Count All Occurrences of Character in String in C Language
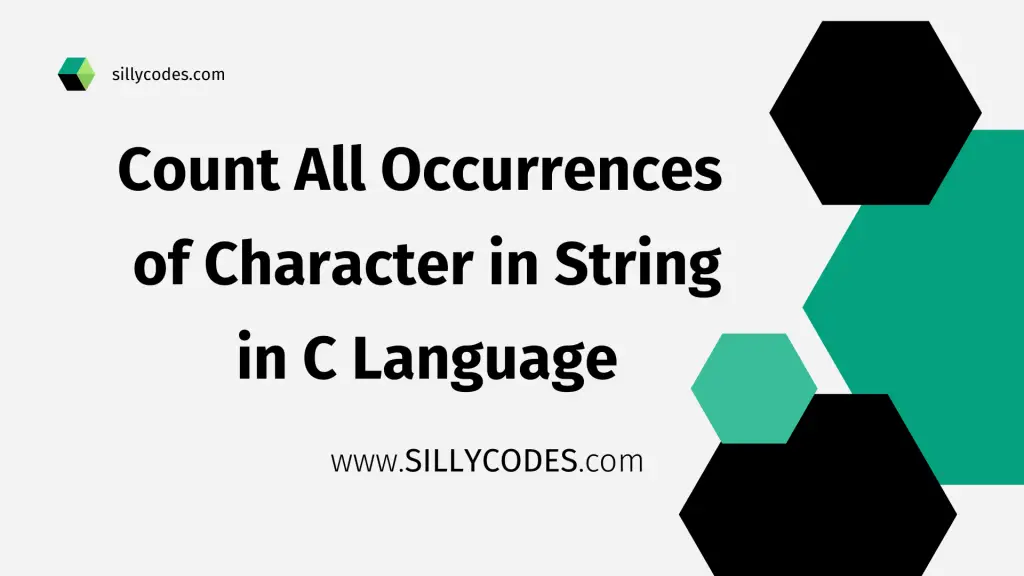
Program Description:
Write a Program to Count All Occurrences of Character in String in C programming language. The program will accept a string and a character from the user and calculates the number of times the character appeared in the given string.
📢 This Program is part of the C String Practice Programs series
Let’s look at the excepted input and output of the program.
Example Input and Output:
Input:
Enter a string : This is not the end, This is just the beginning.
Enter character to search in the string: i
Output:
FOUND: Character 'i' is found 6 times in given string
The character i is appeared six times in the input string.
📢 This program is case-sensitive. So the Uppercase characters and Lowercase characters are different.
We will look at a few methods to count all occurrences of a character in the string program. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
Prerequisites:
We are going to use the C-Strings and Functions, So it is recommended to know the basics of these concepts. please go through the following articles to refresh your knowledge.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
We will look at the three different methods to count all occurrences of a character in a string in C Language. They are,
- Iterative Method
- User-defined Functions
- Library Function ( strchr library function)
Method 1: Count All Occurrences of Character in String in C using Iterative Method:
Let’s look at the step-by-step explanation of the program.
Count All Occurrences of Character in String in C Program Explanation:
- Start the program by defining a global constant to hold the max size of the array – const int SIZE = 100;
- Declare the input string( inputStr) and character to be searched for ( ch)
- Take the input string and character to search from the user and update the inputStr and ch variables respectively. We use the gets function to read the string.
- Initialize a variable named countwith the Zero(0). The count variable is used to count all occurrences of the character ch in the inputStr string.
- To Count all occurrences of the character( ch) in a string( inputStr), Iterate over the string and compare each character with the target character( ch)
- Create a For loop by initializing variable i with 0. It will continue looping as long as the current character (
inputStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( inputStr[i]) is equal to ch- i.e if(inputStr[i] == ch)
- If the above condition is true, Then increment the count variable – i.e count++;
- Otherwise, Continue to the next iteration.
- Finally, Increment control variable i by 1.
- Once the above for loop is completed (step 6 is completed), Check if the variable
countis updated.
- If it is updated, Then we FOUND the ch in the inputStr and count variable contains the count of all occurrences of the character in the string. Print the results on the console.
- Otherwise, i.e if the count == 0, Then the character( ch) is not found in the string( inputStr). So display the NOT FOUND message on the console and terminated the program.
- Stop the program.
Program to count a character in string Iterative Method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/*     program to count all occurrences of a character in a string in c     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;         // Create counter variable and initialize with 'zero'     int i, count = 0;      // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // Iterate over the string     for(i = 0; str[i]; i++)     {         // check if the current character is equal to target('ch') character         if(str[i] == ch)         {             count++;         }     }      // check 'count'     if(count == 0)     {         // if 'count' is still 'zero', then 'ch' not found         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }     else     {         // Found         printf("FOUND: Character '%c' is found %d times in given string \n", ch, count);     }      return 0; } |
Program Output:
Let’s compile and run the program. We are using the GCC compiler.
