program to Check Multiplicability of Two Matrices in C     SillyCodes.com */  #include <stdio.h>  int main() {     // As we are just checking the multiplicability we no need to declare arrays     int rows1, columns1, rows2, columns2;      // Take the Row size and Column size from user     // Row and Column size for first Matrix     printf("Enter the Row size for First Matrix: ");     scanf("%d", &rows1);     printf("Enter the Column Size for First Matrix: ");     scanf("%d", &columns1);      // Take row and column size for the second matrix     printf("Enter the Row size for Second Matrix: ");     scanf("%d", &rows2);     printf("Enter the Column Size for Second Matrix: ");     scanf("%d", &columns2);      // Check if we can mutliply the Matrices     if(columns1 != rows2)     {         printf("ERROR: Can't Multiply Matrices, Please try again\n");     }     else     {         printf("SUCCESS: We can Mutliply the Matrices X and Y \n");         printf("Matrix-X(%dX%d) * Matrix-Y(%dX%d) Generates Matrix-Z(%dX%d) Size \n",                 rows1, columns1, rows2, columns2, rows1, columns2);     }         return 0; } |
Program Output:
Compile the program using GCC (Any compiler)
$ gcc check-multiplicability.c
Run the program
Test 1: Positive Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ ./a.out Enter the Row size for First Matrix(1-100): 2 Enter the Column Size for First Matrix(1-100): 3 Enter the Row size for Second Matrix(1-100): 3 Enter the Column Size for Second Matrix(1-100): 2 SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(2X3) * Matrix-Y(3X2) Generates Matrix-Z(2X2) Size $ ./a.out Enter the Row size for First Matrix(1-100): 4Â Â Enter the Column Size for First Matrix(1-100): 6 Enter the Row size for Second Matrix(1-100): 6 Enter the Column Size for Second Matrix(1-100): 3 SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(4X6) * Matrix-Y(6X3) Generates Matrix-Z(4X3) Size $ |
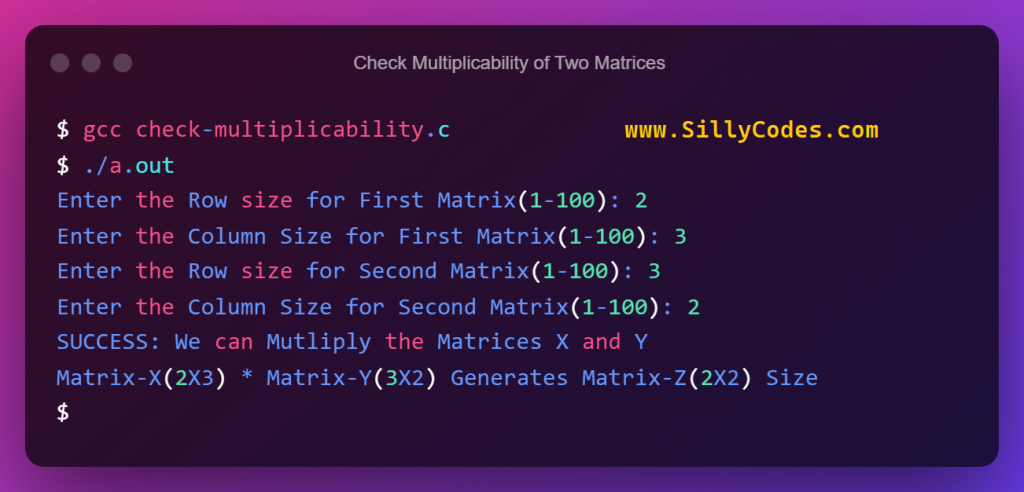
As we can see from the above output, In the first example, The First matrix column size ( 3) is equal to the second matrix row size( 3). So the program displayed we can multiply the matrices
1 2 |
SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(2X3) * Matrix-Y(3X2) Generates Matrix-Z(2X2) Size |
Test 2: Negative Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
//Negative $ ./a.out Enter the Row size for First Matrix(1-100): 3 Enter the Column Size for First Matrix(1-100): 5 Enter the Row size for Second Matrix(1-100): 3 Enter the Column Size for Second Matrix(1-100): 5 ERROR: Can't Multiply Matrices, Please try again $ ./a.out Enter the Row size for First Matrix(1-100): 4 Enter the Column Size for First Matrix(1-100): 2 Enter the Row size for Second Matrix(1-100): 6 Enter the Column Size for Second Matrix(1-100): 3 ERROR: Can't Multiply Matrices, Please try again $ |
In the above examples, The column size of the first matrix is not equal to the row size of the second matrix, So we can’t multiply these matrices.
