Left Rotation of Array in C Language – Left Rotate Array N Times
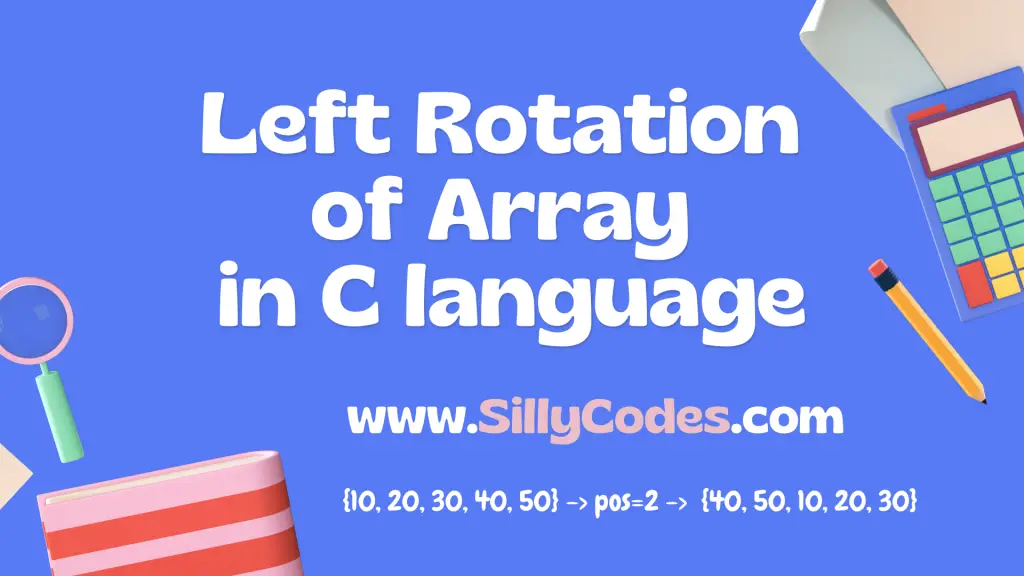
Program Description:
Write a Program to understand the Left Rotation of Array in C Programming Language. The program should accept an array from the user and the number of positions array need to be rotated in the left side direction. Then the program should Left Rotate the array in the specified number of positions and provide the results.
📢This Program is part of the Array Practice Programs Series
Here is the example input and output of the program.
Excepted Input and Output:
Input:
Enter desired size for array(1-100): 6
Please enter array elements(6) : 12 23 34 45 56 67
Enter the number of position to rotate the array: 2
Output:
Original Array: 12 23 34 45 56 67
Array After Left Rotating 2 Positions : 56 67 12 23 34 45
If we observe the above output, The Original Array is 12 23 34 45 56 67 and the user wants to Left rotate array by 2 positions in the left direction, So the resultant array is 56 67 12 23 34 45
Here is the graphical representation of the above-left rotation operation of Array.
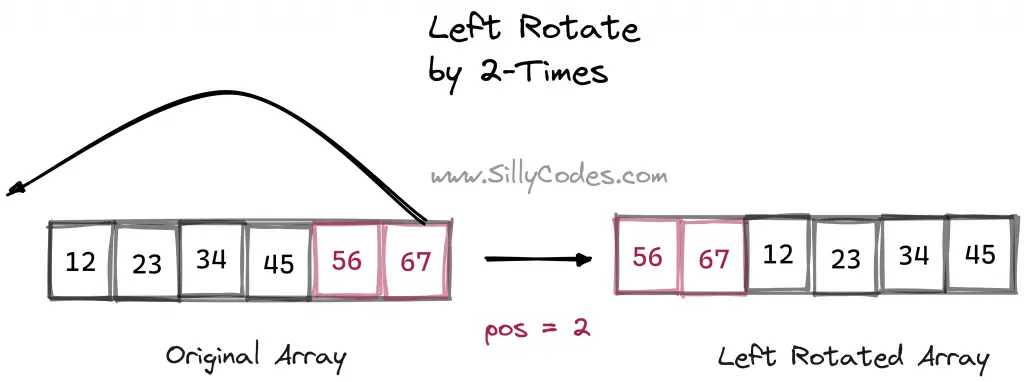
Prerequisites:
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
Let’s look at the algorithm to left rotate the array elements.