Test Case 1:
1 2 3 4 5 6 |
$ gcc count-all-occ.c $ ./a.out Enter a string : This is not the end, This is just the beginning. Enter character to search in the string: i FOUND: Character 'i' is found 6 times in given string $ |
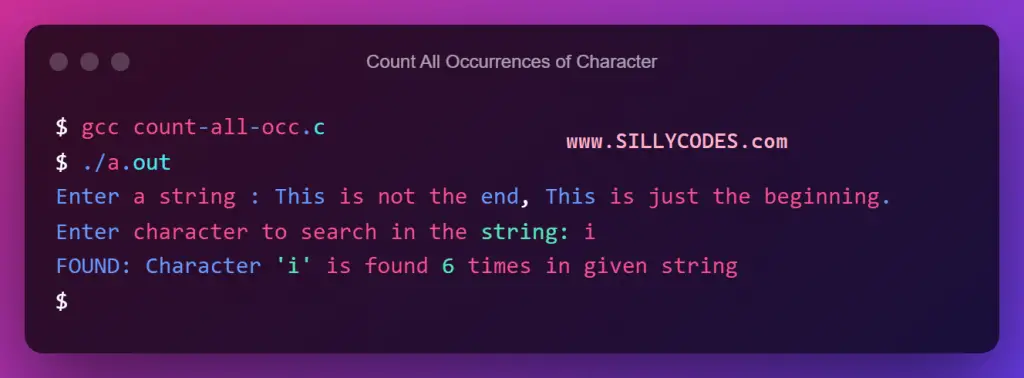
As we can see from the above output, The character i is found in the input string and it appeared six times in the input string.
Test Case 2:
1 2 3 4 5 |
$ ./a.out Enter a string : Git Graph Enter character to search in the string: e NOT FOUND: Character 'e' is not found in the given string $ |
In this example, The character e is not found in the input string Git Graph.
Method 2: Count All Occurrences of Character in String using user-defined functions in C:
In the above method, We have included the complete program inside the main() function. It is not a recommended method, Let’s rewrite the above program to use a function to count the number of occurrences of a character in a string in C.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the rewritten version of the program to count all occurrences of a character in a string using a user-defined function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
/*     program to count all occurrences of a character in a string in c using function     sillycodes.com */  #include<stdio.h>  // Max Size of string const int SIZE = 100;  /** * @brief  - Count All occurances of character and return result * * @param str - Input String * @param ch  - Character to search * @return int - Returns number of times chaacter present in the 'str' string. */ int countAllOccurrences(char *str, char ch) {     // Create counter variable and initialize with 'zero'     int i, count = 0;      // Iterate over the string     for(i = 0; str[i]; i++)     {         // check if the current character is equal to target('ch') character         if(str[i] == ch)         {             count++;         }     }      return count; }  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;         // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // call 'countAllOccurrences' function to count all occurances of 'ch' in 'str'     int cnt = countAllOccurrences(str, ch);      // check 'cnt'     if(cnt == 0)     {         // if 'cnt' is still 'zero', then 'ch' not found         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }     else     {         // Found         printf("FOUND: Character '%c' is found %d times in given string \n", ch, cnt);     }      return 0; } |
We have defined a user-defined function countAllOccurrences() to count all occurrences of character. Here is the syntax of the function
int countAllOccurrences(char *str, char ch)
This function accepts two formal arguments from the caller, They are the string( str) and the character to be searched( ch). And It counts the number of times the character ch is present in the input string str and returns the result back to the caller.
Call the countAllOccurrences() function from the main() function and pass the input string and the character.
1 2 |
// call 'countAllOccurrences' function to count all occurances of 'ch' in 'str' int cnt = countAllOccurrences(str, ch); |
We are also capturing the return value in the cnt variable and display the results based on the cnt variable.
1 2 3 4 5 6 7 8 9 10 11 |
// check 'cnt'     if(cnt == 0)     {         // if 'cnt' is still 'zero', then 'ch' not found         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }     else     {         // Found         printf("FOUND: Character '%c' is found %d times in given string \n", ch, cnt);     } |
Program Output:
Compile the program using your favorite compiler.
$ gcc count-all-occ-func.c
Run the Program.
