Program to Generate Nth Armstrong number in C Language
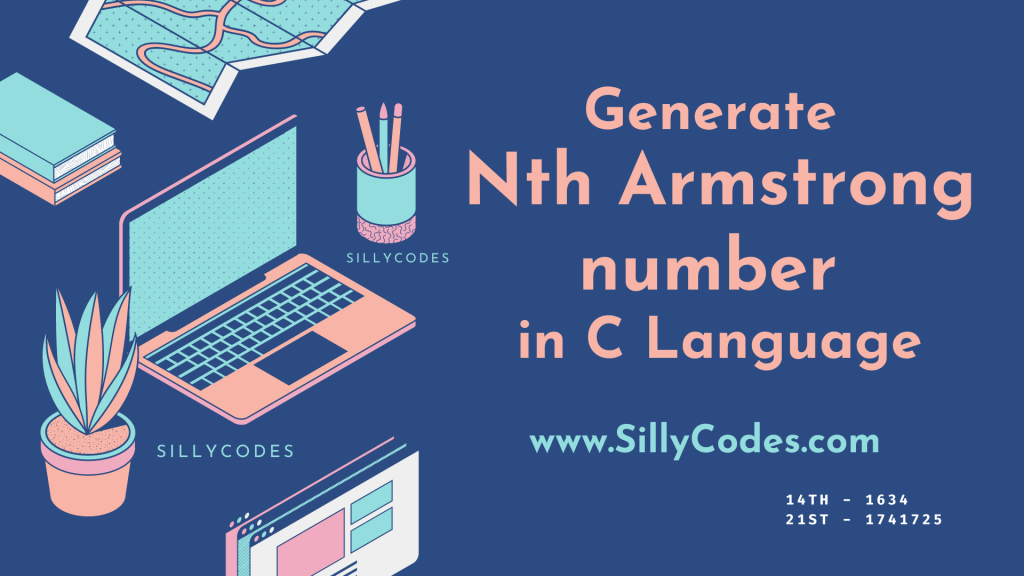
Nth Armstrong number in C Program Description:
Write a C Program to generate the Nth Armstrong number in C language. The program should accept a positive number ( n) from the user and display the Nth (user-provided) Armstrong Number using loops. The program should display an error message on Invalid inputs like negative numbers.
Here is a sample input and output of the program.
Example Input and Output:
Input:
Enter a Number(nth armstrong number): 16
Output:
16(th) Armstrong number is : 9474
What is an N-Digit Armstrong Number?
A positive number of n digits is said to be an Armstrong number of order n, If the sum of the power of n of each digit in the number is equal to the original number itself.
If the number is qwer is Armstrong number, Then it should satisfy the below equation.
abcd = pow(a, n) + pow(b, n) + pow(c, n) + pow(d, n)
Here n is the number of digits in the original number( abcd), which is 4 as we have 4 digits in abcd.
Here are a few Examples of Armstrong Numbers.
Digit | Armstrong Numbers |
---|---|
1 | 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 |
3 | 153, 370, 371, 407 |
4 | 1634, 8208, 9474 |
5 | 54748, 92727, 93084 |
6 | 548834 |
7 | 1741725, 4210818, 9800817, 9926315 |
Related Armstrong Numbers Programs:
This program is part of the Armstrong numbers programs series.
- Program Check 3-digit / N-digit Armstrong Numbers in C language
- Program to Generate Armstrong Numbers up to n (given number)
- Generate Armstrong numbers between two intervals in C
Pre-Requisites (Knowledge Required):
It is recommended to know the basics of the C Loops like the While Loop, For loop, And Arithmetic operators like Modulus operators, and Division Operators.
Nth Armstrong number in C Program Algorithm:
- Take a number from the user and store it in the variable n.
- Check if a given number n is a Negative number, If so display the error message and stop the program. Otherwise, proceed to the next step.
- We need to calculate the pow(digit, nDigits) to check the Armstrong number.
- So we need to count the number of digits in the given number n. To do that, We use a function called countDigits(), Which takes a number as input and returns the number of digits.- int nDigits = countDigits(temp);
- We are going to use two loops, Outer-Loop and Inner-Loop to get the Nth Armstrong number.
- The Outer-Loop: This starts from the number
1 and continues till we find our Nth Armstrong number, We use
cnt variable(default value is
1) to calculate the count of Armstrong numbers. We use the variable
i to control Outer-Loop. – for(i=1; cnt<=n; i++)
- Take the backup of the variable i and store it in variable temp. As we need i for later comparisons.
- The Inner-Loop: Inner-Loop will help us to check if the outer loop variable
i is an Armstrong number or not. If the
i is Armstrong number, Then increment the counter variable
cnt.
- At Each Iteration,
- Get the last digit of the number ( temp) i.e rem = temp %10;
- Calculate the power of rem with nDigits(Number of digits in i or temp) – i.e power(rem, nDigits);. We can use the inbuilt pow function or We can use a custom Power of Number Program in C to calculate the power of a number.
- Then Update the arm variable. – arm = arm + pow(rem, nDigits);
- Remove the last digit using the temp/10 operation.
- Check if the variable
arm is equal to
i. If both are equal, Then
i is an Armstrong number.
- Check our cnt is equal n (Nth Armstrong number), If the cnt is equal to n, We got our Nth Armstrong number. So print the result onto the console.
- Else increment the cnt variable.
- The above step-6 will continue until we find our Nth Armstrong number.
Program to generate Nth Armstrong number in C Language:
We are using the C language’s in-built pow function in this program.