Program to Check Multiplicability of Two Matrices using Functions in C:
Let’s rewrite the above program to use a function ( canMutliply) to check the Multiplicability of two matrices.
Here is the program with canMutliply function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/*     Program to Check Multiplicability of Two Matrices in C using Functions     SillyCodes.com */  #include <stdio.h> #include <stdbool.h>    // for 'bool'  /** * @brief  - Check the mutliplicability of two matrices * * @param rows1 - First Matrix(M1) rows * @param columns1 - M1 columns * @param rows2    - second matrix(M2) rows * @param columns2 - M2 Columns * @return true    - We can multiply M1 and M2 * @return false  - We can't Multiply M1 & M2 */ bool canMutliply(int rows1, int columns1, int rows2, int columns2) {     // Check if we can mutliply the Matrices     if(columns1 != rows2)     {         // Can't Multiply the Matrices         return false;     }      // Can Multiply     return true; }  int main() {     // As we are just checking the multiplicability we no need to declare arrays     int rows1, columns1, rows2, columns2;      // Take the Row size and Column size from user     // Row and Column size for first Matrix     printf("Enter the Row size for First Matrix: ");     scanf("%d", &rows1);     printf("Enter the Column Size for First Matrix: ");     scanf("%d", &columns1);      // Take row and column size for the second matrix     printf("Enter the Row size for Second Matrix: ");     scanf("%d", &rows2);     printf("Enter the Column Size for Second Matrix: ");     scanf("%d", &columns2);      // Call the 'canMutliply' function to check if the multiplicability of matrices     // We can even just pass 'column1' and 'row2' only - Change function definition also     if(canMutliply(rows1, columns1, rows2, columns2))     {         printf("SUCCESS: We can Mutliply the Matrices X and Y \n");         printf("Matrix-X(%dX%d) * Matrix-Y(%dX%d) Generates Matrix-Z(%dX%d) Size \n",                 rows1, columns1, rows2, columns2, rows1, columns2);     }     else     {         printf("ERROR: Can't Multiply Matrices, Please try again\n");     }         return 0; } |
📢As we are using the bool, We need to include the stdbool.h header file.
We have defined a user-defined function called canMultiply. The canMulitply() function will check the Multiplicability of two matrices and return the results.
- Prototype of the function – bool canMutliply(int rows1, int columns1, int rows2, int columns2)
- This function takes four Formal Arguments, They are rows1, columns1, rows2, and columns2
- canMultiply() function returns a Boolean Variable.
- If the matrices are multiplicable, Then canMultiply() function returns One(1), Otherwise, It returns Zero(0)
We called the canMultiply() function from the main() function and based on the return value, We displayed the result on the console.