Algorithm for Left Rotation of Array in C Programming:
Let’s say the input array is numbers array and the total number of the array elements are equal to size. and The variable poscontains the number of elements that need to be rotated in the left direction.
- Array is numbers
- The size of the array is size
- The number of positions to be rotated is pos
To Rotate the array by pos elements,
- Create a Temporary Array( tempArr) with the same size of the input array numbers
- Now, We need to iterate over the input array ( numbers) and insert the elements in the temporary array at the correct rotated position.
- The correct position after rotating the array by
pos position can be calculated using the
(i+pos) % size.
- Where, i is the present element index ( for loop index)
- pos is the number of positions to be shifted in the left direction
- size is the total size of the input array.
- Once the above loop is completed, The temporary array ( tempArr) contains the Array which is rotated by pos positions.
- Replace the Input array ( numbers) elements with the Temporary array ( tempArr) elements.
- Now, The numbers array contains the Left Rotated array.
Left Rotation of Array in C Program Explanation:
Here is the step-by-step explanation of the Left Rotation of the Array program in the C Programming Language.
- Start the program by declaring two arrays. Input Array which is numbers and Temporary Array which is tempArr. The max size of both arrays is equal to 100. ( Change the 100 max size, if you want to use the large arrays).
- Take the desired array size from the user and store it in the size variable.
- Check if the size is going beyond the array bounds using – (size >= 100 || size <= 0) condition. Display an error message if the array is going out of bounds.
- We define two helper functions named
read() function and
display() function to read and print array elements
- The read() function is used to read the input elements from the user and update the numbers array
- The display() function is used to print the array elements on the Standard Output
- Now Call the read() function with numbers array and size as the parameter to Take user input and update the numbers array. – read(numbers, size);
- Make another function call to display() function to print the array elements on the console. – display(numbers, size);
- To Left Rotate the array of elements by positionnumber of elements,
- Once the above loop is completed, The tempArr contains the Left Rotated Array.
- Replace the original array elements with the
tempArr elements.
- Use another For loop start from i=0 to i<size
- Copy tempArr[i] to the numbers[i] – numbers[i] = tempArr[i];
- Display the Left rotated array on the console by calling the display() function from the main() function. – display(numbers, size);
- Stop the program.
Program to understand Left Rotation of Array in C Language:
Here is the example Program for Left Rotation of Array in C Programming Langauge.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
/*     Program Left Rotate Array 'n' positions     Author: SillyCodes.com */  #include <stdio.h>  /** * @brief  - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     // User input for array elements     printf("Please enter array elements(%d) : ", size);     for(i = 0; i < size; i++)     {         // printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief  - Display the 'numbers' array * * @param numbers - Array * @param size    - Array size */ void display(int numbers[], int size) {     int i;     for(i = 0; i < size; i++)     {         printf("%d ", numbers[i]);        }     printf("\n"); }   int main() {      // declare the 'numbers' array     int numbers[100], tempArr[100] = {0};     int i, position=0, size;      // Take the Array1('numbers') details from the user     printf("Enter desired size for array(1-100): ");     scanf("%d", &size);     // check for array bounds     if(size >= 100 || size <= 0)     {         printf("Invalid Size, Try again \n");         return 0;     }     // User input for array elements     read(numbers, size);      printf("Enter the number of position to rotate the array: ");     scanf("%d", &position);      // Print the 'numbers' array     printf("Original Array: ");     display(numbers, size);      // Store the rotated values of the original array in a temparory array     // store at ((i+position) % size) index in the new array     for(i = 0; i<size; i++)     {         // 'numbers[i]' goes to '(i+position) % size' index of 'tempArr'         tempArr[((i+position) %size)] = numbers[i];     }         // Replace the original array with elements with 'tempArr' elements     for ( i = 0; i < size; i++)     {         numbers[i] = tempArr[i];     }         // Print the merged Array     printf("Array After Left Rotating %d Positions : ", position);     display(numbers, size);      return 0; } |
Note that, we have defined two functions read() and display() in the above program.
Program Output:
Let’s Compile and Run the program and Observe the program output.