Don’t forget to include the math.h headerfile to the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
/*     Program to generate Armstrong numbers up to the given number     Author: SillyCodes.com */  #include<stdio.h> #include<math.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  int main() {     int n, temp, rem, arm, i, cnt = 1;      // Take the input from the user.     printf("Enter a Number(nth armstrong number): ");     scanf("%d",&n);      // check if the 'n' is negative. Display the error message.     if(n <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      // Start the loop from '1' go till 'n'     for(i=1; cnt<=n; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // calculate the power of 'rem' with 'nDigits'             // We are using `math.h` in-built 'pow' function             arm = arm + pow(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)         {             if(cnt == n) {                 printf("%d(th) Armstrong number is : %d\n", n, i);                 break;             }             // else             cnt++;         }     }      return 0; } |
Program Output:
📢 As we are using the math.h headerfile, use the -lm compilation flag to include the libm.so library. If you forgot to include the -lm compilation option, You will get a Linking error.
Compile and run the program using the GCC compiler.
$ gcc nth-armstrong.c  -lm
Note, We passed -lm option to GCC. Run the program.
1 2 3 4 |
$ ./a.out Enter a Number(nth armstrong number): 10 10(th) Armstrong number is : 153 $ |
The first 9 Armstrong numbers are 1, 2, 3, 4, 5, 6, 7, 8, and 9. So the 10th Armstrong number is 153. Please check the above table, where we listed the Armstrong numbers up to 10000000.
Let’s try a few more examples.
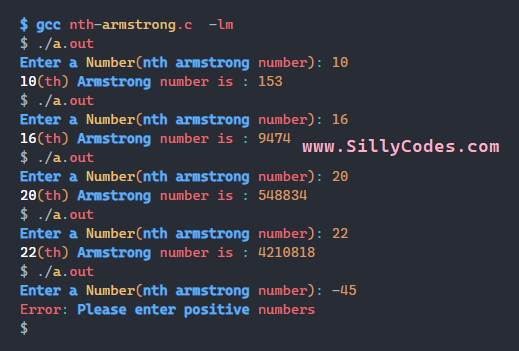
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Enter a Number(nth armstrong number): 16 16(th) Armstrong number is : 9474 Â $ ./a.out Enter a Number(nth armstrong number): 20 20(th) Armstrong number is : 548834 Â $ ./a.out Enter a Number(nth armstrong number): 22 22(th) Armstrong number is : 4210818 $ |
Negative numbers test case
1 2 3 4 |
$ ./a.out Enter a Number(nth armstrong number): -45 Error: Please enter positive numbers $ |
As expected, Program is displaying an error message, If the user enters a negative number.
Calculate Nth Armstrong number in C using the Custom Power function:
Let’s use our custom power function instead of the in-built power function. Here is the prototype of the custom power function.
double power(double base, double exponent);
As we are not going to use the math.h header file pow function, We can remove the math.h header file from our program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 |
/*     Program to generate Armstrong numbers up to the given number     Author: SillyCodes.com */  #include<stdio.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  /* * Calculate the power of number * Learn more at - https://sillycodes.com/program-to-find-power-of-number-in-c */ double power(double base, double exponent) {     long double res = 1.0;     // The power of base and exponent is equal to ( base multiplied exponent times)     // So loop until the exponent became zero.     while(exponent > 0) {         res = res * base;  // res *= base;         exponent--;     }     return res; }  int main() {     int n, temp, rem, arm, i, cnt = 1;      // Take the input from the user.     printf("Enter a Number(nth armstrong number): ");     scanf("%d",&n);      // check if the 'n' is negative. Display the error message.     if(n <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      // Start the loop from '1' go till 'n'     for(i=1; cnt<=n; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // calculate the power of 'rem' with 'nDigits'             // We are using custom power function.             arm = arm + power(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)         {             if(cnt == n) {                 printf("%d(th) Armstrong number is : %d\n", n, i);                 break;             }             // else             cnt++;         }     }      return 0; } |
Program Output:
Compile the program. We no need to include the -lm compilation flag ( as we are not using the math.h)
$ gcc nth-armstrong-custom.c
Run the program. ( ./a.out ). Let’s, try with the positive numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a Number(nth armstrong number): 14 14(th) Armstrong number is : 1634 $ ./a.out Enter a Number(nth armstrong number): 7 7(th) Armstrong number is : 7 $ ./a.out Enter a Number(nth armstrong number): 18 18(th) Armstrong number is : 92727 $ ./a.out Enter a Number(nth armstrong number): 21 21(th) Armstrong number is : 1741725 $ |
As you can see from the above output, We are getting the desired results.
Let’s try with the negative numbers.
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number(nth armstrong number): -9 Error: Please enter positive numbers $ ./a.out Enter a Number(nth armstrong number): -12 Error: Please enter positive numbers $ |
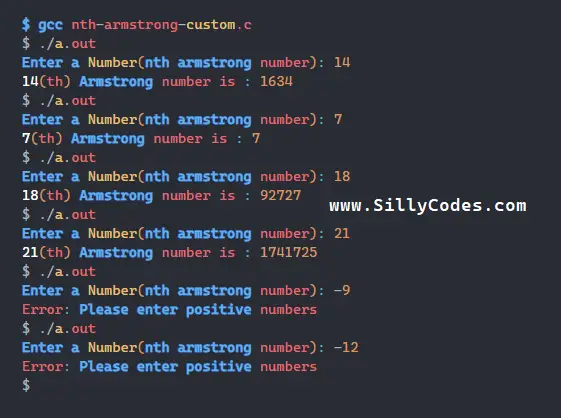
As expected, The program is displaying the error message ( Error: Please enter positive numbers ) when the user enters a Negative number.
Related Programs:
- C Loops Practice Programs Index
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of numberÂ
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
1 Response
[…] C Program to Generate Nth Armstrong Number […]