Program Output:
Let’s compile and run the program and observe the results.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc check-multiplicability-func.c $ ./a.out Enter the Row size for First Matrix: 6 Enter the Column Size for First Matrix: 4 Enter the Row size for Second Matrix: 4 Enter the Column Size for Second Matrix: 6 SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(6X4) * Matrix-Y(4X6) Generates Matrix-Z(6X6) Size $ ./a.out Enter the Row size for First Matrix: 3 Enter the Column Size for First Matrix: 3 Enter the Row size for Second Matrix: 3 Enter the Column Size for Second Matrix: 3 SUCCESS: We can Mutliply the Matrices X and Y Matrix-X(3X3) * Matrix-Y(3X3) Generates Matrix-Z(3X3) Size $ ./a.out Enter the Row size for First Matrix: 7 Enter the Column Size for First Matrix: 5 Enter the Row size for Second Matrix: 7 Enter the Column Size for Second Matrix: 5 ERROR: Can't Multiply Matrices, Please try again $ |
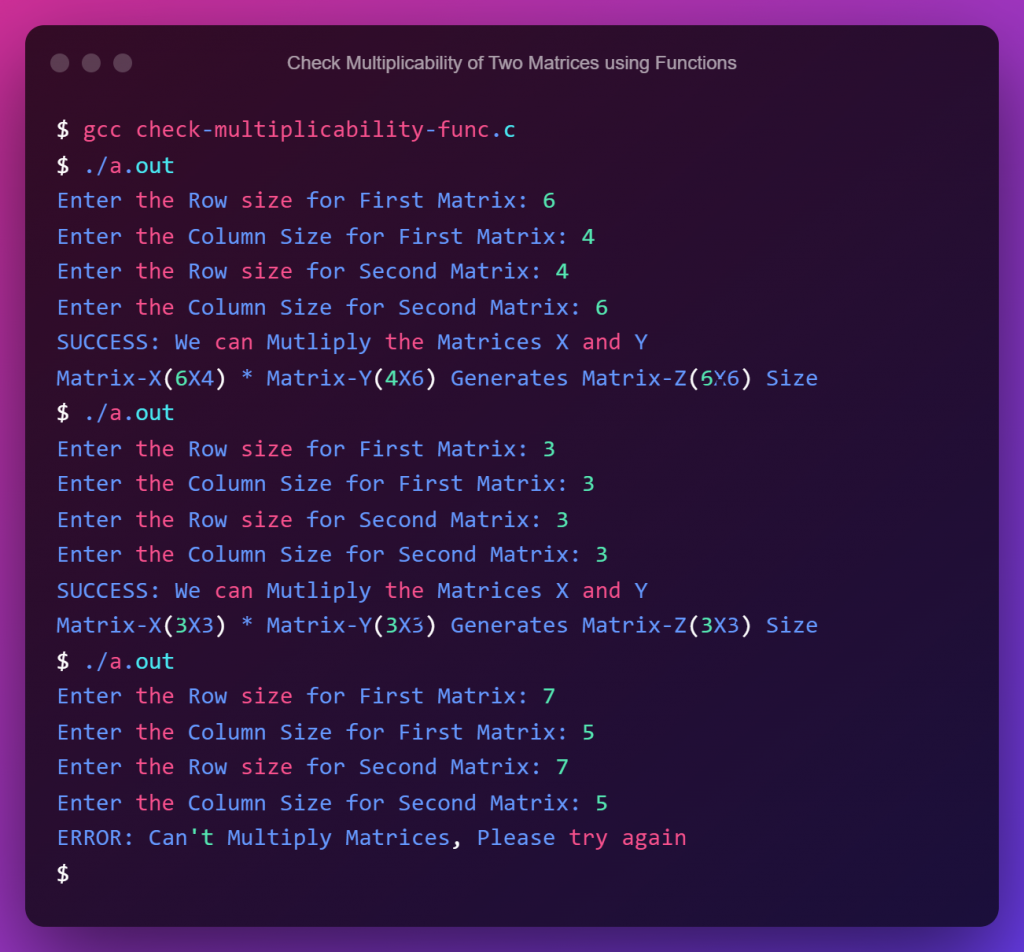
As we can see from the above output, The program is generating the desired results
Related Array Programs:
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
1 Response
[…] C Program to Check Multiplicability of Two Matrices […]