To compile the C Program use the following command.
$ gcc left-rotate-arr.c
The above command generated an executable file named a.out. Run the executable file.
Test Case 1: Positive test cases:
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 6 Please enter array elements(6) : 12 23 34 45 56 67 Enter the number of position to rotate the array: 2 Original Array: 12 23 34 45 56 67 Array After Left Rotating 2 Positions : 56 67 12 23 34 45 $ |
Let’s try free more examples and see the output
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 10 Please enter array elements(10) : 10 20 30 40 50 60 70 80 90 100 Enter the number of position to rotate the array: 5 Original Array: 10 20 30 40 50 60 70 80 90 100 Array After Left Rotating 5 Positions : 60 70 80 90 100 10 20 30 40 50 $ |
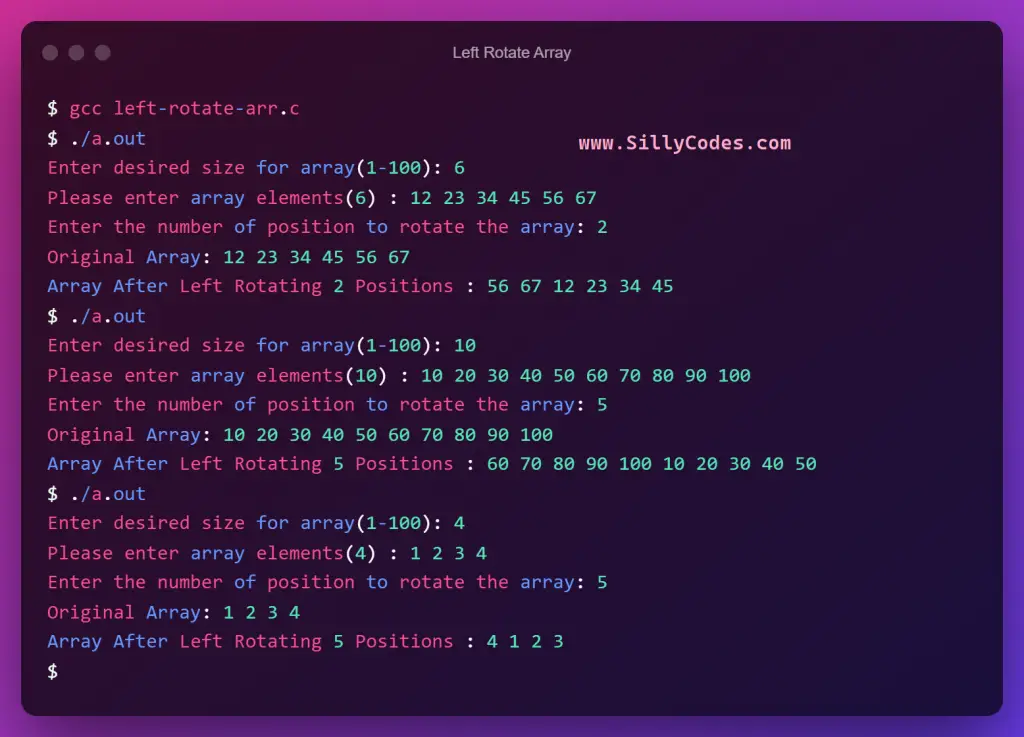
Test Case 2: Rotating more than the size of the array
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 4 Please enter array elements(4) : 1 2 3 4 Enter the number of position to rotate the array: 5 Original Array: 1 2 3 4 Array After Left Rotating 5 Positions : 4 1 2 3 $ |
If you observe the above array size is 4 but we Left Rotated by 5 elements, which is equal to 5-4 = 1. As After rotating 4 elements, We will end up with the original array, So technically we rotated only once.
Test Case 3: Negative tests – Array out of bounds.
1 2 3 4 5 6 7 |
$ ./a.out Enter desired size for array(1-100): 111 Invalid Size, Try again $ ./a.out Enter desired size for array(1-100): 0 Invalid Size, Try again $ |
As we can see from the above outputs, The program is generating the desired results. And displaying the error message if the array is going out of bounds.
Related Array Programs:
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
- C Program to Merge Two Arrays
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array