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter a string : Microprocessors    Enter character to search in the string: s FOUND: Character 's' is found 3 times in given string $ ./a.out Enter a string : positive Enter character to search in the string: y NOT FOUND: Character 'y' is not found in the given string $ |
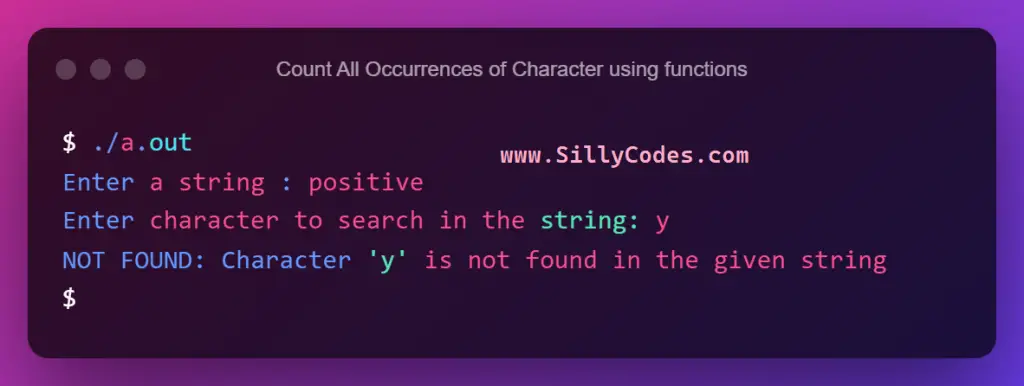
In the above examples, The character s is found 3 times in the Microprocessors string. Similarly, The character y is not found in the positive string.
Method 3: Count All Occurrences of Character in String in C using strchr function:
We can also use the strchr() library function to search for the first occurrence of a character in a string. Let’s use the strchr function to count all occurrences of a character in a string in c language.
📢 As we are using the strchr() library function, We need to include the string.h header file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/*     program to Count all occurrences of a character in a string in c using strchr     sillycodes.com */  #include <stdio.h> #include <string.h>  // Max Size of string const int SIZE = 100;  int main() {      // declare a string with 'SIZE'     char str[SIZE];     char ch;     char *ptr;      // Initialize 'counter' variable with zero     int counter = 0;      // Take the string from the user     printf("Enter a string : ");     gets(str);     // Also take the search character     printf("Enter character to search in the string: ");     scanf(" %c", &ch);      // Store the 'str' in 'tempPtr'     char * tempPtr = str;      // Use 'strchr' with 'tempPtr' to find all occurences of 'ch'     while((tempPtr = strchr(tempPtr, ch)) != NULL)     {         counter++;         tempPtr++;     }      // check 'counter'     if(counter == 0)     {         // if 'counter' is still 'zero', then 'ch' not found         printf("NOT FOUND: Character '%c' is not found in the given string\n", ch);     }     else     {         // Found         printf("FOUND: Character '%c' is found %d times in given string \n", ch, counter);     }      return 0; } |
As we have specified earlier, the strchr() function searches for the first occurrence of character ‘ch’ in the input string tempPtr i.e str. If the character ch is found, then the strchr() function returns a pointer to the first occurrence of ch in tempPtr. Otherwise,(i.e not found), then the function returns a NULL Pointer.
If the character is found, Then inside the while loop, increment the counter variable and the loop will continue to run and search for more occurrences of a character ch in string tempPtr, until the strchr() function returns a NULL pointer. This means we found all occurrences of the character ch in the input string( tempPtr) and updated the counter variable.
Finally, display the value of the counter variable(i.e all occurrences of character in c)
Program Output:
Let’s compile and run the program and observe the output.
1 2 3 4 5 6 |
$ gcc count-all-occ-strchr.c $ ./a.out Enter a string : Communication is key Enter character to search in the string: i FOUND: Character 'i' is found 3 times in given string $ |
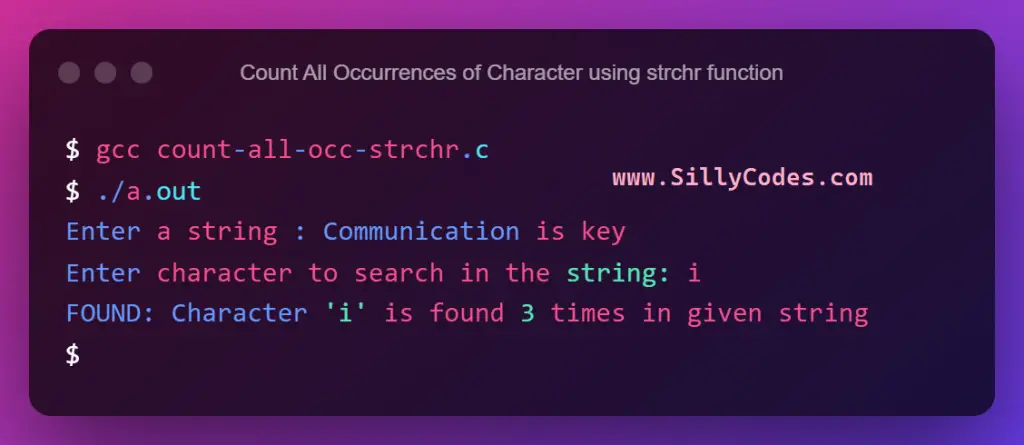
Related Articles:
- C Tutorials Index
- C Programs Index
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
1 Response
[…] C Program to Count All Occurrences of character in string […]